The definitive Node.js handbook
Note: you can get a PDF, ePub, or Mobi version of this handbook for easier reference, or for reading on your Kindle or tablet.
Table of Contents
Introduction to Node.js
This handbook is a getting started guide to Node.js, the server-side JavaScript runtime environment.
Overview
Node.js is a runtime environment for JavaScript
Node.js is an open source cross-platform. Since its introduction in 2009, it got hugely popular and now plays a significant role in the web development scene. If GitHub stars are one popularity indication factor, having 46,000+ stars means it is very popular.
Node.js is built on top of the Google Chrome V8 JavaScript engine, and it’s mainly used to create web servers — but it’s not limited to that.
It’s fast
One of the main selling points of Node.js is speed. JavaScript code running on Node.js (depending on the benchmark) can be twice as fast as compiled languages like C or Java, and orders of magnitude faster than interpreted languages like Python or Ruby, because of its non-blocking paradigm.
It’s simple
Node.js is simple. Extremely simple, actually.
It’s JavaScript
Node.js runs JavaScript code. This means that millions of front-end developers that already use JavaScript in the browser are able to run the server-side code and client-side code using the same programming language without the need to learn a completely different tool.
The paradigms are all the same, and in Node.js the new ECMAScript standards can be used first, as you don’t have to wait for all your users to update their browsers — you decide which ECMAScript version to use by changing the Node.js version.
It runs on V8
Running on the Google V8 JavaScript engine, which is open source — Node.js is able to leverage the work of thousands of engineers that made (and will continue to make) the Chrome JavaScript runtime blazing fast.
It’s an asynchronous platform
In traditional programming languages (C, Java, Python, PHP) all instructions are blocking by default unless you explicitly “opt in” to perform asynchronous operations. If you perform a network request to read some JSON, the execution of that particular thread is blocked until the response is ready.
JavaScript allows to create asynchronous and non-blocking code in a very simple way, by using a single thread, callback functions and event-driven programming. Every time an expensive operation occurs, we pass a callback function that will be called once we can continue with the processing. We’re not waiting for that to finish before going on with the rest of the program.
Such mechanism derives from the browser. We can’t wait until something loads from an AJAX request before being able to intercept click events on the page. It all must happen in real time to provide a good experience to the user.
If you’ve created an onclick handler for a web page you’ve already used asynchronous programming techniques with event listeners.
This allows Node.js to handle thousands of concurrent connections with a single server without introducing the burden of managing threads concurrency, which would be a major source of bugs.
Node provides non-blocking I/O primitives, and generally, libraries in Node.js are written using non-blocking paradigms, making a blocking behavior an exception rather than the normal.
When Node.js needs to perform an I/O operation, like reading from the network, access a database or the filesystem, instead of blocking the thread Node.js will simply resume the operations when the response comes back, instead of wasting CPU cycles waiting.
It has a huge number of libraries
With its simple structure, the node package manager(npm) helped the ecosystem of Node.js proliferate. Now the npm registry hosts almost 500,000 open source packages you can freely use.
A sample Node.js application
The most common example Hello World of Node.js is a web server:
const http = require('http')
const hostname = '127.0.0.1'const port = 3000
const server = http.createServer((req, res) => { res.statusCode = 200 res.setHeader('Content-Type', 'text/plain') res.end('Hello World\n')})
server.listen(port, hostname, () => { console.log(`Server running at http://${hostname}:${port}/`)})
To run this snippet, save it as a server.js
file and run node server.js
in your terminal.
This code first includes the Node.js http
module.
Node.js has an amazing standard library, including a first-class support for networking.
The createServer()
method of http
creates a new HTTP server and returns it.
The server is set to listen on the specified port and hostname. When the server is ready, the callback function is called, in this case informing us that the server is running.
Whenever a new request is received, the request
event is called, providing two objects: a request (an object) and a response (an object).
These 2 objects are essential to handle the HTTP call.
The first provides the request details. In this simple example, this is not used, but you could access the request headers and request data.
The second is used to return data to the caller.
In this case with:
res.statusCode = 200
We set the statusCode
property to 200
, to indicate a successful response.
We set the Content-Type header:
res.setHeader('Content-Type', 'text/plain')
…and we end close the response, adding the content as an argument to end()
:
res.end('Hello World\n')
Node.js frameworks and tools
Node.js is a low-level platform. To make things easier and more interesting for developers, thousands of libraries were built upon Node.js.
Many of those established over time as popular options. Here is a non-comprehensive list to the ones I consider very relevant and worth learning:
- Express One of the most simple yet powerful ways to create a web server. Its minimalist approach and unopinionated focus on the core features of a server is key to its success.
- MeteorAn incredibly powerful full-stack framework, empowering you with an isomorphic approach to building apps with JavaScript and sharing code on the client and the server. Once an off-the-shelf tool that provided everything, it now integrates with front-end libraries such as React, Vue and Angular. Meteor can be used to create mobile apps as well.
- KoaBuilt by the same team behind Express, Koa aims to be even simpler and smaller, building on top of years of knowledge. The new project was born out of the need to create incompatible changes without disrupting the existing community.
- Next.jsThis is a framework to render server-side rendered React applications.
- MicroThis is a very lightweight server to create asynchronous HTTP microservices.
- Socket.ioThis is a real-time communication engine to build network applications.
A brief history of Node.js
A look back on the history of Node.js from 2009 to today
Believe it or not, Node.js is just 9 years old.
In comparison, JavaScript is 23 years old and the web as we know it (after the introduction of Mosaic) is 25 years old.
9 years is such a little amount of time for a technology, but Node.js seems to have been around forever.
I’ve had the pleasure to work with Node.js since the early days when it was just 2 years old, and despite the little information available, you could already feel it was a huge thing.
In this section, I want to draw the big picture of Node.js in its history, to put things in perspective.
A little bit of history
JavaScript is a programming language that was created at Netscape as a scripting tool to manipulate web pages inside their browser, Netscape Navigator.
Part of the business model of Netscape was to sell Web Servers, which included an environment called “Netscape LiveWire”, which could create dynamic pages using server-side JavaScript. So the idea of server-side JavaScript was not introduced by Node.js, it’s old just like JavaScript — but at the time it was not successful.
One key factor that led to the rise of Node.js was timing. A few years ago, JavaScript was starting to be considered a serious language, thanks for the “Web 2.0” applications that showed the world what a modern experience on the web could be like (think Google Maps or GMail).
The JavaScript engines performance bar raised considerably thanks to the browser competition battle, which is still going strong. Development teams behind each major browser work hard every day to give us better performance, which is a huge win for JavaScript as a platform. Chrome V8, the engine that Node.js uses under the hood, is one of those and in particular it’s the Chrome JavaScript engine.
But of course, Node.js is not popular just because of pure luck or timing. It introduced much innovative thinking on how to program in JavaScript on the server.
2009
Node.js is born
The first form of npm is created
2010
2011
npm hits 1.0
Big companies start adopting Node: LinkedIn, Uber
Hapi is born
2012
Adoption continues very rapidly
2013
First big blogging platform using Node.js: Ghost
Koa is born
2014
Big drama: IO.js is a major fork of Node.js, with the goal of introducing ES6 support and move faster
2015
IO.js is merged back into Node.js
npm introduces private modules
Node 4 (no 1, 2, 3 versions were previously released)
2016
Yarn is born: Node 6
2017
npm focuses more on security: Node 8
V8 introduces Node in its testing suite, officially making Node a target for the JavaScript engine, in addition to Chrome
3 billion npm downloads every week
2018
Node 10
mjs experimental support
How to install Node.js
How you can install Node.js on your system: a package manager, the official website installer or nvm
Node.js can be installed in different ways. This post highlights the most common and convenient ones.
Official packages for all the major platforms are available here.
One very convenient way to install Node.js is through a package manager. In this case, every operating system has its own.
On macOS, Homebrew is the de-facto standard, and — once installed — allows to install Node.js very easily, by running this command in the CLI:
brew install node
Other package managers for Linux and Windows are listed here.
nvm is a popular way to run Node.js. It allows you to easily switch the Node.js version, and install new versions to try and easily rollback if something breaks, for example.
It is also very useful to test your code with old Node.js versions.
My suggestion is to use the official installer if you are just starting out and you don’t use Homebrew already. Otherwise, Homebrew is my favorite solution.
How much JavaScript do you need to know to use Node.js?
If you are just starting out with JavaScript, how deeply do you need to know the language?
As a beginner, it’s hard to get to a point where you are confident enough in your programming abilities.
While learning to code, you might also be confused at where does JavaScript end, and where Node.js begins, and vice versa.
I would recommend you to have a good grasp of the main JavaScript concepts before diving into Node.js:
- Lexical Structure
- Expressions
- Types
- Variables
- Functions
- this
- Arrow Functions
- Loops
- Loops and Scope
- Arrays
- Template Literals
- Semicolons
- Strict Mode
- ECMAScript 6, 2016, 2017
With those concepts in mind, you are well on your road to become a proficient JavaScript developer, in both the browser and in Node.js.
The following concepts are also key to understand asynchronous programming, which is one fundamental part of Node.js:
- Asynchronous programming and callbacks
- Timers
- Promises
- Async and Await
- Closures
- The Event Loop
Luckily I wrote a free ebook that explains all those topics, and it’s called JavaScript Fundamentals. It’s the most compact resource you’ll find to learn all of this.
Differences between Node.js and the Browser
How writing JavaScript application in Node.js differs from programming for the Web inside the browser.
Both the browser and Node use JavaScript as their programming language.
Building apps that run in the browser is a completely different thing than building a Node.js application.
Despite the fact that it’s always JavaScript, there are some key differences that make the experience radically different.
A front-end developer that writes Node.js apps has a huge advantage — the language is still the same.
You have a huge opportunity because we know how hard it is to fully, deeply learn a programming language. By using the same language to perform all your work on the web — both on the client and on the server — you’re in a unique position of advantage.
What changes is the ecosystem.
In the browser, most of the time what you are doing is interacting with the DOM, or other Web Platform APIs like Cookies. Those do not exist in Node.js, of course. You don’t have the document
, window
and all the other objects that are provided by the browser.
And in the browser, we don’t have all the nice APIs that Node.js provides through its modules, like the file system access functionality.
Another big difference is that in Node.js you control the environment. Unless you are building an open source application that anyone can deploy anywhere, you know which version of Node.js you will run the application on. Compared to the browser environment, where you don’t get the luxury to choose what browser your visitors will use, this is very convenient.
This means that you can write all the modern ES6–7–8–9 JavaScript that your Node version supports.
Since JavaScript moves so fast, but browsers can be a bit slow and users a bit slow to upgrade — sometimes on the web, you are stuck using older JavaScript/ECMAScript releases.
You can use Babel to transform your code to be ES5-compatible before shipping it to the browser, but in Node.js, you won’t need that.
Another difference is that Node.js uses the CommonJS module system, while in the browser we are starting to see the ES Modules standard being implemented.
In practice, this means that for the time being you use require()
in Node.js and import
in the browser.
The V8 JavaScript Engine
V8 is the name of the JavaScript engine that powers Google Chrome. It’s the thing that takes our JavaScript and executes it while browsing with Chrome.
V8 provides the runtime environment in which JavaScript executes. The DOM, and the other Web Platform APIs are provided by the browser.
The cool thing is that the JavaScript engine is independent by the browser in which it’s hosted. This key feature enabled the rise of Node.js. V8 was chosen for being the engine chosen by Node.js back in 2009, and as the popularity of Node.js exploded, V8 became the engine that now powers an incredible amount of server-side code written in JavaScript.
The Node.js ecosystem is huge and thanks to it V8 also powers desktop apps, with projects like Electron.
Other JS engines
Other browsers have their own JavaScript engine:
and many others exist as well.
All those engines implement the ECMA ES-262 standard, also called ECMAScript, the standard used by JavaScript.
The quest for performance
V8 is written in C++, and it’s continuously improved. It is portable and runs on Mac, Windows, Linux and several other systems.
In this V8 introduction, I will ignore the implementation details of V8. They can be found on more authoritative sites, including the V8 official site, and they change over time, often radically.
V8 is always evolving, just like the other JavaScript engines around, to speed up the Web and the Node.js ecosystem.
On the web, there is a race for performance that’s been going on for years, and we (as users and developers) benefit a lot from this competition because we get faster and more optimized machines year after year.
Compilation
JavaScript is generally considered an interpreted language, but modern JavaScript engines no longer just interpret JavaScript, they compile it.
This happens since 2009 when the SpiderMonkey JavaScript compiler was added to Firefox 3.5, and everyone followed this idea.
JavScript is internally compiled by V8 with just-in-time (JIT) compilation to speed up the execution.
This might seem counter-intuitive,. But since the introduction of Google Maps in 2004, JavaScript has evolved from a language that was generally executing a few dozens of lines of code to complete applications with thousands to hundreds of thousands of lines running in the browser.
Our applications now can run for hours inside a browser, rather than being just a few form validation rules or simple scripts.
In this new world, compiling JavaScript makes perfect sense because while it might take a little bit more to have the JavaScript ready, once done it’s going to be much more performant that purely interpreted code.
How to exit from a Node.js program
There are various ways to terminate a Node.js application.
When running a program in the console you can close it with ctrl-C
, but what I want to discuss here is programmatically exiting.
Let’s start with the most drastic one, and see why you’re better off not using it.
The process
core module is provides a handy method that allows you to programmatically exit from a Node.js program: process.exit()
.
When Node.js runs this line, the process is immediately forced to terminate.
This means that any callback that’s pending, any network request still being sent, any file system access, or processes writing to stdout
or stderr
— all is going to be ungracefully terminated right away.
If this is fine for you, you can pass an integer that signals the operating system the exit code:
process.exit(1)
By default the exit code is 0
, which means success. Different exit codes have different meaning, which you might want to use in your own system to have the program communicate to other programs.
You can read more on exit codes here.
You can also set the process.exitCode
property:
process.exitCode = 1
and when the program will later end, Node.js will return that exit code.
A program will gracefully exit when all the processing is done.
Many times with Node.js we start servers, like this HTTP server:
const express = require('express')const app = express()
app.get('/', (req, res) => { res.send('Hi!')})
app.listen(3000, () => console.log('Server ready'))
This program is never going to end. If you call process.exit()
, any currently pending or running request is going to be aborted. This is not nice.
In this case you need to send the command a SIGTERM
signal, and handle that with the process signal handler:
Note: process
does not require a require
, it's automatically available.
const express = require('express')
const app = express()
app.get('/', (req, res) => { res.send('Hi!')})
app.listen(3000, () => console.log('Server ready'))
process.on('SIGTERM', () => { app.close(() => { console.log('Process terminated') })})
What are signals? Signals are a Portable Operating System Interface (POSIX) intercommunication system: a notification sent to a process in order to notify it of an event that occurred.
SIGKILL
is the signals that tells a process to immediately terminate, and would ideally act like process.exit()
.
SIGTERM
is the signals that tells a process to gracefully terminate. It is the signal that's sent from process managers like upstart
or supervisord
and many others.
You can send this signal from inside the program, in another function:
process.kill(process.pid, 'SIGTERM')
Or from another Node.js running program, or any other app running in your system that knows the PID of the process you want to terminate.
How to read environment variables from Node.js
The process
core module of Node provides the env
property which hosts all the environment variables that were set at the moment the process was started.
Here is an example that accesses the NODE_ENV
environment variable, which is set to development
by default.
process.env.NODE_ENV // "development"
Setting it to production
before the script runs will tell Node.js that this is a production environment.
In the same way you can access any custom environment variable you set.
Where to host a Node.js app
A Node.js application can be hosted in a lot of places, depending on your needs.
Here is a non-exhaustive list of the options you can explore when you want to deploy your app and make it publicly accessible.
I will list the options from simplest and constrained to more complex and powerful.
Simplest option ever: local tunnel
Even if you have a dynamic IP, or you’re under a NAT, you can deploy your app and serve the requests right from your computer using a local tunnel.
This option is suited for some quick testing, demo a product or sharing of an app with a very small group of people.
A very nice tool for this, available on all platforms, is ngrok.
Using it, you can just type ngrok PORT
and the PORT you want is exposed to the internet. You will get a ngrok.io domain, but with a paid subscription you can get a custom URL as well as more security options (remember that you are opening your machine to the public Internet).
Another service you can use is localtunnel.
Zero configuration deployments
Glitch
Glitch is a playground and a way to build your apps faster than ever, and see them live on their own glitch.com subdomain. You cannot currently have a a custom domain, and there are a few restrictions in place, but it’s really great to prototype. It looks fun (and this is a plus), and it’s not a dumbed down environment — you get all the power of Node.js, a CDN, secure storage for credentials, GitHub import/export and much more.
Provided by the company behind FogBugz and Trello (and co-creators of Stack Overflow).
I use it a lot for demo purposes.
Codepen
Codepen is an amazing platform and community. You can create a project with multiple files, and deploy it with a custom domain.
Serverless
A way to publish your apps, and have no server at all to manage, is Serverless. Serverless is a paradigm where you publish your apps as functions, and they respond on a network endpoint (also called FAAS — Functions As A Service).
To very popular solutions are:
They both provide an abstraction layer to publishing on AWS Lambda and other FAAS solutions based on Azure or the Google Cloud offering.
PAAS
PAAS stands for Platform As A Service. These platforms take away a lot of things you should otherwise worry about when deploying your application.
Zeit Now
Zeit is an interesting option. You just type now
in your terminal, and it takes care of deploying your application. There is a free version with limitations, and the paid version is more powerful. You simply forget that there’s a server, you just deploy the app.
Nanobox
Heroku
Heroku is an amazing platform.
Microsoft Azure
Azure is the Microsoft Cloud offering.
Google Cloud Platform
Google Cloud is an amazing structure for your apps.
Virtual Private Server
In this section you find the usual suspects, ordered from more user friendly to less user friendly:
- Amazon Web Services, in particular I mention Amazon Elastic Beanstalk as it abstracts away a little bit the complexity of AWS.
Since they provide an empty Linux machine on which you can work, there is no specific tutorial for these.
There are lots more options in the VPS category, those are just the ones I used and I would recommend.
Bare metal
Another solution is to get a bare metal server, install a Linux distribution, connect it to the internet (or rent one monthly, like you can do using the Vultr Bare Metal service)
How to use the Node.js REPL
REPL stands for Read-Evaluate-Print-Loop, and it’s a great way to explore the Node.js features in a quick way.
The node
command is the one we use to run our Node.js scripts:
node script.js
If we omit the filename, we use it in REPL mode:
node
If you try it now in your terminal, this is what happens:
❯ node>
the command stays in idle mode and waits for us to enter something.
Tip: if you are unsure how to open your terminal, Google “How to open terminal on <your operating system>”.
The REPL is waiting for us to enter some JavaScript code.
Start simple and enter:
> console.log('test')testundefined>
The first value, test
, is the output we told the console to print, then we get undefined which is the return value of running console.log()
.
We can now enter a new line of JavaScript.
Use the tab to autocomplete
The cool thing about the REPL is that it’s interactive.
As you write your code, if you press the tab
key the REPL will try to autocomplete what you wrote to match a variable you already defined or a predefined one.
Exploring JavaScript objects
Try entering the name of a JavaScript class, like Number
, add a dot and press tab
.
The REPL will print all the properties and methods you can access on that class:

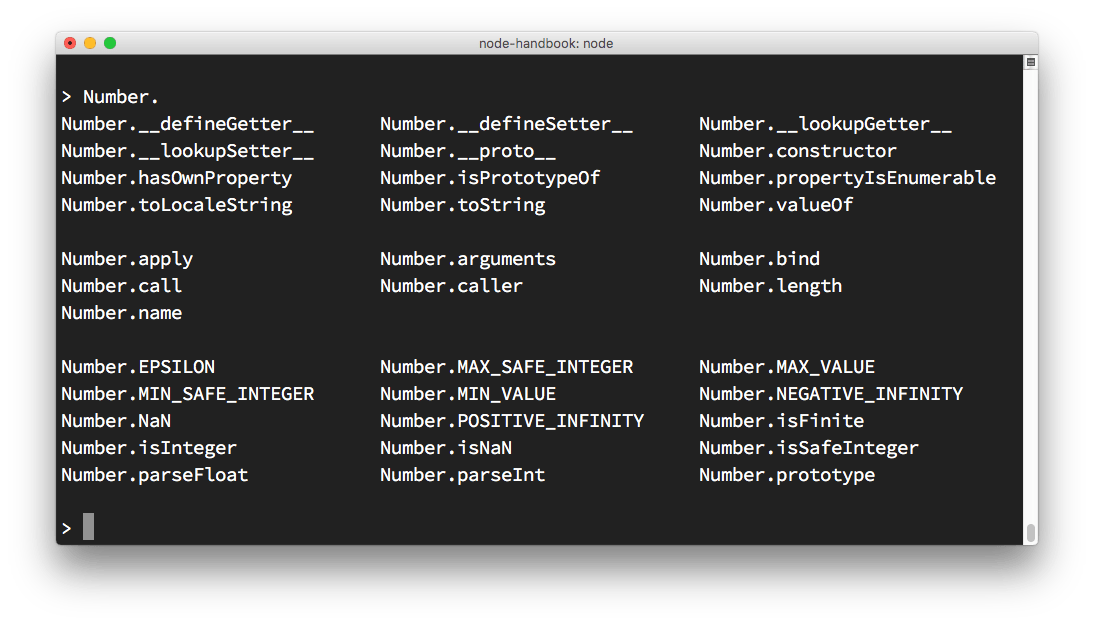
Explore global objects
You can inspect the globals you have access to by typing global.
and pressing tab
:


The _ special variable
If after some code you type _
, that is going to print the result of the last operation.
Dot commands
The REPL has some special commands, all starting with a dot .
. They are
.help
: shows the dot commands help.editor
: enables editor more, to write multiline JavaScript code with ease. Once you are in this mode, enter ctrl-D to run the code you wrote..break
: when inputting a multi-line expression, entering the .break command will abort further input. Same as pressing ctrl-C..clear
: resets the REPL context to an empty object and clears any multi-line expression currently being input..load
: loads a JavaScript file, relative to the current working directory.save
: saves all you entered in the REPL session to a file (specify the filename).exit
: exists the repl (same as pressing ctrl-C two times)
The REPL knows when you are typing a multi-line statement without the need to invoke .editor
.
For example if you start typing an iteration like this:
[1, 2, 3].forEach(num => {
and you press enter
, the REPL will go to a new line that starts with 3 dots, indicating you can now continue to work on that block.
... console.log(num)... })
If you type .break
at the end of a line, the multiline mode will stop and the statement will not be executed.
Node.js, accept arguments from the command line
How to accept arguments in a Node.js program passed from the command line
You can pass any number of arguments when invoking a Node.js application using:
node app.js
Arguments can be standalone or have a key and a value.
For example:
node app.js flavio
or
node app.js name=flavio
This changes how you will retrieve this value in the Node.js code.
The way you retrieve it is using the process
object built into Node.js.
It exposes an argv
property, which is an array that contains all the command line invocation arguments.
The first argument is the full path of the node
command.
The second element is the full path of the file being executed.
All the additional arguments are present from the third position going forward.
You can iterate over all the arguments (including the node path and the file path) using a loop:
process.argv.forEach((val, index) => { console.log(`${index}: ${val}`)})
You can get only the additional arguments by creating a new array that excludes the first 2 params:
const args = process.argv.slice(2)
If you have one argument without an index name, like this:
node app.js flavio
you can access it using
const args = process.argv.slice(2)args[0]
In this case:
node app.js name=flavio
args[0]
is name=flavio
, and you need to parse it. The best way to do so is by using the minimist
library, which helps dealing with arguments:
const args = require('minimist')(process.argv.slice(2))args['name'] //flavio
Output to the command line using Node.js
How to print to the command line console using Node.js, from the basic console.log to more complex scenarios
Basic output using the console module
Node.js provides a console
module which provides tons of very useful ways to interact with the command line.
It is basically the same as the console
object you find in the browser.
The most basic and most used method is console.log()
, which prints the string you pass to it to the console.
If you pass an object, it will render it as a string.
You can pass multiple variables to console.log
, for example:
const x = 'x'const y = 'y'console.log(x, y)
and Node.js will print both.
We can also format pretty phrases by passing variables and a format specifier.
相關推薦
The definitive Node.js handbook
Note: you can get a PDF, ePub, or Mobi version of this handbook for easier reference, or for reading on your Kindle or tablet.Table of ContentsIntroduction
[WASM] Run WebAssembly in Node.js using the node-loader
WebAssembly is great for targeting performance bottlenecks in the browser. Now with node-loader, we can do the same on the server through Node.js While No
Create a Node.js App Using the Express Application Generator Tool
1) Install Express dependencies, create the project directory, and run the generatornpm i -g express express-generatormkdir express-projectcd express-proje
The Node.js Foundation and JS Foundation Announce an Intent to Merge (A Message from the Boards…
The Node.js Foundation and JS Foundation Announce an Intent to Merge (A Message from the Boards and a FAQ around the Announcement)*The introduction of this
The Node.js Ecosystem Is Chaotic and Insecure
The Node.js Ecosystem Is Chaotic and InsecureA modern web developer at workIt seems like only yesterday we had the “left-pad” fiasco where Azer Koçulu ende
Key figures from the Node.js Foundation user survey
Key figures from the Node.js Foundation user surveyA couple days ago, the Node.js Foundation released its first-ever Node.js User Survey Report. It is choc
Node.js使用MongoDB3.4+Access control is not enabled for the database解決方案
今天使用MongoDB時遇到了一些問題 建立資料庫連線時出現了warnings 出現這個警告的原因是新版本的MongDB為了讓我們建立一個安全的資料庫 必須要進行驗證 後來在外網找到了答案 解決方案如下: 建立管理員 use admin d
node.js design patterns (the arrow function)
大家首先來看一片程式碼: 這個程式碼很簡單 定義了一個簡單的 greeter 原型 、 接受name作為引數。然後再原型中加入了greet 方法 例項化之後傳入引數呼叫 greet方法。打印出hello world function DelayedG
node.js對象數據類型
js對象 arr 基本 strong doc 九九乘法 oca 自定義 number 在這裏復習下前端JS的數據類型:前端JS中的數據類型: 1.基本/原生/值類型 string、number、boolean、null、undefined 2.引用/對象
node.js(連接mysql)
權限 alt 第三方 定義 let creat 服務器 dcl local mysql語句中的SQL sql語句中的分類: ---DDL:(data define language)定義數據列(create,drop,alter,truncate)
Node.js Path 模塊
工具 詳細 模塊 module tro ebp dex ble put var path = require(‘path‘); module.exports = { entry: ‘./app/index.js‘, output: { filename:
window下安裝nvm、node.js、npm的步驟
http cnp node 自帶 ack pre 最新 test 通過 1.下載nvm(nodejs版本管理工具) https://github.com/coreybutler/nvm-windows/releases 下載nvm-noinstall.zip,解壓即可使用2
node.js cannot find module 'mysql'
nod 分享 warn npm oam 如果 pac erro .com nodejs cannot find module ‘mysql‘ 問題分析 在windows平臺下,測試nodejs連接mysql數據庫。 首先 在控制臺中安裝mysql依賴包 一開始我是
安裝node.js
進制 idt inux none class next 獲取 figure acl 本安裝教程以Node.js v4.4.3 LTS(長期支持版本)版本為例。 Node.js安裝包及源碼下載地址為:https://nodejs.org/en/download/。 你
antd + node.js + mongoose小總結
node push cse define oos 新博客 mongoose exe ant 最近開發太忙,都沒時間更新博客,想通過這篇博客總結一下相關經驗,以備後續能用到: 一、antd 1.onChange of undefined問題:可能是頁面中表單取了相同的名稱,也
Node.js的Buffer那些你可能不知道的用法
ins min 來看 imu write work and sse ase 在大多數介紹Buffer的文章中,主要是圍繞數據拼接和內存分配這兩方面的。比如我們使用fs模塊來讀取文件內容的時候,返回的就是一個Buffer: fs.readFile(‘filename‘, fu
基於原生JS+node.js+mysql打造的簡易前後端分離用戶登錄系統
power 3.2 80端口 文檔 type ima 原生 倉庫 json 一、登錄頁面 這個沒什麽說的,就放兩張圖 二、服務器端 用express(文檔)搭建服務器,數據褲用mysql(基礎語句),新建一個users,再新建一張users表,我們用這張表。 服務器主要是
node.js(一)
io模型 對象 交互 開發 理解 後端 google 異步io tex 一、nodejs是什麽?? 我們知道JavaScript是運行在瀏覽器中的,瀏覽器為它提供了一個上下文(context),從而讓JavaScript得以解析執行。 nodeJS其實可以這麽理解,它是另
node.js的Buffer類
cee source 3.3 encoding all 個數 p s lis other 1、創建 創建一個長度為256的Buffer實例:var buf = new Buffer(256); 創建一個數組:var buf = new Buffer([10, 20, 30,
node.js利用captchapng模塊實現圖片驗證碼
parse math style ase 圖片驗證碼 all pre 驗證 parseint 安裝captchapng模塊 npm install captchapng nodejs中使用 var express = require(‘express‘); v