Check out these useful ECMAScript 2015 (ES6) tips and tricks
阿新 • • 發佈:2019-01-16
Check out these useful ECMAScript 2015 (ES6) tips and tricks
EcmaScript 2015 (aka ES6) has been around for couple of years now, and various new features can be used in clever ways. I wanted to list and discuss some of them, since I think you’ll find them useful.
If you know of other tips, please let me know in the comment and I’ll be happy to add them.
1. Enforcing required parameters
ES6 provides default parameter values that allow you to set some default value to be used if the function is called without that parameter.
In the following example, we are setting the required()
function as the default value for both a
and b
parameters. This means that if either a
b
is not passed, the required()
function is called and you’ll get an error.
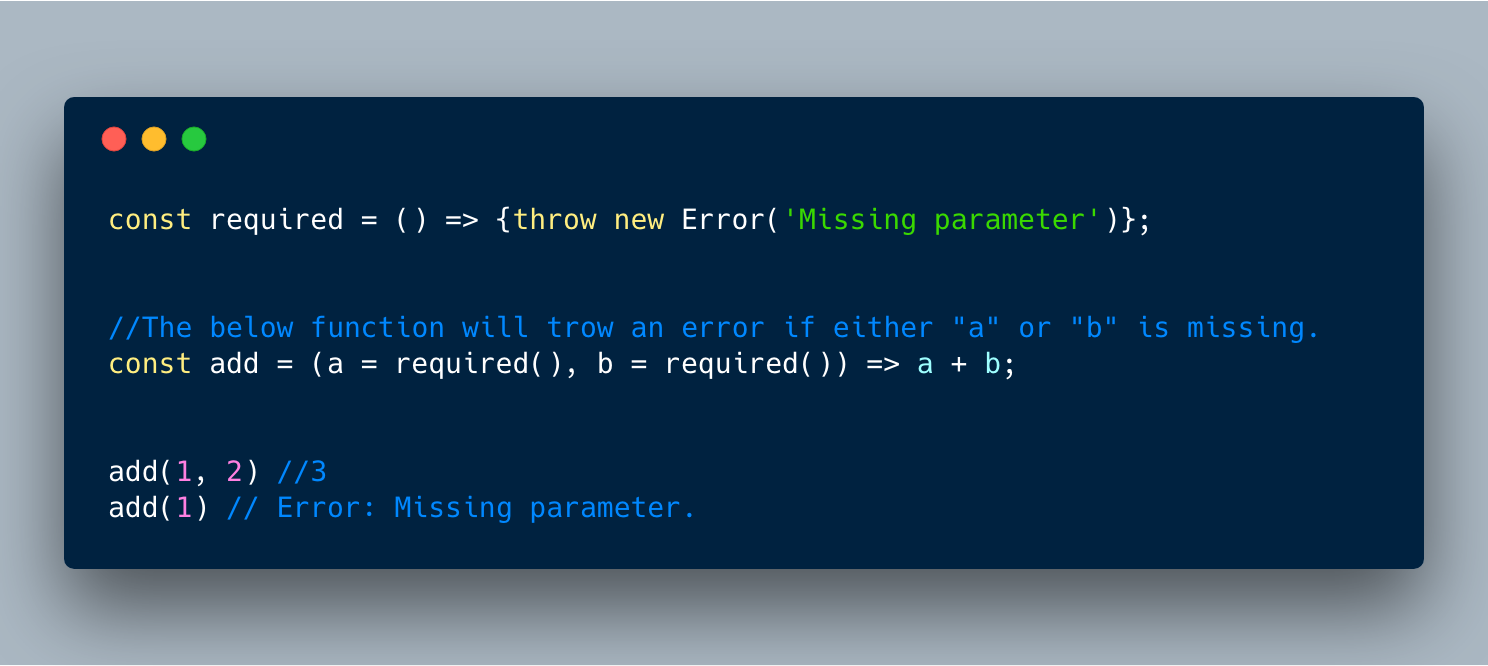
const required = () => {throw new Error('Missing parameter')};
//The below function will trow an error if either "a" or "b" is missing.
const add = (a = required(), b = required()) => a + b;
add(1, 2) //3
add(1) // Error: Missing parameter.
2. The mighty “reduce”
Array’s reduce method is extremely versatile. It is typically used to convert an array of items into a single value. But you can do a lot more with it.