C++第4章 實驗
阿新 • • 發佈:2019-01-02
P120.3:
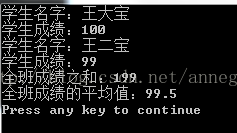
實驗3: 設計一個用來表示直角座標系的LOCATION類,在主程式中建立類LOCATION的兩個物件A和B,要求A的座標點在第3象限,B的座標點在第2象限,分別採用成員函式和友元函式計算給定兩個座標點之間的距離。
#include<iostream> #include<cmath> using namespace std; class Location{ public: double a,b,d1,d2; Location(double a ,double b); double getx(); double gety(); double distance(Location&,Location&); friend double distance1(Location&,Location&); private: double x,y; }; Location::Location(double a,double b) { x=a; y=b; } double Location:: getx() { return x; } double Location:: gety() { return y; } double Location::distance(Location&c,Location&d) { d1=sqrt((c.x-d.x)*(c.x-d.x)+(c.y-d.y)*(c.y-d.y)); cout<<"Distance1="<<d1<<endl; return 0;} double distance1(Location& c,Location& d) { double d2; d2=sqrt((c.x-d.x)*(c.x-d.x)+(c.y-d.y)*(c.y-d.y)); cout<<"Distance2="<<d2<<endl; return 0;} int main() { Location A(-1,-1); Location B(-1,1); cout<<"A("<<A.getx()<<","<<A.gety()<<") , B("<<B.getx()<<","<<B.gety()<<")"<<endl; A.distance(A,B); distance1(A,B); return 0; }
P121 實驗4:宣告一個student類,在該類中包括一個數據成員score、兩個靜態資料成員total_score和count;還包括一個成員函式account用於設定分數、累計學生的成績之和、累計學生人數,一個靜態成員函式sum用於返回學生的成績之和,另一個靜態資料成員函式average用於求全班成績的平均值。在main函式中,輸入某班同學的成績,並呼叫上述函式求出全班同學的成績之和與平均分。
#include<iostream> #include<string> using namespace std; class student{ public: student(string name1,int score1); void account(); static void sum(); static void average(); private: string name; int score; static float total_score; static float count; static float ave; }; float student:: total_score=0.0; float student:: count=0.0; float student::ave=0.0; student::student(string name1,int score1) { name=name1; score=score1; ++count; total_score=total_score+score; ave=total_score/count;} void student::account() { cout<<"學生名字:"<<name<<endl; cout<<"學生成績:"<<score<<endl;} void student::sum() {cout<<"全班成績之和:"<<total_score<<endl;} void student::average() { cout<<"全班成績的平均值:"<<ave<<endl;} int main() { student stu1("王大寶",100); student stu2("王二寶",99); stu1.account(); stu2.account(); stu2.sum(); stu2.average(); return 0; }