Python實現釘釘訂閱訊息功能
阿新 • • 發佈:2020-01-15
釘釘設定機器人
首先在釘釘設定釘釘機器人
群設定—> 智慧群助手—>新增機器人—>自定義
新增完成,得到一個Webhook API地址
Python指令碼實現推送釘釘訊息
釘釘官方給出了機器人介面的文件:https://ding-doc.dingtalk.com/doc#/serverapi2/qf2nxq
但是該文件只實現了JAVA和PHP的示例。以下是python指令碼的示例。
# -*- coding: utf-8 -*- """Created on Mon Jan 13 11:18:59 2020 python3 @author: 謝公子 """ import json import requests def send_msg(url,data): headers = {'Content-Type': 'application/json;charset=utf-8'} r = requests.post(url,data = json.dumps(data),headers=headers) return r.text if __name__ == '__main__': data = { "msgtype": "text","text": { "content": "hello,word!test" },} url = 'https://oapi.dingtalk.com/robot/send?access_token=xx' #此處為釘釘機器人的webhook地址 print(send_msg(url,data))
如果要實現簽名認證,如下
# -*- coding: utf-8 -*- """ Created on Mon Jan 13 11:18:59 2020 python3 @author: mi """ import json import requests import time import hmac import hashlib import base64 import urllib from urllib import parse def send_msg(url,headers=headers) return r.text def auth(secret): timestamp = round(time.time() * 1000) secret = secret #祕鑰 secret_enc = bytes(secret.encode('utf-8')) string_to_sign = '{}\n{}'.format(timestamp,secret) #把 timestamp+"\n"+金鑰 當做簽名字串 string_to_sign string_to_sign_enc = bytes(string_to_sign.encode('utf-8')) hmac_code = hmac.new(secret_enc,string_to_sign_enc,digestmod=hashlib.sha256).digest() #使用HmacSHA256演算法計算簽名,得到 hmac_code hmac_code_base64=base64.b64encode(hmac_code) #將hmac_code進行Base64 encode sign = urllib.parse.quote(hmac_code_base64) #進行urlEncode,得到最終的簽名sign authlist=[timestamp,sign] return authlist if __name__ == '__main__': data = { "msgtype": "link","link": { "text": "","title": "時代的火車向前開","messageUrl": "https://www.dingtalk.com/s?__biz=MzA4NjMwMTA2Ng" } } authlist = auth("你的簽名") url = "https://oapi.dingtalk.com/robot/send?access_token=xxx"+"×tamp="+str(authlist[0])+"&sign="+authlist[1] print(send_msg(url,data))
訊息型別
text型別 { "msgtype": "text","text": { "content": "我就是我,是不一樣的煙火@156xxxx8827" },"at": { "atMobiles": [ "156xxxx8827","189xxxx8325" ],"isAtAll": false } }
效果圖如下
link型別 { "msgtype": "link","link": { "text": "這個即將釋出的新版本,創始人xx稱它為“紅樹林”。 而在此之前,每當面臨重大升級,產品經理們都會取一個應景的代號,這一次,為什麼是“紅樹林”?","picUrl": "","messageUrl": "https://www.dingtalk.com/s?__biz=MzA4NjMwMTA2Ng } }
效果圖如下
markdown型別 { "msgtype": "markdown","markdown": { "title":"杭州天氣","text": "#### 杭州天氣 @156xxxx8827\n" + "> 9度,西北風1級,空氣良89,相對溫度73%\n\n" + "> 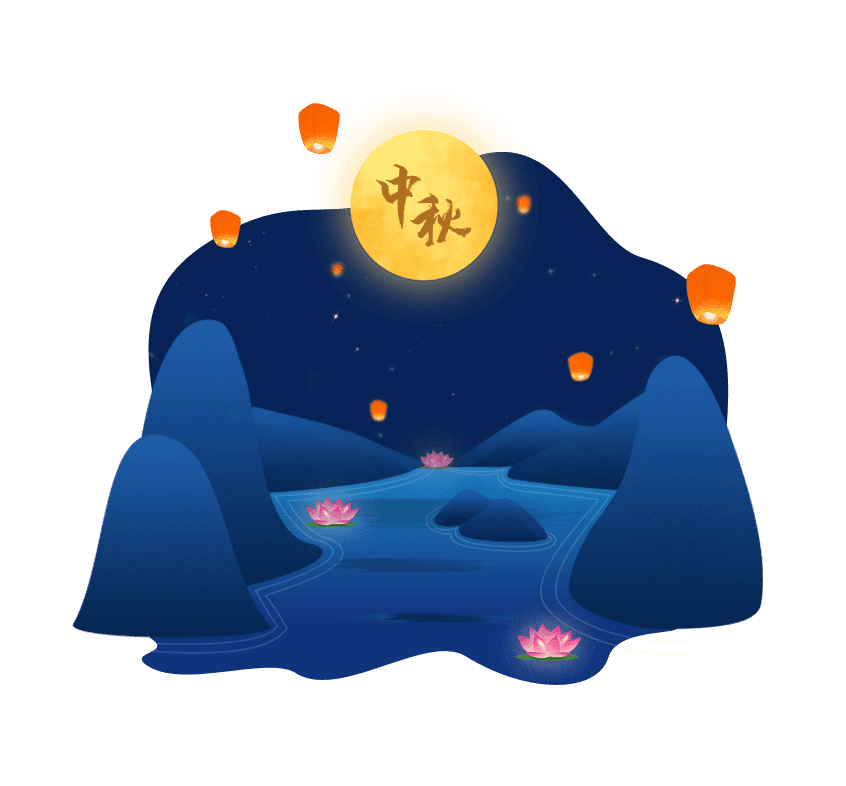\n" + "> ###### 10點20分發布 [天氣](http://www.thinkpage.cn/) \n" },"isAtAll": false } }
效果圖如下
整體跳轉ActionCard型別
{ "actionCard": { "title": "喬布斯 20 年前想打造一間蘋果咖啡廳,而它正是 Apple Store 的前身","text": " ### 喬布斯 20 年前想打造的蘋果咖啡廳 Apple Store 的設計正從原來滿滿的科技感走向生活化,而其生活化的走向其實可以追溯到 20 年前蘋果一個建立咖啡館的計劃","hideAvatar": "0","btnOrientation": "0","singleTitle" : "閱讀全文","singleURL" : "https://www.dingtalk.com/" },"msgtype": "actionCard" }
效果圖如下
獨立跳轉ActionCard型別
{ "actionCard": { "title": "喬布斯 20 年前想打造一間蘋果咖啡廳,而它正是 Apple Store 的前身","btns": [ { "title": "內容不錯","actionURL": "https://www.dingtalk.com/" },{ "title": "不感興趣","actionURL": "https://www.dingtalk.com/" } ] },"msgtype": "actionCard" }
效果圖如下
FeedCard型別 { "feedCard": { "links": [ { "title": "時代的火車向前開","messageURL": "https://www.dingtalk.com/s?__biz=MzA4NjMwMTA2Ng==&mid=2650316842&idx=1&sn=60da3ea2b29f1dcc43a7c8e4a7c97a16&scene=2&srcid=09189AnRJEdIiWVaKltFzNTw&from=timeline&isappinstalled=0&key=&ascene=2&uin=&devicetype=android-23&version=26031933&nettype=WIFI","picURL": "https://www.dingtalk.com/" },{ "title": "時代的火車向前開2","picURL": "https://www.dingtalk.com/" } ] },"msgtype": "feedCard" }
效果圖如下
總結
以上所述是小編給大家介紹的Python實現釘釘訂閱訊息功能,希望對大家有所幫助,如果大家有任何疑問請給我留言,小編會及時回覆大家的。在此也非常感謝大家對我們網站的支援!
如果你覺得本文對你有幫助,歡迎轉載,煩請註明出處,謝謝!