《笨辦法學python》筆記(完結)
阿新 • • 發佈:2019-01-31
列印:
%r
%r 與 %s 的區別就好比 repr() 函式處理物件與 str() 函式處理物件的差別。
%s => str(),比較智慧
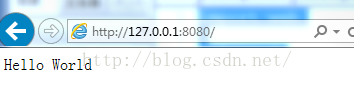
控制檯顯示:

開啟瀏覽器,http://127.0.0.1:8080?name=web

多個引數; 修改app.py
瀏覽器訪問:http://127.0.0.1:8080/?name=web&greet=python

建立HTML表單 目錄結構: gothonweb |----bin |----app.py |----templates |----index.html |----hello_form.html 新建一個hello_form.html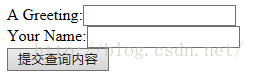
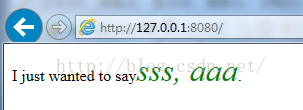
控制檯:
其實這裡還能看出HTTP的連線是短連線,每次都是客戶端的隨機埠重新發起一次連線 瀏覽器訪問/,發起一次GET請求,app.py中index.GET返回了hello_form.html,當填好表單以後,通過form,將POST請求發給了index.POST做處理,再返回響應給瀏覽器 建立佈局模板(類似其它的模板繼承) 目錄結構: gothonweb |----bin |----app.py |----templates |----index.html |----hello_form.html |----layout.html 修改index.html
修改app.py
%r => repr(),處理較為簡單和直接
結果: hello world (str) hello 'world' (repr) 2016-10-28 11:37:28.554000 time is 2016-10-28 11:37:28.554000 (str) datetime.datetime(2016, 10, 28, 11, 37, 28, 554000) time is datetime.datetime(2016, 10, 28, 11, 37, 28, 554000) (repr) %r, repr就是對string的一個格式化處理,即,返回的是一個格式化處理過的string(新物件) argv,可變引數:from datetime import datetime s = "world" print("hello %s (str)" %s) print("hello %r (repr)" %s) timeinfo = datetime.today() print(str(timeinfo)) print("time is %s (str)" %timeinfo) print(repr(timeinfo)) print("time is %r (repr)" %timeinfo)
終端輸入:python test1.py a b c 結果: ('The script is called:', 'test1.py') ('Your first variable is:', 'a') ('Your second variable is:', 'b') ('Your third variable is:', 'c') argv的第一個引數是檔名,所以接收一個引數都是argv[1] 讀寫檔案 open( )開啟檔案 close( )關閉兼儲存檔案 read( )讀取檔案內容 readline( )讀取一行內容 write( )寫入內容#argv,可變引數(argument variable) from sys import argv #引數解包(unpack),將引數放進對應的變數中 script, first, secind, third = argv print("The script is called:",script) print("Your first variable is:",first) print("Your second variable is:",secind) print("Your third variable is:",third)
列表#with,簡化了try…expect…finally,並且可以自動關閉檔案 with open("xx.txt", "r") as f: #read(),一次性將檔案獨進一個string中 print(f.read()) print(type(f.read())) with open("xx.txt", "r") as f: for line in f: print(line) #一行一行讀取問價內容
list_1 = ['a','b','b','c','d','e']
#pop(),刪除最後一個元素
list_1.pop()
print(list_1)
#count(),統計這個元素
print(list_1.count("b"))
#remove(),刪除指定元素
list_1.remove('b')
print(list_1)
#extend(),新增元素組或者當作兩個列表相加,新增在原列表最後
list_1.extend(['q','t'])
print(list_1)
#append(),在列表最後新增元素
list_1.append('w')
print(list_1)
</span><span style="font-size:24px;">#insert(),元素插入指定位置
list_1.insert(1,'s')
print(list_1)
#reverse(),反向列表元素
list_1.reverse()
print(list_1)
#sort(),排序列表元素,可以有三個引數sorted(data, cmp=None, key=None, reverse=False),reverse引數是升序降序
list_1.sort()
print(list_1)
結果:
['a', 'b', 'b', 'c', 'd']
2
['a', 'b', 'c', 'd']
['a', 'b', 'c', 'd', 'q', 't']
['a', 'b', 'c', 'd', 'q', 't', 'w']
['a', 's', 'b', 'c', 'd', 'q', 't', 'w']
['w', 't', 'q', 'd', 'c', 'b', 's', 'a']
['a', 'b', 'c', 'd', 'q', 's', 't', 'w']
web.py
目錄結構:
gothonweb
|----bin
|----app.py
第一個程式
編寫app.py:
import web
urls = (
'/','index',
)
app = web.application(urls, globals())
class index:
def GET(self):
greeting = "Hello World"
return greeting
if __name__=="__main__":
app.run()
執行app.py
開啟瀏覽器,http://127.0.0.1:8080/
控制檯顯示:
$def with(greeting)
<html>
<head>
<meta charset="UTF-8">
<title>Test</title>
</head>
<body>
$if greeting:
I just wanted to say<em style="color:green;font-size: 2em;">$greeting</em>.
$else:
<em>Hello</em>,World!
</body>
</html>
修改app.py
import web
urls = (
'/','index',
)
app = web.application(urls, globals())
render = web.template.render('templates/')
class index(object):
def GET(self):
greeting = "Hello World"
return render.index(greeting=greeting)
if __name__=="__main__":
app.run()
開啟瀏覽器,http://127.0.0.1:8080/
import web
urls = (
'/','index',
)
app = web.application(urls, globals())
render = web.template.render('templates/')
class index(object):
def GET(self):
form = web.input(name="Nobody")
greeting = "Hello %s"%form.name
return render.index(greeting=greeting)
if __name__=="__main__":
app.run()
開啟瀏覽器,http://127.0.0.1:8080/
開啟瀏覽器,http://127.0.0.1:8080?name=web
多個引數; 修改app.py
import web
urls = (
'/','index',
)
app = web.application(urls, globals())
render = web.template.render('templates/')
class index(object):
def GET(self):
form = web.input(name="Nobody", greet=None)
if form.greet:
greeting = "Hello %s, %s"%(form.name, form.greet)
return render.index(greeting=greeting)
else:
return "ERROR:GREET IS REQUIRED!"
if __name__=="__main__":
app.run()
瀏覽器訪問:http://127.0.0.1:8080/?name=web
瀏覽器訪問:http://127.0.0.1:8080/?name=web&greet=python
建立HTML表單 目錄結構: gothonweb |----bin |----app.py |----templates |----index.html |----hello_form.html 新建一個hello_form.html
<html>
<head>
<meta charset="UTF-8">
<title>Hello</title>
</head>
<body>
<form action="/" method="POST">
A Greeting:<input type="text" name="greet">
<br/>
Your Name:<input type="text" name="name">
<br/>
<input type="submit">
</form>
</body>
</html>
修改app.py
import web
urls = (
'/','index',
)
app = web.application(urls, globals())
render = web.template.render('templates/')
class index(object):
def GET(self):
return render.hello_form()
def POST(self):
form = web.input(name="Nobody", greet="Hello")
greeting = "%s, %s"%(form.name, form.greet)
return render.index(greeting=greeting)
if __name__=="__main__":
app.run()
瀏覽器訪問http://127.0.0.1:8080/
控制檯:
其實這裡還能看出HTTP的連線是短連線,每次都是客戶端的隨機埠重新發起一次連線 瀏覽器訪問/,發起一次GET請求,app.py中index.GET返回了hello_form.html,當填好表單以後,通過form,將POST請求發給了index.POST做處理,再返回響應給瀏覽器 建立佈局模板(類似其它的模板繼承) 目錄結構: gothonweb |----bin |----app.py |----templates |----index.html |----hello_form.html |----layout.html 修改index.html
def with(greeting)
$if greeting:
I just wanted to say<em style="color:green;font-size: 2em;">$greeting</em>.
$else:
<em>Hello</em>,World!
修改hello_form.html
<form action="/" method="POST">
A Greeting:<input type="text" name="greet">
<br/>
Your Name:<input type="text" name="name">
<br/>
<input type="submit">
</form>
新建layout.html
$def with(content)
<html>
<head>
<meta charset="UTF-8">
<title></title>
</head>
<body>
$:content
</body>
</html>
修改app.py
import web
urls = (
'/','index',
)
app = web.application(urls, globals())
render = web.template.render('templates/', base="layout")
class index(object):
def GET(self):
return render.hello_form()
def POST(self):
form = web.input(name="Nobody", greet="Hello")
greeting = "%s, %s"%(form.name, form.greet)
return render.index(greeting=greeting)
if __name__=="__main__":
app.run()