Root of AVL Tree
阿新 • • 發佈:2018-04-04
mage ted 技術分享 tinc pre math input span pos
Output Specification:
An AVL tree is a self-balancing binary search tree. In an AVL tree, the heights of the two child subtrees of any node differ by at most one; if at any time they differ by more than one, rebalancing is done to restore this property. Figures 1-4 illustrate the rotation rules.
Now given a sequence of insertions, you are supposed to tell the root of the resulting AVL tree.
Input Specification:
Each input file contains one test case. For each case, the first line contains a positive integer N (≤) which is the total number of keys to be inserted. Then N distinct integer keys are given in the next line. All the numbers in a line are separated by a space.
Output Specification:
For each test case, print the root of the resulting AVL tree in one line.
Sample Input 1:
5
88 70 61 96 120
Sample Output 1:
70
Sample Input 2:
7
88 70 61 96 120 90 65
Sample Output 2:
88
AC代碼
1 #include<stdio.h> 2 #include<stdlib.h> 3 4 typedef struct AVLNode *AVLTree; 5 struct AVLNode{6 int data; 7 AVLTree right; 8 AVLTree left; 9 //int height; 10 }; 11 int Max(int a,int b){ 12 return (a>b?a:b); 13 } 14 15 int GetTreeHeight(AVLTree T){ 16 int HL,HR; 17 if(T){ 18 HL = GetTreeHeight(T->left); 19 HR = GetTreeHeight(T->right); 20 return Max(HL,HR)+1; 21 } 22 else return 0; 23 } 24 AVLTree rotationLL(AVLTree T){ 25 AVLTree temp = T->left; 26 T->left = temp->right; 27 temp->right = T; 28 return temp; 29 } 30 AVLTree rotationRR(AVLTree T){ 31 32 AVLTree temp = T->right; 33 T->right = temp->left; 34 temp->left = T; 35 return temp; 36 } 37 AVLTree rotationLR(AVLTree T){ 38 T->left = rotationRR(T->left); 39 return rotationLL(T); 40 } 41 AVLTree rotationRL(AVLTree T){ 42 T->right = rotationLL(T->right); 43 return rotationRR(T); 44 } 45 46 AVLTree InsertAVLNode(AVLTree T,int X){ 47 if(!T){ 48 T = (AVLTree)malloc(sizeof(struct AVLNode)); 49 T->data = X; 50 T->right = T->left = NULL; 51 // T->hight = 0; 52 }else 53 if(X<T->data){ 54 T->left = InsertAVLNode(T->left,X); 55 if(GetTreeHeight(T->left) - GetTreeHeight(T->right) == 2){ 56 if(X < T->left->data){ 57 T = rotationLL(T); 58 }else 59 { 60 T = rotationLR(T); 61 } 62 } 63 }else if(X > T->data){ 64 T->right = InsertAVLNode(T->right,X); 65 if(GetTreeHeight(T->right) - GetTreeHeight(T->left)==2){ 66 if(X < T->right->data){ 67 T = rotationRL(T); 68 }else 69 { 70 T = rotationRR(T); 71 } 72 } 73 } 74 75 //T->height = Max(GetTreeHeight(T->left) , GetTreeHeight(T->right)) 76 return T; 77 } 78 int main(){ 79 80 int k; 81 int Data; 82 AVLTree T = NULL; 83 scanf("%d",&k); 84 for(int i = 0; i <k; i++){ 85 86 scanf("%d",&Data); 87 T = InsertAVLNode(T,Data); 88 } 89 printf("%d",T->data); 90 return 0; 91 }
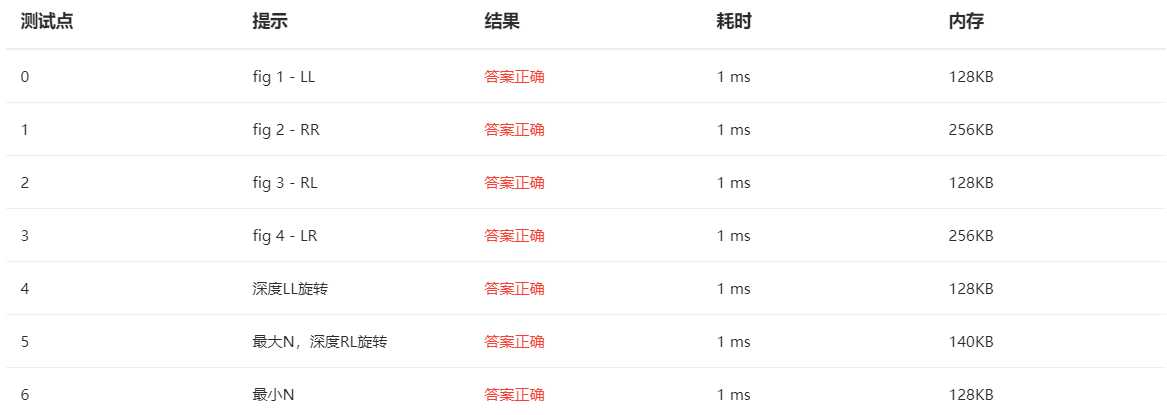
Root of AVL Tree