Vue檢視渲染原理解析,從構建VNode到生成真實節點樹
阿新 • • 發佈:2020-08-09
## 前言
在 `Vue` 核心中除了響應式原理外,檢視渲染也是重中之重。我們都知道每次更新資料,都會走檢視渲染的邏輯,而這當中牽扯的邏輯也是十分繁瑣。
本文主要解析的是初始化檢視渲染流程,你將會了解到從掛載元件開始,`Vue` 是如何構建 `VNode`,又是如何將 `VNode` 轉為真實節點並掛載到頁面。
## 掛載元件($mount)
`Vue` 是一個建構函式,通過 `new` 關鍵字進行例項化。
```js
// src/core/instance/index.js
function Vue (options) {
if (process.env.NODE_ENV !== 'production' &&
!(this instanceof Vue)
) {
warn('Vue is a constructor and should be called with the `new` keyword')
}
this._init(options)
}
```
在例項化時,會呼叫 `_init` 進行初始化。
```js
// src/core/instance/init.js
Vue.prototype._init = function (options?: Object) {
const vm: Component = this
// ...
if (vm.$options.el) {
vm.$mount(vm.$options.el)
}
}
```
`_init` 內會呼叫 `$mount` 來掛載元件,而 `$mount` 方法實際呼叫的是 `mountComponent`。
```js
// src/core/instance/lifecycle.js
export function mountComponent (
vm: Component,
el: ?Element,
hydrating?: boolean
): Component {
vm.$el = el
// ...
callHook(vm, 'beforeMount')
let updateComponent
/* istanbul ignore if */
if (process.env.NODE_ENV !== 'production' && config.performance && mark) {
// ...
} else {
updateComponent = () => {
vm._update(vm._render(), hydrating) // 渲染頁面函式
}
}
// we set this to vm._watcher inside the watcher's constructor
// since the watcher's initial patch may call $forceUpdate (e.g. inside child
// component's mounted hook), which relies on vm._watcher being already defined
new Watcher(vm, updateComponent, noop, { // 渲染watcher
before () {
if (vm._isMounted && !vm._isDestroyed) {
callHook(vm, 'beforeUpdate')
}
}
}, true /* isRenderWatcher */)
hydrating = false
// manually mounted instance, call mounted on self
// mounted is called for render-created child components in its inserted hook
if (vm.$vnode == null) {
vm._isMounted = true
callHook(vm, 'mounted')
}
return vm
}
```
`mountComponent` 除了呼叫一些生命週期的鉤子函式外,最主要是 `updateComponent`,它就是負責渲染檢視的核心方法,其只有一行核心程式碼:
```js
vm._update(vm._render(), hydrating)
```
`vm._render` 建立並返回 `VNode`,`vm._update` 接受 `VNode` 將其轉為真實節點。
`updateComponent` 會被傳入 `渲染Watcher`,每當資料變化觸發 `Watcher` 更新就會執行該函式,重新渲染檢視。`updateComponent` 在傳入 `渲染Watcher` 後會被執行一次進行初始化頁面渲染。
所以我們著重分析的是 `vm._render` 和 `vm._update` 兩個方法,這也是本文主要了解的原理——`Vue` 檢視渲染流程。
## 構建VNode(_render)
首先是 `_render` 方法,它用來構建元件的 `VNode`。
```js
// src/core/instance/render.js
Vue.prototype._render = function () {
const { render, _parentVnode } = vm.$options
vnode = render.call(vm._renderProxy, vm.$createElement)
return vnode
}
```
`_render` 內部會執行 `render` 方法並返回構建好的 `VNode`。`render` 一般是模板編譯後生成的方法,也有可能是使用者自定義。
```js
// src/core/instance/render.js
export function initRender (vm) {
vm._c = (a, b, c, d) => createElement(vm, a, b, c, d, false)
vm.$createElement = (a, b, c, d) => createElement(vm, a, b, c, d, true)
}
```
`initRender` 在初始化就會執行為例項上繫結兩個方法,分別是 `vm._c` 和 `vm.$createElement`。它們兩者都是呼叫 `createElement` 方法,它是建立 `VNode` 的核心方法,最後一個引數用於區別是否為使用者自定義。
`vm._c` 應用場景是在編譯生成的 `render` 函式中呼叫,`vm.$createElement` 則用於使用者自定義 `render` 函式的場景。就像上面 `render` 在呼叫時會傳入引數 `vm.$createElement`,我們在自定義 `render` 函式接收到的引數就是它。
### createElement
```js
// src/core/vdom/create-elemenet.js
export function createElement (
context: Component,
tag: any,
data: any,
children: any,
normalizationType: any,
alwaysNormalize: boolean
): VNode | Array {
if (Array.isArray(data) || isPrimitive(data)) {
normalizationType = children
children = data
data = undefined
}
if (isTrue(alwaysNormalize)) {
normalizationType = ALWAYS_NORMALIZE
}
return _createElement(context, tag, data, children, normalizationType)
}
```
`createElement` 方法實際上是對 `_createElement` 方法的封裝,它允許傳入的引數更加靈活。
```js
export function _createElement (
context: Component,
tag?: string | Class | Function | Object,
data?: VNodeData,
children?: any,
normalizationType?: number
): VNode | Array {
if (isDef(data) && isDef(data.is)) {
tag = data.is
}
if (!tag) {
// in case of component :is set to falsy value
return createEmptyVNode()
}
// support single function children as default scoped slot
if (Array.isArray(children) &&
typeof children[0] === 'function'
) {
data = data || {}
data.scopedSlots = { default: children[0] }
children.length = 0
}
if (normalizationType === ALWAYS_NORMALIZE) {
children = normalizeChildren(children)
} else if (normalizationType === SIMPLE_NORMALIZE) {
children = simpleNormalizeChildren(children)
}
let vnode, ns
if (typeof tag === 'string') {
let Ctor
ns = (context.$vnode && context.$vnode.ns) || config.getTagNamespace(tag)
if (config.isReservedTag(tag)) {
// platform built-in elements
vnode = new VNode(
config.parsePlatformTagName(tag), data, children,
undefined, undefined, context
)
} else if (isDef(Ctor = resolveAsset(context.$options, 'components', tag))) {
// component
vnode = createComponent(Ctor, data, context, children, tag)
} else {
// unknown or unlisted namespaced elements
// check at runtime because it may get assigned a namespace when its
// parent normalizes children
vnode = new VNode(
tag, data, children,
undefined, undefined, context
)
}
} else {
// direct component options / constructor
vnode = createComponent(tag, data, context, children)
}
if (Array.isArray(vnode)) {
return vnode
} else if (isDef(vnode)) {
if (isDef(ns)) applyNS(vnode, ns)
if (isDef(data)) registerDeepBindings(data)
return vnode
} else {
return createEmptyVNode()
}
}
```
`_createElement` 引數中會接收 `children`,它表示當前 `VNode` 的子節點,因為它是任意型別的,所以接下來需要將其規範為標準的 `VNode` 陣列;
```js
// 這裡規範化 children
if (normalizationType === ALWAYS_NORMALIZE) {
children = normalizeChildren(children)
} else if (normalizationType === SIMPLE_NORMALIZE) {
children = simpleNormalizeChildren(children)
}
```
`simpleNormalizeChildren` 和 `normalizeChildren` 均用於規範化 `children`。由 `normalizationType` 判斷 `render` 函式是編譯生成的還是使用者自定義的。
```js
// 1. When the children contains components - because a functional component
// may return an Array instead of a single root. In this case, just a simple
// normalization is needed - if any child is an Array, we flatten the whole
// thing with Array.prototype.concat. It is guaranteed to be only 1-level deep
// because functional components already normalize their own children.
export function simpleNormalizeChildren (children: any) {
for (let i = 0; i < children.length; i++) {
if (Array.isArray(children[i])) {
return Array.prototype.concat.apply([], children)
}
}
return children
}
// 2. When the children contains constructs that always generated nested Arrays,
// e.g. , , v-for, or when the children is provided by user
// with hand-written render functions / JSX. In such cases a full normalization
// is needed to cater to all possible types of children values.
export function normalizeChildren (children: any): ?Array {
return isPrimitive(children)
? [createTextVNode(children)]
: Array.isArray(children)
? normalizeArrayChildren(children)
: undefined
}
```
`simpleNormalizeChildren` 方法呼叫場景是 render 函式當函式是編譯生成的。`normalizeChildren` 方法的呼叫場景主要是 render 函式是使用者手寫的。
經過對 `children` 的規範化,`children` 變成了一個型別為 `VNode` 的陣列。之後就是建立 `VNode` 的邏輯。
```js
// src/core/vdom/patch.js
let vnode, ns
if (typeof tag === 'string') {
let Ctor
ns = (context.$vnode && context.$vnode.ns) || config.getTagNamespace(tag)
if (config.isReservedTag(tag)) {
// platform built-in elements
vnode = new VNode(
config.parsePlatformTagName(tag), data, children,
undefined, undefined, context
)
} else if (isDef(Ctor = resolveAsset(context.$options, 'components', tag))) {
// component
vnode = createComponent(Ctor, data, context, children, tag)
} else {
// unknown or unlisted namespaced elements
// check at runtime because it may get assigned a namespace when its
// parent normalizes children
vnode = new VNode(
tag, data, children,
undefined, undefined, context
)
}
} else {
// direct component options / constructor
vnode = createComponent(tag, data, context, children)
}
```
如果 `tag` 是 `string` 型別,則接著判斷如果是內建的一些節點,建立一個普通 `VNode`;如果是為已註冊的元件名,則通過 `createComponent` 建立一個元件型別的 `VNode`;否則建立一個未知的標籤的 `VNode`。
如果 `tag` 不是 `string` 型別,那就是 `Component` 型別, 則直接呼叫 `createComponent` 建立一個元件型別的 `VNode` 節點。
最後 `_createElement` 會返回一個 `VNode`,也就是呼叫 `vm._render` 時建立得到的`VNode`。之後 `VNode` 會傳遞給 `vm._update` 函式,用於生成真實dom。
## 生成真實dom(_update)
```js
// src/core/instance/lifecycle.js
Vue.prototype._update = function (vnode: VNode, hydrating?: boolean) {
const vm: Component = this
const prevEl = vm.$el
const prevVnode = vm._vnode
const prevActiveInstance = activeInstance
activeInstance = vm
vm._vnode = vnode
// Vue.prototype.__patch__ is injected in entry points
// based on the rendering backend used.
if (!prevVnode) {
// initial render
vm.$el = vm.__patch__(vm.$el, vnode, hydrating, false /* removeOnly */)
} else {
// updates
vm.$el = vm.__patch__(prevVnode, vnode)
}
activeInstance = prevActiveInstance
// update __vue__ reference
if (prevEl) {
prevEl.__vue__ = null
}
if (vm.$el) {
vm.$el.__vue__ = vm
}
// if parent is an HOC, update its $el as well
if (vm.$vnode && vm.$parent && vm.$vnode === vm.$parent._vnode) {
vm.$parent.$el = vm.$el
}
// updated hook is called by the scheduler to ensure that children are
// updated in a parent's updated hook.
}
```
`_update` 裡最核心的方法就是 `vm.__patch__` 方法,不同平臺的 `__patch__` 方法的定義會稍有不同,在 web 平臺中它是這樣定義的:
```js
// src/platforms/web/runtime/index.js
import { patch } from './patch'
// install platform patch function
Vue.prototype.__patch__ = inBrowser ? patch : noop
```
可以看到 `__patch__` 實際呼叫的是 `patch` 方法。
```js
// src/platforms/web/runtime/patch.js
import * as nodeOps from 'web/runtime/node-ops'
import { createPatchFunction } from 'core/vdom/patch'
import baseModules from 'core/vdom/modules/index'
import platformModules from 'web/runtime/modules/index'
// the directive module should be applied last, after all
// built-in modules have been applied.
const modules = platformModules.concat(baseModules)
export const patch: Function = createPatchFunction({ nodeOps, modules })
```
而 `patch` 方法是由 `createPatchFunction` 方法建立返回出來的函式。
```js
// src/core/vdom/patch.js
const hooks = ['create', 'activate', 'update', 'remove', 'destroy']
export function createPatchFunction (backend) {
let i, j
const cbs = {}
const { modules, nodeOps } = backend
for (i = 0; i < hooks.length; ++i) {
cbs[hooks[i]] = []
for (j = 0; j < modules.length; ++j) {
if (isDef(modules[j][hooks[i]])) {
cbs[hooks[i]].push(modules[j][hooks[i]])
}
}
}
// ...
return function patch (oldVnode, vnode, hydrating, removeOnly){}
}
```
這裡有兩個比較重要的物件 `nodeOps` 和 `modules`。`nodeOps` 是封裝的原生dom操作方法,在生成真實節點樹的過程中,dom相關操作都是呼叫 `nodeOps` 內的方法。
`modules` 是待執行的鉤子函式。在進入函式時,會將不同模組的鉤子函式分類放置到 `cbs` 中,其中包括自定義指令鉤子函式,ref 鉤子函式。在 `patch` 階段,會根據操作節點的行為取出對應型別進行呼叫。
### patch
```js
// initial render
vm.$el = vm.__patch__(vm.$el, vnode, hydrating, false /* removeOnly */)
```
在首次渲染時,`vm.$el` 對應的是根節點 dom 物件,也就是我們熟知的 id 為 app 的 div。它作為 `oldVNode` 引數傳入 `patch`:
```js
return function patch (oldVnode, vnode, hydrating, removeOnly) {
if (isUndef(vnode)) {
if (isDef(oldVnode)) invokeDestroyHook(oldVnode)
return
}
let isInitialPatch = false
const insertedVnodeQueue = []
if (isUndef(oldVnode)) {
// empty mount (likely as component), create new root element
isInitialPatch = true
createElm(vnode, insertedVnodeQueue)
} else {
const isRealElement = isDef(oldVnode.nodeType)
if (!isRealElement && sameVnode(oldVnode, vnode)) {
// patch existing root node
patchVnode(oldVnode, vnode, insertedVnodeQueue, null, null, removeOnly)
} else {
if (isRealElement) {
// mounting to a real element
// check if this is server-rendered content and if we can perform
// a successful hydration.
if (oldVnode.nodeType === 1 && oldVnode.hasAttribute(SSR_ATTR)) {
oldVnode.removeAttribute(SSR_ATTR)
hydrating = true
}
if (isTrue(hydrating)) {
if (hydrate(oldVnode, vnode, insertedVnodeQueue)) {
invokeInsertHook(vnode, insertedVnodeQueue, true)
return oldVnode
} else if (process.env.NODE_ENV !== 'production') {
warn(
'The client-side rendered virtual DOM tree is not matching ' +
'server-rendered content. This is likely caused by incorrect ' +
'HTML markup, for example nesting block-level elements inside ' +
'
, or missing
. Bailing hydration and performing ' + 'full client-side render.' ) } } // either not server-rendered, or hydration failed. // create an empty node and replace it oldVnode = emptyNodeAt(oldVnode) } // replacing existing element const oldElm = oldVnode.elm const parentElm = nodeOps.parentNode(oldElm) // create new node createElm( vnode, insertedVnodeQueue, // extremely rare edge case: do not insert if old element is in a // leaving transition. Only happens when combining transition + // keep-alive + HOCs. (#4590) oldElm._leaveCb ? null : parentElm, nodeOps.nextSibling(oldElm) ) // update parent placeholder node element, recursively if (isDef(vnode.parent)) { let ancestor = vnode.parent const patchable = isPatchable(vnode) while (ancestor) { for (let i = 0; i < cbs.destroy.length; ++i) { cbs.destroy[i](ancestor) } ancestor.elm = vnode.elm if (patchable) { for (let i = 0; i < cbs.create.length; ++i) { cbs.create[i](emptyNode, ancestor) } // #6513 // invoke insert hooks that may have been merged by create hooks. // e.g. for directives that uses the "inserted" hook. const insert = ancestor.data.hook.insert if (insert.merged) { // start at index 1 to avoid re-invoking component mounted hook for (let i = 1; i < insert.fns.length; i++) { insert.fns[i]() } } } else { registerRef(ancestor) } ancestor = ancestor.parent } } // destroy old node if (isDef(parentElm)) { removeVnodes([oldVnode], 0, 0) } else if (isDef(oldVnode.tag)) { invokeDestroyHook(oldVnode) } } } invokeInsertHook(vnode, insertedVnodeQueue, isInitialPatch) return vnode.elm } ``` 通過檢查屬性 `nodeType`(真實節點才有的屬性), 判斷 `oldVnode` 是否為真實節點。 ```js const isRealElement = isDef(oldVnode.nodeType) if (isRealElement) { // ... oldVnode = emptyNodeAt(oldVnode) } ``` 很明顯第一次的 `isRealElement` 是為 `true`,因此會呼叫 `emptyNodeAt` 將其轉為 `VNode`: ```js function emptyNodeAt (elm) { return new VNode(nodeOps.tagName(elm).toLowerCase(), {}, [], undefined, elm) } ``` 接著會呼叫 `createElm` 方法,它就是將 `VNode` 轉為真實dom 的核心方法: ```js function createElm ( vnode, insertedVnodeQueue, parentElm, refElm, nested, ownerArray, index ) { if (isDef(vnode.elm) && isDef(ownerArray)) { // This vnode was used in a previous render! // now it's used as a new node, overwriting its elm would cause // potential patch errors down the road when it's used as an insertion // reference node. Instead, we clone the node on-demand before creating // associated DOM element for it. vnode = ownerArray[index] = cloneVNode(vnode) } vnode.isRootInsert = !nested // for transition enter check if (createComponent(vnode, insertedVnodeQueue, parentElm, refElm)) { return } const data = vnode.data const children = vnode.children const tag = vnode.tag if (isDef(tag)) { vnode.elm = vnode.ns ? nodeOps.createElementNS(vnode.ns, tag) : nodeOps.createElement(tag, vnode) setScope(vnode) /* istanbul ignore if */ if (__WEEX__) { // ... } else { createChildren(vnode, children, insertedVnodeQueue) if (isDef(data)) { invokeCreateHooks(vnode, insertedVnodeQueue) } insert(parentElm, vnode.elm, refElm) } if (process.env.NODE_ENV !== 'production' && data && data.pre) { creatingElmInVPre-- } } else if (isTrue(vnode.isComment)) { vnode.elm = nodeOps.createComment(vnode.text) insert(parentElm, vnode.elm, refElm) } else { vnode.elm = nodeOps.createTextNode(vnode.text) insert(parentElm, vnode.elm, refElm) } } ``` 一開始會呼叫 `createComponent` 嘗試建立元件型別的節點,如果成功會返回 `true`。在建立過程中也會呼叫 `$mount` 進行元件範圍內的掛載,所以走的還是 `patch` 這套流程。 ```js if (createComponent(vnode, insertedVnodeQueue, parentElm, refElm)) { return } ``` 如果沒有完成建立,代表該 `VNode` 對應的是真實節點,往下繼續建立真實節點的邏輯。 ```js vnode.elm = vnode.ns ? nodeOps.createElementNS(vnode.ns, tag) : nodeOps.createElement(tag, vnode) ``` 根據 `tag` 建立對應型別真實節點,賦值給 `vnode.elm`,它作為父節點容器,建立的子節點會被放到裡面。 然後呼叫 `createChildren` 建立子節點: ```js function createChildren (vnode, children, insertedVnodeQueue) { if (Array.isArray(children)) { if (process.env.NODE_ENV !== 'production') { checkDuplicateKeys(children) } for (let i = 0; i < children.length; ++i) { createElm(children[i], insertedVnodeQueue, vnode.elm, null, true, children, i) } } else if (isPrimitive(vnode.text)) { nodeOps.appendChild(vnode.elm, nodeOps.createTextNode(String(vnode.text))) } } ``` 內部進行遍歷子節點陣列,再次呼叫 `createElm` 建立節點,而上面建立的 `vnode.elm` 作為父節點傳入。如此迴圈,直到沒有子節點,就會建立文字節點插入到 `vnode.elm` 中。 執行完成後出來,會呼叫 `invokeCreateHooks`,它負責執行 dom 操作時的 `create` 鉤子函式,同時將 `VNode` 加入到 `insertedVnodeQueue` 中: ```js function invokeCreateHooks (vnode, insertedVnodeQueue) { for (let i = 0; i < cbs.create.length; ++i) { cbs.create[i](emptyNode, vnode) } i = vnode.data.hook // Reuse variable if (isDef(i)) { if (isDef(i.create)) i.create(emptyNode, vnode) if (isDef(i.insert)) insertedVnodeQueue.push(vnode) } } ``` 最後一步就是呼叫 `insert` 方法將節點插入到父節點: ```js function insert (parent, elm, ref) { if (isDef(parent)) { if (isDef(ref)) { if (nodeOps.parentNode(ref) === parent) { nodeOps.insertBefore(parent, elm, ref) } } else { nodeOps.appendChild(parent, elm) } } } ``` 可以看到 `Vue` 是通過遞迴呼叫 `createElm` 來建立節點樹的。同時也說明最深的子節點會先呼叫 `insert` 插入節點。所以整個節點樹的插入順序是“先子後父”。插入節點方法就是原生dom的方法 `insertBefore` 和 `appendChild`。 ```js if (isDef(parentElm)) { removeVnodes([oldVnode], 0, 0) } ``` `createElm` 流程走完後,構建完成的節點樹已經插入到頁面上了。其實 `Vue` 在初始化渲染頁面時,並不是把原來的根節點 `app` 給真正替換掉,而是在其後面插入一個新的節點,接著再把舊節點給移除掉。 所以在 `createElm` 之後會呼叫 `removeVnodes` 來移除舊節點,它裡面同樣是呼叫的原生dom方法 `removeChild`。 ```js invokeInsertHook(vnode, insertedVnodeQueue, isInitialPatch) ``` ```js function invokeInsertHook (vnode, queue, initial) { // delay insert hooks for component root nodes, invoke them after the // element is really inserted if (isTrue(initial) && isDef(vnode.parent)) { vnode.parent.data.pendingInsert = queue } else { for (let i = 0; i < queue.length; ++i) { queue[i].data.hook.insert(queue[i]) } } } ``` 在 `patch` 的最後就是呼叫 `invokeInsertHook` 方法,觸發節點插入的鉤子函式。 至此整個頁面渲染的流程完畢~ ## 總結 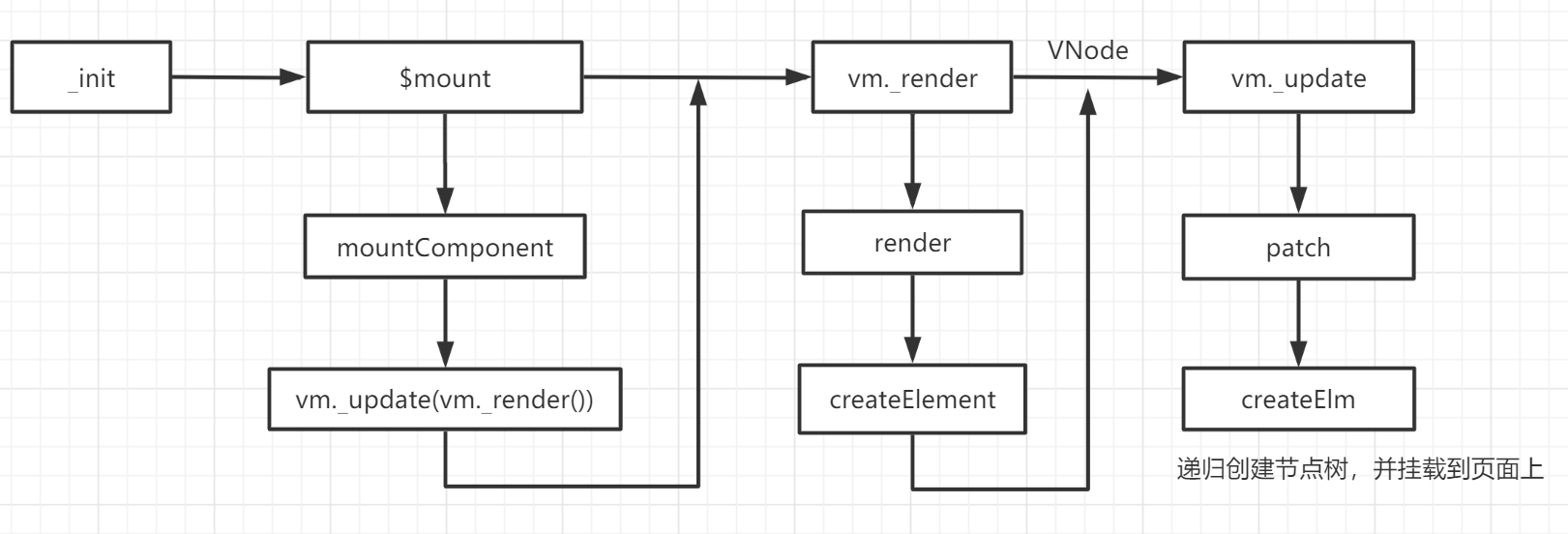 初始化呼叫 `$mount` 掛載元件。 `_render` 開始構建 `VNode`,核心方法為 `createElement`,一般會建立普通的 `VNode` ,遇到元件就建立元件型別的 `VNode`,否則就是未知標籤的 `VNode`,構建完成傳遞給 `_update`。 `patch` 階段根據 `VNode` 建立真實節點樹,核心方法為 `createElm`,首先遇到元件型別的 `VNode`,內部會執行 `$mount`,再走一遍相同的流程。普通節點型別則建立一個真實節點,如果它有子節點開始遞迴呼叫 `createElm`,使用 `insert` 插入子節點,直到沒有子節點就填充內容節點。最後遞迴完成後,同樣也是使用 `insert` 將整個節點樹插入到頁面中,再將舊的根節點移除。 **往期相關文章:** [手摸手帶你理解Vue響應式原理](https://juejin.im/post/6844904196790190088) [手摸手帶你理解Vue的Computed原理](https://juejin.im/post/6844904199596015624) [手摸手帶你理解Vue的Watch原理](https://juejin.im/post/6844904201752068109) [Vue你不得不知道的非同步更新機制和nextTick原理](https://juejin.im/post/6850418113667432462)