Android 本地tomcat伺服器接收處理手機上傳的資料之案例演示
阿新 • • 發佈:2018-11-15
上一篇:Android 本地tomcat伺服器接收處理手機上傳的資料之環境搭建
本篇基於上一篇搭建的伺服器端環境,具體介紹Android真機上傳資料到tomcat伺服器的互動過程
場景:Android客戶端上傳使用者名稱和密碼到tomcat服務端,tomcat伺服器自動接收Android客戶端上傳的資料,並打印出結果
一、tomcat伺服器端實現
1.首先啟動tomcat伺服器
直接點選“finish”即可,啟動後效果如下:
2. 編寫servlet實現:ServletDemo1.java
package com.servlet.demo;
import java.io.IOException;
import java.io.PrintWriter;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
/**
* Servlet implementation class ServletDemo1
*/
@WebServlet(asyncSupported = true, urlPatterns = { "/ServletDemo1" })
public class ServletDemo1 extends HttpServlet {
private static final long serialVersionUID = 1L;
private static Log Log = LogFactory.getLog(ServletDemo1.class);
/**
* The doGet method of the servlet. <br>
*
* This method is called when a form has its tag value method equals to get.
*
* @param request
* the request send by the client to the server
* @param response
* the response send by the server to the client
* @throws ServletException
* if an error occurred
* @throws IOException
* if an error occurred
*/
public void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
response.setContentType("text/html");
PrintWriter out = response.getWriter();
out.println("<!DOCTYPE HTML PUBLIC \"-//W3C//DTD HTML 4.01 Transitional//EN\">");
out.println("<HTML>");
out.println(" <HEAD><TITLE>A Servlet</TITLE></HEAD>");
out.println(" <BODY>");
out.print(" This is ");
out.print(this.getClass());
out.println(", using the GET method");
out.println(" </BODY>");
out.println("</HTML>");
out.flush();
out.close();
// 獲取請求的資料,並向控制檯輸出
String username = request.getParameter("username");
String password = request.getParameter("password");
System.out.println("-----> doGet username:" + username + " password:" + password);
}
/**
* The doPost method of the servlet. <br>
*
* This method is called when a form has its tag value method equals to
* post.
*
* @param request
* the request send by the client to the server
* @param response
* the response send by the server to the client
* @throws ServletException
* if an error occurred
* @throws IOException
* if an error occurred
*/
public void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
response.setContentType("text/html");
PrintWriter out = response.getWriter();
out.println("<!DOCTYPE HTML PUBLIC \"-//W3C//DTD HTML 4.01 Transitional//EN\">");
out.println("<HTML>");
out.println(" <HEAD><TITLE>A Servlet</TITLE></HEAD>");
out.println(" <BODY>");
out.print(" This is ");
out.print(this.getClass());
out.println(", using the POST method");
out.println(" </BODY>");
out.println("</HTML>");
out.flush();
out.close();
// 獲取請求的資料,並向控制檯輸出
String username = request.getParameter("username");
String password = request.getParameter("password");
System.out.println("-----> doPost username:" + username + " password:" + password);
}
}
3.執行Servlet
![]()
![]()
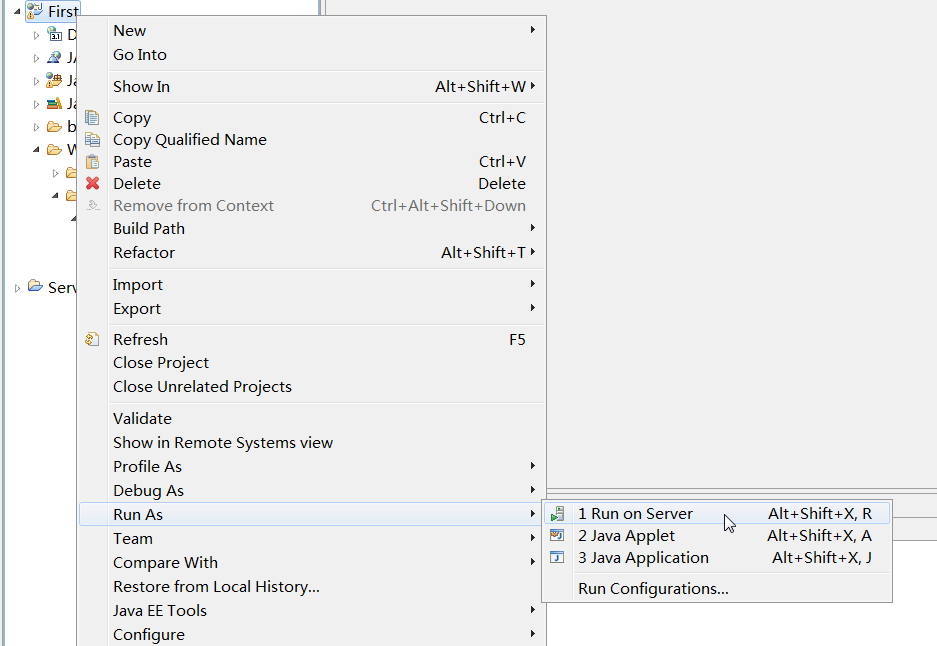
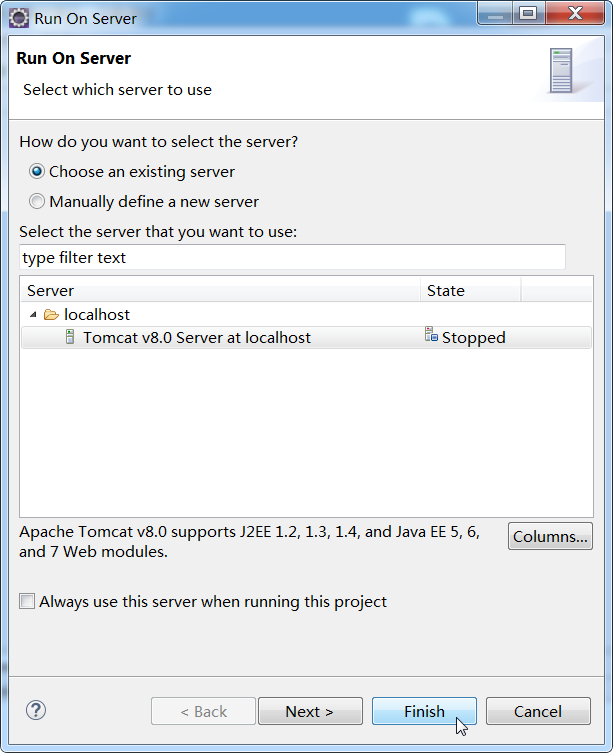
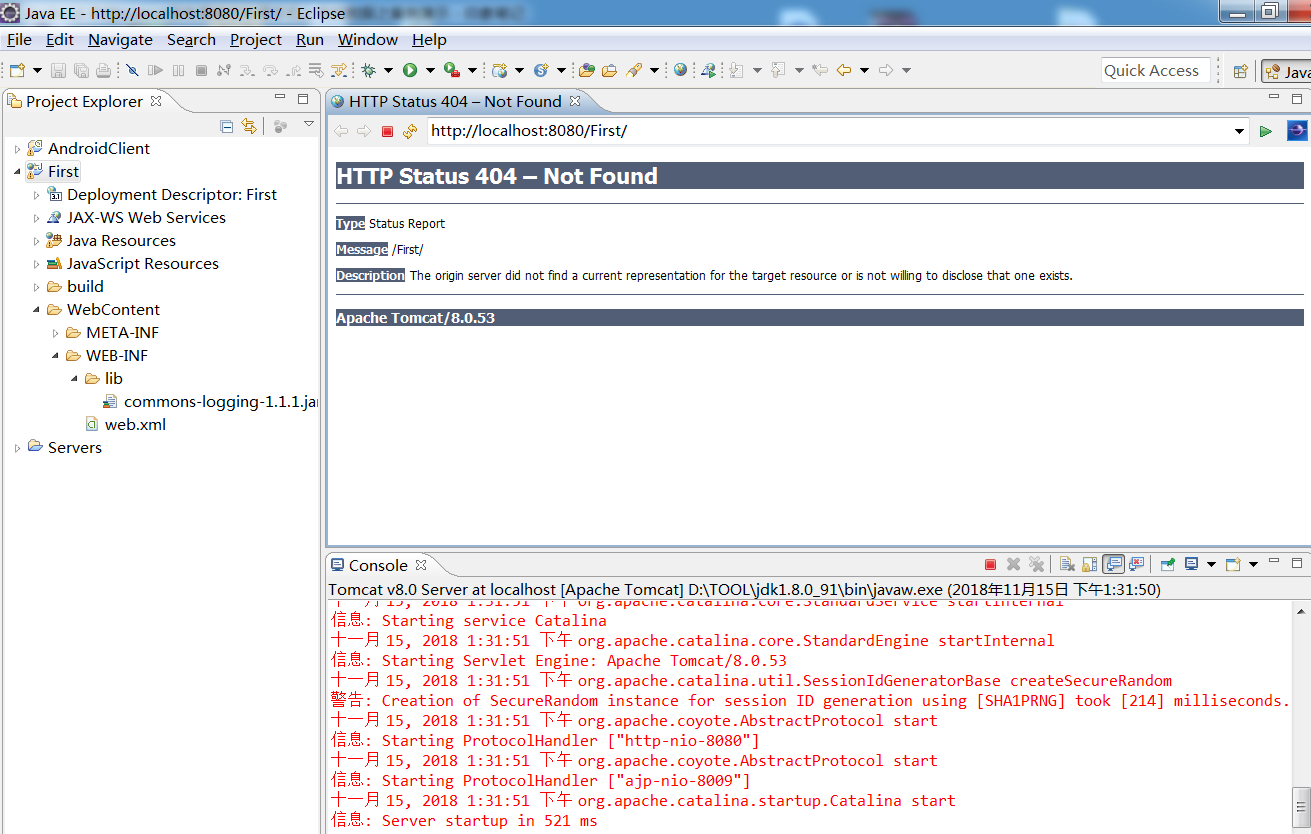
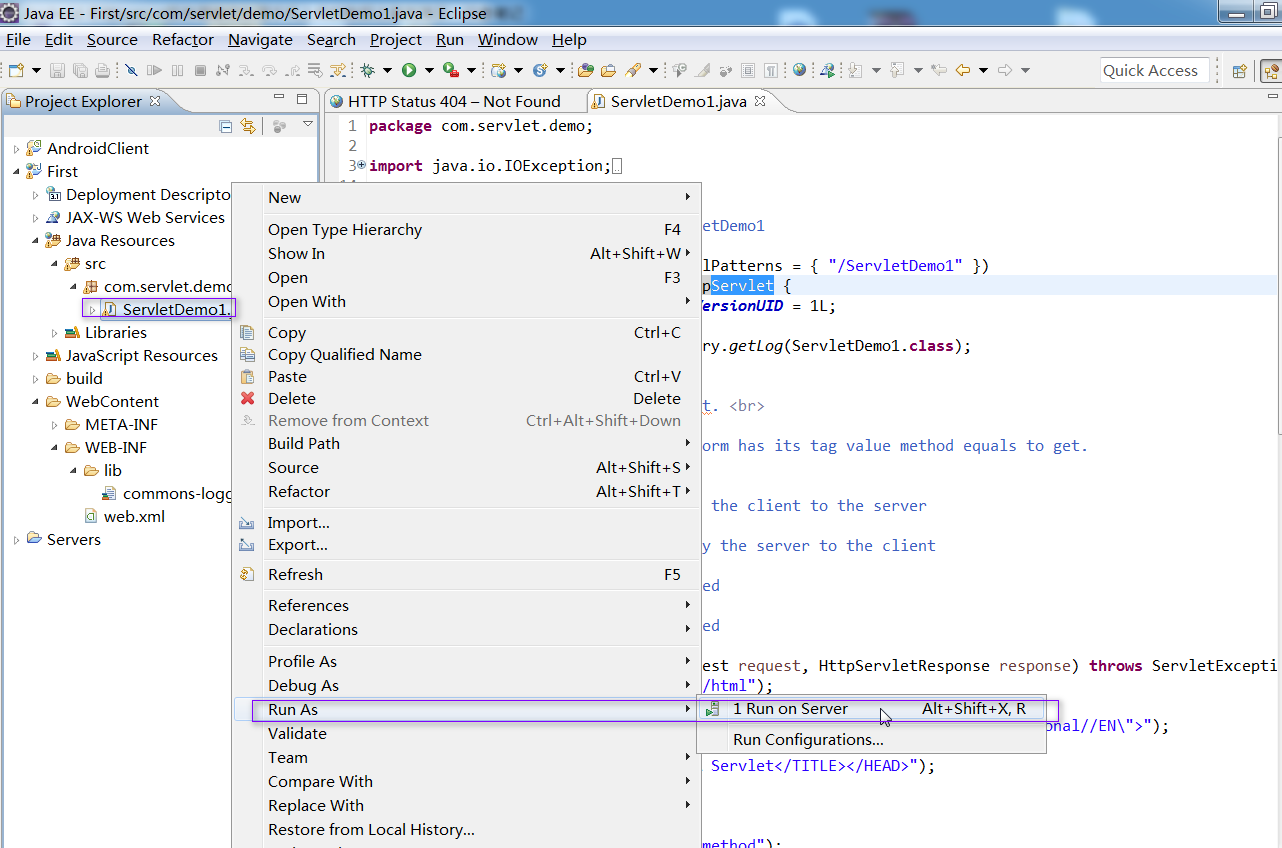
執行成功:
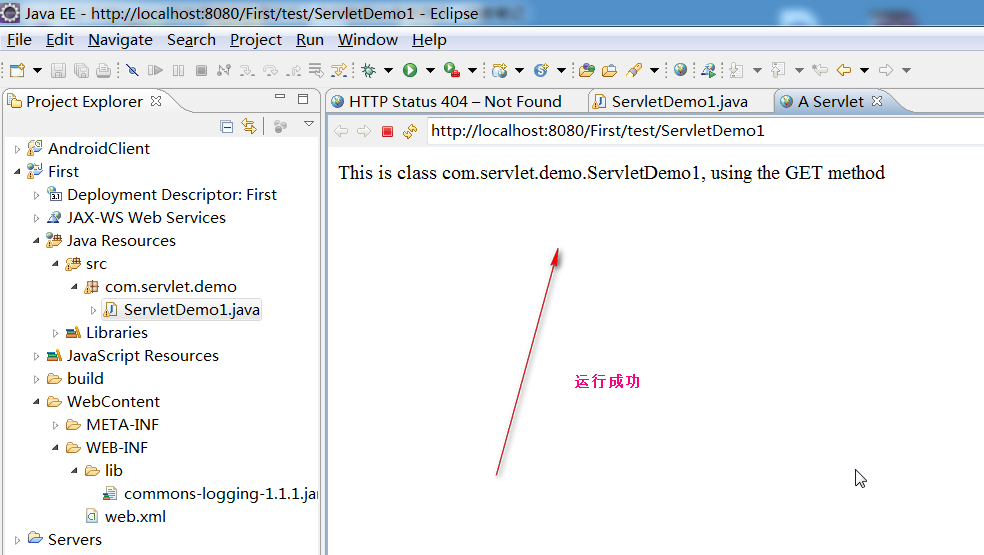
二、Android客戶端實現 1. 主頁 MainActivity package com.example.client; import android.app.Activity; import android.os.Bundle; import android.view.View; import android.widget.Button; import android.widget.EditText; public class MainActivity extends Activity { private EditText username; private EditText password; private Button signup; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); username = (EditText) findViewById(R.id.account); password = (EditText) findViewById(R.id.password); signup = (Button) findViewById(R.id.btnSign); signup.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { onLogin(); } }); } // 發起HTTP請求 public void onLogin() { String url = "http://192.168.191.1:8080/First/test/ServletDemo1"; new HttpTread(url, username.getText().toString(), password.getText().toString()).start(); } } 說明: 1. 上述 "http://192.168.191.1:8080/First/test/ServletDemo1",其中ip地址的確定方法,請檢視《Android 本地搭建Tomcat伺服器供真機測試》介紹 2. First:是web工程名 3. test/ServletDemo1:是如下高亮部分對映的名稱
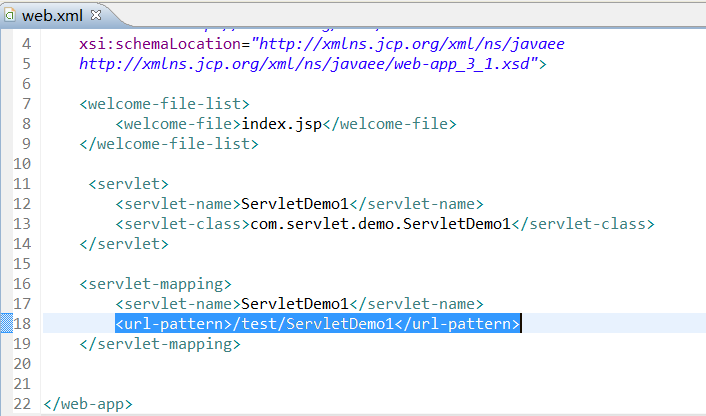
2. 執行緒HttpTread package com.example.client; import java.io.BufferedReader; import java.io.IOException; import java.io.InputStreamReader; import java.net.HttpURLConnection; import java.net.MalformedURLException; import java.net.URL; public class HttpTread extends Thread { String url; String username; String password; public HttpTread(String url, String username, String password) { this.url = url; this.username = username; this.password = password; } private void send() throws IOException { // 將username和password傳給Tomcat伺服器 url = url + "?username=" + username + "&password=" + password; try { URL httpUrl = new URL(url); // 獲取網路連線 HttpURLConnection conn = (HttpURLConnection) httpUrl.openConnection(); // 設定請求方法為GET方法 conn.setRequestMethod("GET"); // 或 "POST" // 設定訪問超時時間 conn.setReadTimeout(5000); BufferedReader reader = new BufferedReader(new InputStreamReader(conn.getInputStream())); String str; StringBuffer sb = new StringBuffer(); // 讀取伺服器返回的資訊 while ((str = reader.readLine()) != null) { sb.append(str); } // 把服務端返回的資料打印出來 System.out.println("HttpTreadResult:" + sb.toString()); } catch (MalformedURLException e) { } } @Override public void run() { super.run(); try { send(); } catch (IOException e) { e.printStackTrace(); } } } 3. AndroidManifest.xml <?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" package="com.example.client" android:versionCode="1" android:versionName="1.0" > <uses-sdk android:minSdkVersion="25" android:targetSdkVersion="25" /> <uses-permission android:name="android.permission.INTERNET" /> <application android:allowBackup="true" android:icon="@drawable/ic_launcher" android:label="@string/app_name" android:theme="@style/AppTheme" > <activity android:name=".MainActivity" android:label="@string/app_name" > <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> </application> </manifest> 說明: 需要宣告許可權:<uses-permission android:name="android.permission.INTERNET" /> 編碼結束。 4. Android 客戶端主頁面:
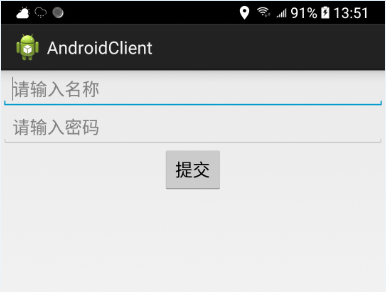
填寫使用者名稱和密碼,例如:使用者名稱:aaaa 密碼:bbb123
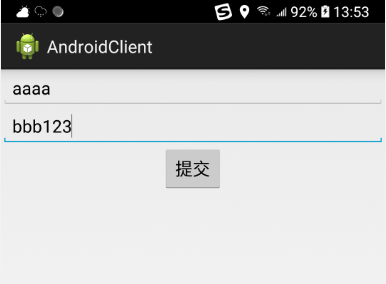
點選“提交”按鈕。切換到Eclipse中,可以看到Tomcat自動打印出所提交的資料:
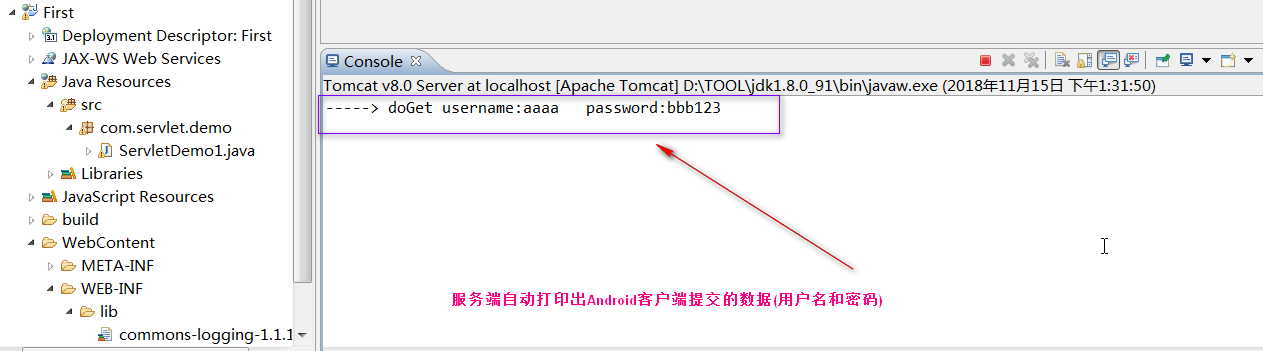
完成。 Android客戶端和tomcat服務端完整原始碼