OrmLite資料庫升級
阿新 • • 發佈:2019-01-09
在應用開發過程中,隨著需求的不斷變化,難免會修改資料庫,或是增加一張表,或是增加一個欄位。這就需要對資料庫進行升級,我們只要升級資料庫的版本號,那麼下次使用時就會自動呼叫onUpgrade方法,當然具體的修改需要我們自己來實現。
最簡單粗暴的方法就是將原來的表全部刪除,然後重新建立,之前的程式碼示例中就是這麼做的。
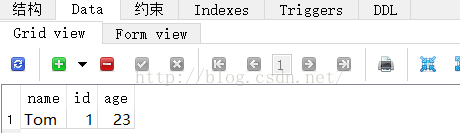
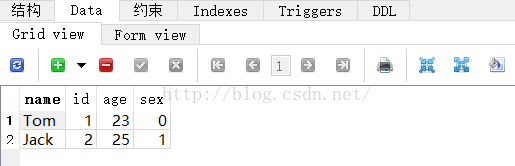
新增的欄位成功添加了,並且預設值為0。
原始碼地址
這樣做的好處呢就是簡單,不容易出現問題,但是會導致使用者資料丟失,體驗就比較差了,最好是針對資料庫的變化做相應的升級處理。 增加一張表 當前資料庫版本為1,現在要增加一張表"table_person",首先建立Person實體類,@Override public void onCreate(SQLiteDatabase database, ConnectionSource connectionSource) { // TODO Auto-generated method stub try { TableUtils.createTable(connectionSource, Student.class); TableUtils.createTable(connectionSource, Profession.class); TableUtils.createTable(connectionSource, Worker.class); } catch (SQLException e) { // TODO Auto-generated catch block e.printStackTrace(); } } @Override public void onUpgrade(SQLiteDatabase database, ConnectionSource connectionSource, int oldVersion, int newVersion) { // TODO Auto-generated method stub try { TableUtils.dropTable(connectionSource, Student.class, true); TableUtils.dropTable(connectionSource, Profession.class, true); TableUtils.dropTable(connectionSource, Worker.class, true); onCreate(database, connectionSource); } catch (SQLException e) { // TODO Auto-generated catch block e.printStackTrace(); } }
資料庫版本號升級為2,@DatabaseTable(tableName = "table_person") public class Person { @DatabaseField(generatedId = true) public int id; @DatabaseField(columnName = "name") public String name; @DatabaseField(columnName = "age") public int age; public Person() { } public Person(String name, int age) { this.name = name; this.age = age; } }
// Database Version
private static final int DB_VERSION = 2;
下次使用資料庫時就會執行onUpgrade方法,傳遞了三個引數,此時oldVersion值為1,newVersion值為2,我們可以據此判斷以做出相應的處理,一定要在此處新增,不能再onCreate中處理,之前也提到過,onCreate的方法只有在第一次建立資料庫中才呼叫,當然也可以手動呼叫,但即使手動呼叫,也必須在onUpgrade方法中。這樣資料庫中就增加了一張表。 還要在Helper類中新增mPersonDao。if (newVersion == 2) { try { TableUtils.createTable(connectionSource, Person.class); } catch (SQLException e) { e.printStackTrace(); } }
public BaseDao<Person, Integer> getPersonDao() {
if (mPersonDao == null) {
mPersonDao = new BaseDaoImpl<>(mContext, Person.class);
}
return mPersonDao;
}
向新建的表裡插入一條記錄,測試這張表是否建立成功。
private void add() {
Person p = new Person("Tom", 23);
Helper.getInstance().getPersonDao().insert(p);
Utils.copyDBToSDcrad();
}
成功插入了一條資料,說明這張表建立成功。
表中新增一個欄位
基本步驟與新增表差不多,也是升級版本號,在onUpgrade中處理。
在Person實體類新增一個新的欄位,
@DatabaseField(columnName = "sex")
public int sex;
資料庫版本號升級到3, // Database Version
private static final int DB_VERSION = 3;
在onUpgrade方法中使用sqlite語句插入這個欄位, if (newVersion == 3) {
try {
getDao(Person.class).executeRaw("ALTER TABLE `table_person` ADD COLUMN sex INTEGER DEFAULT 0;");
Helper.getInstance().reset();
} catch (SQLException e) {
e.printStackTrace();
}
}
新增完欄位後,需要重新生成Dao物件,否則有可能使用之前生成的,導致資料庫操作失敗。 public void reset() {
mStudentDao = new BaseDaoImpl<>(mContext, Student.class);
mProfessionDao = new BaseDaoImpl<>(mContext, Profession.class);
mWorkerDao = new BaseDaoImpl<>(mContext, Worker.class);
mPersonDao = new BaseDaoImpl<>(mContext, Person.class);
}
測試一下, private void add() {
// Person p = new Person("Tom", 23);
// Helper.getInstance().getPersonDao().insert(p);
Person p = new Person("Jack", 25, 1);
Helper.getInstance().getPersonDao().insert(p);
Utils.copyDBToSDcrad();
}
新增的欄位成功添加了,並且預設值為0。
原始碼地址