How to Create a Cordova Plugin from Scratch
How to Create a Cordova Plugin from Scratch

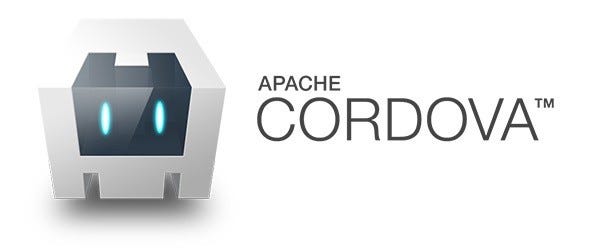
If you heading here, that means you were searching for something or somehow we create custom Cordova plugin to meet your needs, for accessibility into phone features, for which you cannot find any plugins existing, If you thought so, then you are at the right place, here I would be creating a custom Cordova plugin through the plugman. Need not worry, I would be taking you step by step how to create a custom Cordova plugin and use in Cordova application, I would be cooking a simple application.
Simple Recipe For Custom Cordova Plugin
The application, on click of a button in Cordova application, would be showing the native-android/android dialogue to pick the date from the user and display to the user in Cordova application date picked by user in paragraph tag.
plugin responsibilities include
- Receive default date or no date from the user (set default by its own) and set it to the native/android widget(here date picker), that is invoked from the plugin, by the Cordova application.
- Send Or Output the JsonObject with the date user have selected from the native/android widget(here date picker) to the user.
application responsibilities include
- Send default date or no date from the user to the Cordova plugin, in form of the JSON object.
- Show / Handle the JSON Object received from the Cordova plugin.
your responsibilities for application and plugin should be clean and clear otherwise you would be confused about the app and plugin outputs and inputs till the end, and the code would be least reusable .SO MAKE IT CLEAN.
Let's start coding.
$: cordova create datepicker com.datepicker datepickerapp
This will create a new project, Cordova application, this application is the base application for, our the plugin that we will create.
This application is kind of the application tester for the Cordova plugin, that would be generic in all its nature.

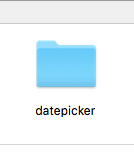
Now let's create a plugin that, this could be done with the simple command, here I would be naming the plugin as “datepickercordovaplugin” , I appended the Cordova plugin at the end, so that it is more intuitive.
For creating the plugin, we first need to install plugman, If you don’t have it, you can install it, very easily and in minutes, by executing the following command.
npm i plugman
$: plugman create — name datepickercordovaplugin — plugin_id com.kuldeep.datepickercordovaplugin — plugin_version 1.0.0

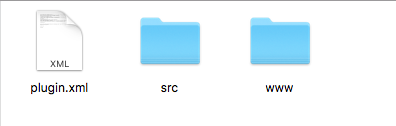
Src, for now, is empty, so in order to create the plugin we need to add a platform for which, we are creating the plugin, for now, I am creating Only for android for simplicity, so execute the following command.
$: plugman platform add — platform_name android


Now, let's make our hands dirty by writing some Java☕(for android) and JS?(for Cordova interface to the plugin)Code.
Let's start.
I am first adding the JAVA Code, datepickercordovaplugin.java , Which was created when the plugman added the platform to the datepicker.
Hey, I am doing everything Visual Studio Code, its easy for me, use the editor as per your convenience.


“datepickercordovaplugin.java” add the following code into, it much of the code is self-explanatory with comments.
package com.kuldeep.datepickercordovaplugin;
import android.app.DatePickerDialog;
import android.widget.DatePicker;
import org.apache.cordova.CallbackContext;
import org.apache.cordova.CordovaPlugin;
import org.apache.cordova.PluginResult;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import java.util.Calendar;
/**
* Cordova main class , which could receive call from the javascript file.
* this class is responsible for call to the other java file and source code.
*
* this @class datepicker would be calling android platfrom datepicker and will result
* in jsonobject with date selected by the user.
* @result {
* "date":31/07/1994
* }
*/
public class datepickercordovaplugin extends CordovaPlugin {
private Calendar calendar;
PluginResult result = null;
private CallbackContext callbackContext = null;
private int year,month,day;
@Override
public boolean execute(String action, JSONArray args, CallbackContext callbackContext) throws JSONException {
this.callbackContext = callbackContext;
if (action.equals("showDateMethod")) {
if(args.length() != 0){
//when the default , date is provided by the first json object of the array, and array contain the json object.
year = args.getJSONObject(0).getInt("year");
month = args.getJSONObject(0).getInt("month");
day = args.getJSONObject(0).getInt("day");
DatePickerShow(999,year, month+1, day);
//for now result is no_result for the plugin and call back is true, hence resukt would be below,
//sent after use input
PluginResult result = new PluginResult(PluginResult.Status.NO_RESULT);
result.setKeepCallback(true);
}
else{
//when the default , date is not provided by the first json object of the array, and array is empty.
calendar = Calendar.getInstance();
year = calendar.get(Calendar.YEAR);
month = calendar.get(Calendar.MONTH);
day = calendar.get(Calendar.DAY_OF_MONTH);
this.DatePickerShow(999,year, month+1, day);
//for now result is no_result for the plugin and call back is true, hence resukt would be below,
//sent after use input
PluginResult result = new PluginResult(PluginResult.Status.NO_RESULT);
result.setKeepCallback(true);
}
return true;
}
return false;
}
/**
*
* @param id
* @param year
* @param month
* @param day
*/
protected void DatePickerShow(int id,int year,int month,int day) {
if (id == 999) {
//would invoke the default
// datepicker if the android platform, with the date provided in
//parameter of the function.
DatePickerDialog datePicker = new DatePickerDialog(this.cordova.getActivity(), new DatePickerDialog.OnDateSetListener() {
@Override
public void onDateSet(DatePicker view, int year, int monthOfYear, int dayOfMonth) {
String date = String.valueOf(dayOfMonth) + "/" + String.valueOf(monthOfYear+1)
+ "/" + String.valueOf(year);
setDate(date);
}
}, year, month, day);
datePicker.show();
}
}
/**
*
* @param date
* @result is the Plugin result, on callback result.
*/
protected void setDate(String date){
//Once the date is selected from the user, date would be sent
//here and the new JSON Object would be created for the result of the plugin
//would be sent to the user in cordova, where JS function give the result to the user.
JSONObject jsonObject = new JSONObject();
try {
jsonObject.put("date",date);
} catch (JSONException e) {
e.printStackTrace();
}
result = new PluginResult(PluginResult.Status.OK,
jsonObject);
result.setKeepCallback(false);
if (callbackContext != null) {
callbackContext.sendPluginResult(result);
//no more result , hence the context is cleared.
callbackContext = null;
}
}
}
If you are comfortable with native Android code, then the code is much easy to understand, if you are unable to understand them, just understand by the code intuitive names and comments summary or just drop me a message below.
I am now adding the JS Code, datepickercordovaplugin.js, Which was created when the plugman created the project.


This is a generic JS file, for any platform, for Android here in the example is much easier to understand.
“datepickercordovaplugin.js”
/**
* @author Kuldeep Kumar
* @summary js interface for the cordova plugin
*/
var exec = require('cordova/exec');
//I have customized the code for my convience
//easy to understand , you can it remain the same to.
var testing = function(){};
/**
* This function is called from the cordova app @showDatePicker
* and this will invoke the active #showDateMethod in datepickercordovaplugin
* @param {} arg0 would be provided by the user from the cordova application
* @param {*} success
* @param {*} error
*/
testing.prototype.showDatePicker = function (arg0, success, error) {
exec(success, error, 'datepickercordovaplugin', 'showDateMethod', [arg0]);
};
testing.install = function () {
if (!window.plugins) {
window.plugins = {};
}
window.plugins = new testing();
return window.plugins;
};
cordova.addConstructor(testing.install);
We are almost ready,?? the except we have to know about one more, very important file, i.e “plugin.xml”

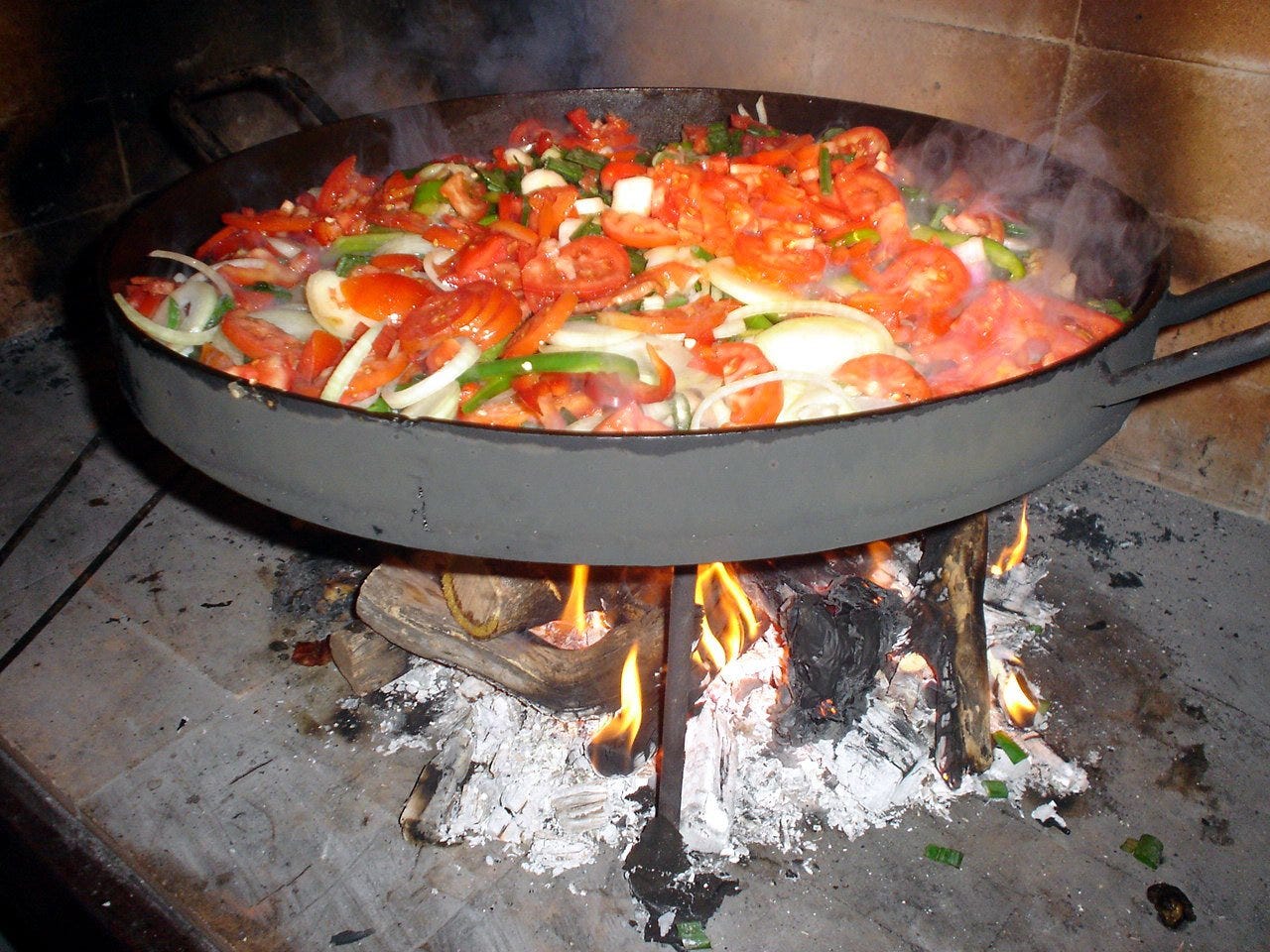
plugin.xml is very important in the sense, it is the first file,that would be read by Cordova application, while installing the Cordova plugin into the cordova app.
I have included clear comments about everything you need to know about it.
“plugin.xml”
<?xml version='1.0' encoding='utf-8'?>
<!-- autogenerated info if you for the plugin. -->
<plugin id="com.kuldeep.datepickercordovaplugin" version="1.0.0" xmlns="http://apache.org/cordova/ns/plugins/1.0" xmlns:android="http://schemas.android.com/apk/res/android">
<!-- name of the plugin -->
<name>datepickercordovaplugin</name>
<!-- js path of the plugin -->
<js-module name="datepickercordovaplugin" src="www/datepickercordovaplugin.js">
<!-- cordova wireing of the plugin -->
<clobbers target="cordova.plugins.datepickercordovaplugin" />
</js-module>
<!-- android platform specifications -->
<platform name="android">
<!-- autogenerated content for after install specification -->
<config-file parent="/*" target="res/xml/config.xml">
<feature name="datepickercordovaplugin">
<param name="android-package" value="com.kuldeep.datepickercordovaplugin.datepickercordovaplugin" />
</feature>
</config-file>
<!-- these tags gona a contain if, you want to put anything inside the AndroidManifest file -->
<config-file parent="/*" target="AndroidManifest.xml">
</config-file>
<!-- paths of various JAVA files in your plugin and there after install specification according to the package name, you provided -->
<!-- here we mean take .java file from src->android->datepickercordovaplugin.java into the src->com->kuldeep->datepickercordovaplugin->datepickercordovaplugin->datepickercordovaplugin.java -->
<!-- for after install effect please see inside your platform android folder inside that go to src and then follow your package folder -->
<source-file src="src/android/datepickercordovaplugin.java" target-dir="src/com/kuldeep/datepickercordovaplugin/datepickercordovaplugin" />
</platform>
</plugin>
Complete documentation of it Plugin.xml you can find on
Otherwise just comment what you want to know, I will try my best to resolve it.?
You are done with the coding part, for now, lets put it in a plate of cordova and use it.?️
for installation use the following code for the same.
$: plugman install — platform android — project platforms/android — plugin “ “fullpathtowards your plugin / datepickercordovaplugin”
Now how to invoke the plugin is much easier than, any of the above task, it is as simple as eating the cupcake.?
//parameter for the call of the datepicker function.var temp = {
"year":1994,
"month":3,
"day":16
};//invoking the plugin, by window object as i have wired from the //window object. and then the function name.
window.plugins.showDatePicker(temp, function (s) {
console.log(s);//whatever is returned by the plugin.
}, function (e) {
console.log(e);
});
Hope you were able to include the code on the right place still have doubts then, just use my code, from git repository link given below.
Now just run the project.
$: cordova run android