iOS MVVM架構總結
阿新 • • 發佈:2018-12-14
為什麼使用MVVM
iOS中,我們使用的大部分都是MVC架構。雖然MVC的層次明確,但是由於功能日益的增加、程式碼的維護,使得更多的程式碼被寫在了Controller中,這樣Controller就顯得非常臃腫。
為了給Controller瘦身,後來又從MVC衍生出了一種新的架構模式MVVM架構。
MVVM分別指什麼
MVVM就是在MVC的基礎上分離出業務處理的邏輯到ViewModel層,即:
Model層:請求的原始資料
View層:檢視展示,由ViewController來控制
ViewModel層:負責業務處理和資料轉化
簡單來說,就是API請求完資料,解析成Model,之後在ViewModel中轉化成能夠直接被檢視層使用的資料,交付給前端(View層)。
MVVM與MVC的不同
首先我們簡化一下MVC的架構模式圖:
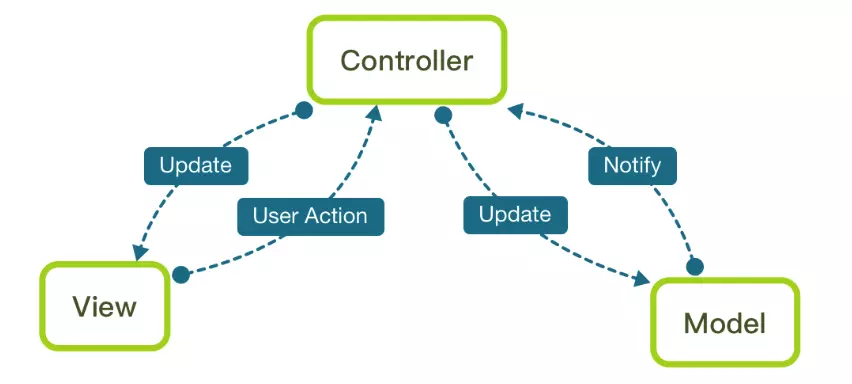
在這裡,Controller需要做太多得事情,表示邏輯、業務邏輯,所以程式碼量非常的大。而MVVM:
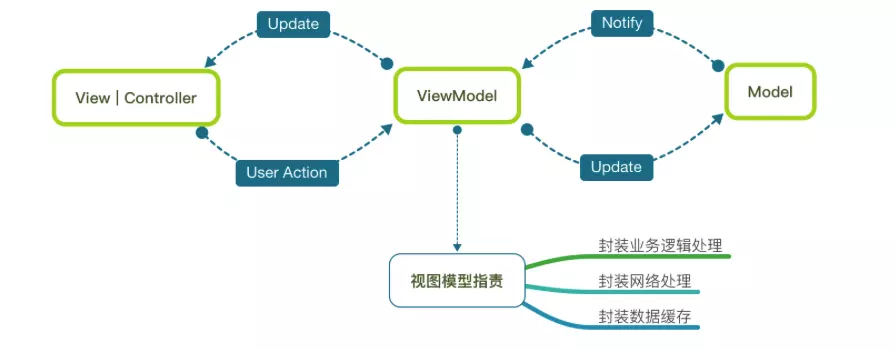
MVVM的實現
比如我們有一個需求:一個頁面,需要判斷使用者是否手動設定了使用者名稱。如果設定了,正常顯示使用者名稱;如果沒有設定,則顯示“部落格園0122”這種格式。(雖然這些本應是伺服器端判斷的)
我們看看MVC和MVVM兩種架構都是怎麼實現這個需求的
MVC:
Model類:
#import <Foundation/Foundation.h> @interface User : NSObject @property (nonatomic, copy) NSString *userName; @property (nonatomic, assign) NSInteger userId; - (instancetype)initWithUserName:(NSString *)userName userId:(NSInteger)userId; @end @implementation User- (instancetype)initWithUserName:(NSString *)userName userId:(NSInteger)userId { self = [super init]; if (!self) return nil; _userName = userName; _userId = userId; return self; } @end
ViewController類:
#import "HomeViewController.h" #import "User.h" @interface HomeViewController () @property (nonatomic, strong) UILabel *lb_userName; @property (nonatomic, strong) User *user; @end @implementation HomeViewController - (void)viewDidLoad { [super viewDidLoad]; //建立User例項並初始化 if (_user.userName.length > 0) { _lb_userName.text = _user.userName; } else { _lb_userName.text = [NSString stringWithFormat:@"部落格園%ld", _user.userId]; } } @end
這裡我們需要將表示邏輯也放在ViewController中。
MVVM:
Model類:
#import <Foundation/Foundation.h> @interface User : NSObject @property (nonatomic, copy) NSString *userName; @property (nonatomic, assign) NSInteger userId; @end
ViewModel類:
宣告:
#import <Foundation/Foundation.h> #import "User.h" @interface UserViewModel : NSObject @property (nonatomic, strong) User *user; @property (nonatomic, copy) NSString *userName; - (instancetype)initWithUserName:(NSString *)userName userId:(NSInteger)userId; @end
實現:
#import "UserViewModel.h" @implementation UserViewModel - (instancetype)initWithUserName:(NSString *)userName userId:(NSInteger)userId { self = [super init]; if (!self) return nil; _user = [[User alloc] initWithUserName:userName userId:userId]; if (_user.userName.length > 0) { _userName = _user.userName; } else { _userName = [NSString stringWithFormat:@"部落格園%ld", _user.userId]; } return self; } @end
Controller類:
#import "HomeViewController.h" #import "UserViewModel.h" @interface HomeViewController () @property (nonatomic, strong) UILabel *lb_userName; @property (nonatomic, strong) UserViewModel *userViewModel; @end @implementation HomeViewController - (void)viewDidLoad { [super viewDidLoad]; _userViewModel = [[UserViewModel alloc] initWithUserName:@"liu" userId:123456]; _lb_userName.text = _userViewModel.userName; } @end
可見,Controller中我們不需要再做多餘的判斷,那些表示邏輯我們已經移植到了ViewModel中,ViewController明顯輕量了很多。說白了,就是把原來ViewController層的業務邏輯和頁面邏輯等剝離出來放到ViewModel層。
總結:
- MVVM同MVC一樣,目的都是分離Model與View,但是它更好的將表示邏輯分離出來,減輕了Controller的負擔;
- ViewController中不要引入Model,引入了就難免會在Controller中對Model做處理;
- 對於很簡單的介面使用MVVM會增加程式碼量,但如果介面中內容很多、Cell樣式也很多的情況下使用MVVM可以很好地將VC中處理Cell相關的工作分離出來。
寫到這裡,MVVM基本上就算結束了。重要的還是去實踐,在實踐中檢驗真理。
https://www.jianshu.com/p/f1d0f7f01130