大數高精度之java處理
例題1
原題:
Octal FractionsTime Limit: 1000MS | Memory Limit: 10000K |
Total Submissions: 6648 | Accepted: 3627 |
Description
Fractions in octal (base 8) notation can be expressed exactly in decimal notation. For example, 0.75 in octal is 0.953125 (7/8 + 5/64) in decimal. All octal numbers of n digits to the right of the octal point can be expressed in no more than 3n decimal digits to the right of the decimal point.Write a program to convert octal numerals between 0 and 1, inclusive, into equivalent decimal numerals.
Input
Output
Your output will consist of a sequence of lines of the formwhere the left side is the input (in octal), and the right hand side the decimal (base 10) equivalent. There must be no trailing zeros, i.e. Dm is not equal to 0.
Sample Input
0.75 0.0001 0.01234567
Sample Output
0.75 [8] = 0.953125 [10] 0.0001 [8] = 0.000244140625 [10] 0.01234567 [8] = 0.020408093929290771484375 [10]
Source
java.source:import java.util.*;
import java.io.*;
import java.math.*;
public class da {
public static void main(String args[])
{
BigDecimal a,t,c,ans,tmp;
String s;
Scanner cin=new Scanner(new BufferedInputStream(System.in));
t=BigDecimal.valueOf(8);
while(cin.hasNextLine())
{
s=cin.nextLine();
tmp=t;
ans=BigDecimal.ZERO;
for(int i=2;i<s.length();i++)
{
c=BigDecimal.valueOf(s.charAt(i)-'0');
ans=ans.add(c.divide(tmp));
tmp=tmp.multiply(t);
}
System.out.println(s+" [8] = "+ans+" [10]");
}
}
}
例題2
原題:
Water Treatment PlantsTime Limit: 1000MS | Memory Limit: 10000K |
Total Submissions: 798 | Accepted: 478 |
Description
River polution control is a major challenge that authorities face in order to ensure future clean water supply. Sewage treatment plants are used to clean-up the dirty water comming from cities before being discharged into the river.As part of a coordinated plan, a pipeline is setup in order to connect cities to the sewage treatment plants distributed along the river. It is more efficient to have treatment plants running at maximum capacity and less-used ones switched off for a period. So, each city has its own treatment plant by the river and also a pipe to its neighbouring city upstream and a pipe to the next city downstream along the riverside. At each city's treatment plant there are three choices:
- either process any water it may receive from one neighbouring city, together with its own dirty water, discharging the cleaned-up water into the river;
- or send its own dirty water, plus any from its downstream neighbour, along to the upstream neighbouring city's treatment plant (provided that city is not already using the pipe to send it's dirty water downstream);
- or send its own dirty water, plus any from the upstream neighbour, to the downstream neighbouring city's plant, if the pipe is not being used.
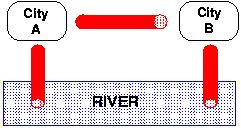
The choices above ensure that:
every city must have its water treated somewhere and
at least one city must discharge the cleaned water into the river.
Let's represent a city discharging water into the river as "V" (a downwards flow), passing water onto its neighbours as ">" (to the next city on its right) or else "<" (to the left). When we have several cities along the river bank, we assign a symbol to each (V, < or >) and list the cities symbols in order. For example, two cities, A and B, can
each treat their own sewage and each discharges clean water into the river. So A's action is denoted V as is B's and we write "VV" ;
or else city A can send its sewage along the pipe (to the right) to B for treatment and discharge, denoted ">V";
or else city B can send its sewage to (the left to) A, which treats it with its own dirty water and discharges (V) the cleaned water into the river. So A discharges (V) and B passes water to the left (<), and we denote this situation as "V<".
We could not have "><" since this means A sends its water to B and B sends its own to A, so both are using the same pipe and this is not allowed. Similarly "<<" is not possible since A's "<" means it sends its water to a non-existent city on its left.
So we have just 3 possible set-ups that fit the conditions:
A B A > B A < B V V V V RIVER~ ~ ~ ~ ~ ~ ~ ~ ~ ~ ~ ~ ~ ~ ~RIVER "VV" ">V" "V<"
If we now consider three cities, we can determine 8 possible set-ups.
Your task is to produce a program that given the number of cities NC (or treatment plants) in the river bank, determines the number of possible set-ups, NS, that can be made according to the rules define above.
You need to be careful with your design as the number of cities can be as large as 100.
Input
The input consists of a sequence of values, one per line, where each value represents the number of cities.Output
Your output should be a sequence of values, one per line, where each value represents the number of possible set-ups for the corresponding number of cities read in the same input line.Sample Input
2 3 20
Sample Output
3 8 102334155
Source
題意:有n個城市,它們由一個汙水處理系統連線著,每個城市可以選擇
1、將左邊城市過來的汙水和右邊城市過來的汙水連同本身的汙水排到河裡 >V<
2、將左邊來的汙水連同自己的汙水排到右邊 >>
3、將右邊來的汙水連同自己的汙水排到左邊 <<
問總共有多少種處理情況,即不同又符合實際的><V組合。
思路:DP+高精度。DP部分,易得最右邊城市的狀態只可能用3種:>V, V, <。故分三種狀態討論,設dp[i][0]為第i個城市的狀態為:> V ,dp[i][1]為:V ,dp[i][2]為:<。由實際流動的可能性可以得到狀態轉移方程:
dp[i][0] = dp[i-1][0] + dp[i-1][1];
dp[i][1] = dp[i-1][0] + dp[i-1][1] + dp[i-1][2];
dp[i][2] = dp[i-1][0] + dp[i-1][1] + dp[i-1][2];
然後可以整理為:dp[i] = 3 * dp[i-1] -dp[i-2]。然後用java的BigInteger預處理就OK了。
My java.source:
import java.util.*;
import java.io.*;
import java.math.*;
public class Main {
public static void main(String args[])
{
Scanner cin=new Scanner(new BufferedInputStream(System.in));
BigInteger dp[]=new BigInteger[111];
dp[1]=BigInteger.valueOf(1);
dp[2]=BigInteger.valueOf(3);
for(int i=3;i<=100;i++)
{
dp[i]=BigInteger.valueOf(3).multiply(dp[i-1]).subtract(dp[i-2]);
}
while(cin.hasNext())
{
int n;
n=cin.nextInt();
System.out.println(dp[n]);
}
}
}
例題3
原題:
NUMBER BASE CONVERSIONTime Limit: 1000MS | Memory Limit: 10000K |
Total Submissions: 4322 | Accepted: 1949 |
Description
Write a program to convert numbers in one base to numbers in a second base. There are 62 different digits:{ 0-9,A-Z,a-z }
HINT: If you make a sequence of base conversions using the output of one conversion as the input to the next, when you get back to the original base, you should get the original number.
Input
The first line of input contains a single positive integer. This is the number of lines that follow. Each of the following lines will have a (decimal) input base followed by a (decimal) output base followed by a number expressed in the input base. Both the input base and the output base will be in the range from 2 to 62. That is (in decimal) A = 10, B = 11, ..., Z = 35, a = 36, b = 37, ..., z = 61 (0-9 have their usual meanings).Output
The output of the program should consist of three lines of output for each base conversion performed. The first line should be the input base in decimal followed by a space then the input number (as given expressed in the input base). The second output line should be the output base followed by a space then the input number (as expressed in the output base). The third output line is blank.Sample Input
8 62 2 abcdefghiz 10 16 1234567890123456789012345678901234567890 16 35 3A0C92075C0DBF3B8ACBC5F96CE3F0AD2 35 23 333YMHOUE8JPLT7OX6K9FYCQ8A 23 49 946B9AA02MI37E3D3MMJ4G7BL2F05 49 61 1VbDkSIMJL3JjRgAdlUfcaWj 61 5 dl9MDSWqwHjDnToKcsWE1S 5 10 42104444441001414401221302402201233340311104212022133030
Sample Output
62 abcdefghiz 2 11011100000100010111110010010110011111001001100011010010001 10 1234567890123456789012345678901234567890 16 3A0C92075C0DBF3B8ACBC5F96CE3F0AD2 16 3A0C92075C0DBF3B8ACBC5F96CE3F0AD2 35 333YMHOUE8JPLT7OX6K9FYCQ8A 35 333YMHOUE8JPLT7OX6K9FYCQ8A 23 946B9AA02MI37E3D3MMJ4G7BL2F05 23 946B9AA02MI37E3D3MMJ4G7BL2F05 49 1VbDkSIMJL3JjRgAdlUfcaWj 49 1VbDkSIMJL3JjRgAdlUfcaWj 61 dl9MDSWqwHjDnToKcsWE1S 61 dl9MDSWqwHjDnToKcsWE1S 5 42104444441001414401221302402201233340311104212022133030 5 42104444441001414401221302402201233340311104212022133030 10 1234567890123456789012345678901234567890
Source
My java.source:
import java.io.*;
import java.text.*;
import java.util.*;
import java.math.*;
public class Main {
public static void main(String[] args)
{
Scanner cin=new Scanner(System.in);
int cas,i,w;
BigInteger sum,t,b1,b2;
char c;
cas=cin.nextInt();
while((cas--)!=0)
{
b1=cin.nextBigInteger();// 讀入大數。
b2=cin.nextBigInteger();
String s=cin.next(); // 讀入字串,無空格,而cin.nextline()有。
sum=BigInteger.valueOf(0);
t=BigInteger.valueOf(1);
for(i=s.length()-1;i>=0;i--)// 先將s轉換為10進位制。
{
c=s.charAt(i);
if(c>='0'&&c<='9')
w=c-'0';
else if(c>='A'&&c<='Z')
w=c-'A'+10;
else
w=c-'a'+36;
sum=sum.add(BigInteger.valueOf(w).multiply(t));
t=t.multiply(b1);
}
int top=0;
int stack[]=new int[200];
while(sum.compareTo(BigInteger.valueOf(0))!=0)// 轉化為b2進位制的數,存在stack[]中。
{
stack[++top]=sum.mod(b2).intValue();
sum=sum.divide(b2);
}
System.out.print(b1+" "+s+"\n"+b2+" ");
if(top==0)
System.out.print(0);// 要注意為0的情況。
while(top!=0)
{
w=stack[top--];
if(w<10)
c=(char)(w+'0');
else if(w<36)
c=(char)(w-10+'A');
else
c=(char)(w-36+'a');
System.out.print(c);
}
System.out.print("\n\n");
}
}
}
例題4
原題:
HeritageTime Limit: 1000MS | Memory Limit: 65536K |
Total Submissions: 7129 | Accepted: 2645 |
Description
Your rich uncle died recently, and the heritage needs to be divided among your relatives and the church (your uncle insisted in his will that the church must get something). There are N relatives (N <= 18) that were mentioned in the will. They are sorted in descending order according to their importance (the first one is the most important). Since you are the computer scientist in the family, your relatives asked you to help them. They need help, because there are some blanks in the will left to be filled. Here is how the will looks:Relative #1 will get 1 / ... of the whole heritage,
Relative #2 will get 1 / ... of the whole heritage,
---------------------- ...
Relative #n will get 1 / ... of the whole heritage.
The logical desire of the relatives is to fill the blanks in such way that the uncle's will is preserved (i.e the fractions are non-ascending and the church gets something) and the amount of heritage left for the church is minimized.
Input
The only line of input contains the single integer N (1 <= N <= 18).Output
Output the numbers that the blanks need to be filled (on separate lines), so that the heritage left for the church is minimized.Sample Input
2
Sample Output
2 3
Source
題意:將一份遺產分成n份,1/X1, 1/X2, 1/X3, ...,1/Xn。 使得X1 >= X2 >= X3 >= ... >= Xn,都為正整數。最後剩下的分給教堂,讓其分得的財產最少,但不能沒有。
思路:貪心+高精度。首先由貪心的思想可以知道,如果剩下1/6的遺產給最後一人和教堂,易得最後一人的得到的遺產為1/7。由這個思想可以列出 1/(Xn-1) = 1 - 1/X1 - 1/X2 -...- 1/X(n-1)。然後這個式子和X(n+1)的式子相消,化簡,可得X(n+1) = Xn * (Xn - 1) + 1。然後就用java的大數A掉。
題意思路註釋轉自:http://blog.sina.com.cn/s/blog_6635898a0100ovom.htmlMy java source:
import java.util.*;
import java.io.*;
import java.math.*;
import java.text.*;
public class Main{
public static void main(String args[])
{
int i,n,cas;
BigInteger ans;
Scanner cin=new Scanner(System.in);
while(cin.hasNext())
{
ans=BigInteger.valueOf(2);
n=cin.nextInt();
for(i=1;i<=n;i++)
{
System.out.println(ans);
ans=ans.multiply(ans.subtract(BigInteger.valueOf(1))).add(BigInteger.valueOf(1));
}
}
}
}
例題5
原題:
Integer InquiryTime Limit: 1000MS | Memory Limit: 10000K |
Total Submissions: 30091 | Accepted: 11718 |
Description
One of the first users of BIT's new supercomputer was Chip Diller. He extended his exploration of powers of 3 to go from 0 to 333 and he explored taking various sums of those numbers.``This supercomputer is great,'' remarked Chip. ``I only wish Timothy were here to see these results.'' (Chip moved to a new apartment, once one became available on the third floor of the Lemon Sky apartments on Third Street.)
Input
The input will consist of at most 100 lines of text, each of which contains a single VeryLongInteger. Each VeryLongInteger will be 100 or fewer characters in length, and will only contain digits (no VeryLongInteger will be negative).The final input line will contain a single zero on a line by itself.
Output
Your program should output the sum of the VeryLongIntegers given in the input.Sample Input
123456789012345678901234567890 123456789012345678901234567890 123456789012345678901234567890 0
Sample Output
370370367037037036703703703670
Source
My java.source:
import java.util.*;
import java.io.*;
import java.math.*;
import java.text.*;
public class Main{
public static void main(String args[])
{
BigInteger num,ans;
Scanner cin=new Scanner(System.in);
ans=BigInteger.valueOf(0);
while(cin.hasNext())
{
num=cin.nextBigInteger();
if(num.compareTo(BigInteger.valueOf(0))==0)
break;
ans=ans.add(num);
}
System.out.println(ans);
}
}
例題6
原題:
Just the FactsTime Limit: 1000MS | Memory Limit: 65536K |
Total Submissions: 8747 | Accepted: 4645 |
Description
The expression N!, read as "N factorial," denotes the product of the first N positive integers, where N is nonnegative. So, for example,N N! 0 1 1 1 2 2 3 6 4 24 5 120 10 3628800
For this problem, you are to write a program that can compute the last non-zero digit of any factorial for (0 <= N <= 10000). For example, if your program is asked to compute the last nonzero digit of 5!, your program should produce "2" because 5! = 120, and 2 is the last nonzero digit of 120.
Input
Input to the program is a series of nonnegative integers not exceeding 10000, each on its own line with no other letters, digits or spaces. For each integer N, you should read the value and compute the last nonzero digit of N!.Output
For each integer input, the program should print exactly one line of output. Each line of output should contain the value N, right-justified in columns 1 through 5 with leading blanks, not leading zeroes. Columns 6 - 9 must contain " -> " (space hyphen greater space). Column 10 must contain the single last non-zero digit of N!.Sample Input
1 2 26 125 3125 9999
Sample Output
1 -> 1 2 -> 2 26 -> 4 125 -> 8 3125 -> 2 9999 -> 8
Source
java.source:
import java.io.*;
import java.util.*;
import java.math.*;
import java.text.*;
public class Main {
public static void main(String args[])
{
BigInteger n,nn;
String s;
int i;
Scanner cin=new Scanner(System.in);
while(cin.hasNext())
{
n=cin.nextBigInteger();
nn=BigInteger.valueOf(1);
for(i=2;i<=n.intValue();i++)
{
nn=nn.multiply(BigInteger.valueOf(i));
}
s=nn.toString();
for(i=s.length()-1;i>=0;i--)
{
if(s.charAt(i)!='0')
{
break;
}
}
System.out.printf("%5d -> %c\n",n,s.charAt(i));
}
}
}
相關推薦
大數高精度之java處理
例題1 原題: Octal Fractions Time Limit: 1000MS Memory Limit: 10000K Total Submissions: 6648 Accepted: 3627 Description Fractions in
Game of Taking Stones(java大數高精度)
Two people face two piles of stones and make a game. They take turns to take stones. As game rules, there are two different methods of tak
JAVA大數高精度
板子一 import java.math.BigDecimal; import java.math.BigInteger; import java.util.Scanner; public class Main { static BigDecimal gold =
Java 大數高精度函式(BigInteger)
Java 提交格式 import .. 標頭檔案 .. public class Main { public static void main(String[] args) { Scanner cin = new Scanner(Sy
JAVA大數高精度練習
<span style="font-family:KaiTi_GB2312;font-size:18px;">import java.math.BigInteger; import java.util.*; import java.io.*; /
JAVA大數高精度應用2
統計[a,b]範圍內有多少個Fib數 import java.math.BigInteger; import java.util.*; import java.io.*; public class Main { public static void main(Stri
高精度乘法 JAVA 和 C++ 版本
本文采用JAVA和C++手動計算大數乘法。 這是是個常見、標準的乘法演算法。簡單易懂,可以多次看記下來。 C++ #include <iostream> #include <vector> #include <string> using n
初識高精度之P1096 HanoiHanoiHanoi雙塔問題
這道題是一道用來練習遞推的經典題目,但是在推出遞推關係式,滿心歡喜將程式碼提交後只通過了四組資料,i can’t believe that;馬上溜去看題解,嗯,一個大牛說這是道水題(沒毛病),用高精度提交就可以了。。。。 高精度???我就乖乖滾去百度了 題目如下 題目描述 給定AAA
高精度之高精度除法(高精除以高精)
好像NOIP並不會用到,但是作為強迫症的我還是堅持學了。高精度除以高精度我所知道的有兩個思路: 手動模擬法 還是手動模擬除法過程,但是注意在截取了被除數的正確片段之後應該試商,即列舉k從1到9看當k等於多少才合適,但是如果每次迴圈都試一邊的話時間複雜度必
高精度之高精度除法(高精除以低精)
一.整除版高精度除法: 思路,手動模擬除法過程,包括餘數用X記錄,每次讀到新位計算出被除數,然後計算。 //高精度除法 整除版 #include<iostream> #in
高精度之高精度乘法
話說高精度乘法真的沒有什麼好介紹的,直接上程式碼: #include<cstdio> #include<cstdlib> #include<algorithm>
高精度之高精度加減法
C++ 該死的高精度加減法 沒看書直接硬上的結果是,一個簡單的高精度減法我用了一個多小時只寫了一個90分的程式碼。之前準備NOIP的時候確實是學了,可惜現在早就還給老師了。 結果回家一看標準程式,頓時有種想哭的趕腳。 先上我的90分程式碼:
java中大數處理和高精度小數處理(so easy)
試用範圍:當對資料處理時,最大的long型不能裝下,這時候就需要採用大數BigInteger來解決 簡單的例子:package cn.hncu.BigNUM; import java.math
Java 使用BigDecimal類處理高精度計算
positive urn 使用 println highlight 轉換 posit exception val Java在java.math包中提供的API類BigDecimal,用來對超過16位有效位的數進行精確的運算。雙精度浮點型變量double可以處理16位有效數,
JAVA高精度演算法 BigInteger用法詳解 大數四則運算
在用C或者C++處理大數時感覺非常麻煩,但是在Java中有兩個類BigInteger和BigDecimal分別表示大整數類和大浮點數類,至於兩個類的物件能表示最大範圍不清楚,理論上能夠表示無線大的數,只要計算機記憶體足夠大。 這兩個類都在java.math.*包中,因此每次必須在開頭處引用該包。
Java高併發解決方案之非同步處理
(() -> { // 請求1 CompletableFuture<List<Integer>> completionStage1 = CompletableFuture.supplyAsync(() -> { //
1001 】Exponentiation (Java大數,高精度)
題幹: Problems involving the computation of exact values of very large magnitude and precision are common. For example, the computation of
整數大數模擬 高精度加法 高精度減法 高精度乘法 高精度除法 c/c++ java
描述 請計算a與b加減乘除的結果。a與b的值不超過100位,且為整數。 輸入 第一行,用例數T。 第二行,整數n,(1,2,3,4)分別表示加減乘除。 第三行,整數a與b。 輸出 輸出a與b計算後的值。(除法只需保留整數位)。 樣例輸入 4 1 1 2 2 10 8 3 4
java處理高精度的商業計算
在公司寫程式碼時,發現從mongodb中取出高精度資料時,會自動轉成科學計數法! 例如資料庫裡存的是`0.0002`,取出來時,變成了2.0E-4. 對於這種高精度的資料,一般要用到BigDecima
java中小數的處理:高精度運算用bigDecimal類,精度保留方法,即舍入方式的指定
一、 計算機的小數計算一定範圍內精確,超過範圍只能取近似值: 計算機儲存的浮點數受儲存bit位數影響,只能保證一定範圍內精準,超過bit範圍的只能取近似值。 java中各型別的精度範圍參見:http://blog.csdn.net/longshenlmj/