[C++]-string類的常用操作
阿新 • • 發佈:2020-07-30
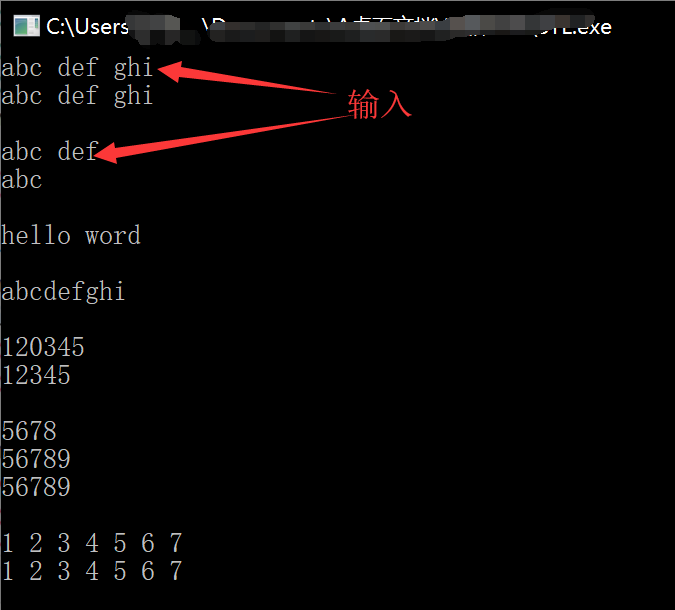#include<iostream> #include<string> #include<algorithm> using namespace std; int main() { /* ----獲取字串---- */ string s1; getline(cin, s1); // getline函式獲取一行字元,遇到回車結束 cout<<s1<<endl; cout<<endl; string s2; cin>>s2; // cin獲取一個單詞,遇到空格就會結束 cout<<s2<<endl; cout<<endl; /* ---- +=操作符---- */ string s3 = "hello"; s3 += " word"; // +=可以拼接字串 cout<<s3<<endl; cout<<endl; /* ----sort排序---- */ string s4 = "cbdeafghi"; sort(s4.begin(), s4.end()); // sort可以對string中的字元排序 cout<<s4<<endl; cout<<endl; /* ----插入刪除---- */ string s5 = "12345"; s5.insert(s5.begin()+2, '0'); // 在第2個索引位置插入字元0,原本該位置及之後的字元後移 cout<<s5<<endl; s5.erase(s5.begin()+2); // 刪除索引2位置的字元 cout<<s5<<endl; cout<<endl; /* ----字串切片---- */ string s6 = "0123456789", s; s = s6.substr(5, 4); // 從索引5開始(包括第5個)獲取連續的4個字元 cout<<s<<endl; s = s6.substr(5, -1); // 直接從第5個索引位置起擷取所有字元 cout<<s<<endl; s = s6.substr(5, 20); // 當第二個引數超出索引值,也只取到最後就結束 cout<<s<<endl; cout<<endl; /* ----遍歷方式---- */ string s7 = "1234567"; for(int i=0; i<s7.length(); i++) // 普通的for迴圈 cout<<s7[i]<<" "; cout<<endl; for(string::iterator it=s7.begin(); it!=s7.end(); ++it)// 使用迭代器 cout<<*it<<" "; cout<<endl; }