JavaScript刷LeetCode -- 915. Partition Array into Disjoint Intervals
阿新 • • 發佈:2018-12-23
一、題目
Given an array A, partition it into two (contiguous) subarrays left and right so that:
- Every element in left is less than or equal to every element in right.
- left and right are non-empty.
- left has the smallest possible size.
Return the length of left after such a partitioning. It is guaranteed that such a partitioning exists.
二、題目大意
找出一個分割點將陣列A分為左右兩部分,這兩部分必須滿足:
- 左邊部分的元素都小於右邊的元素
- 每部分至少有一個元素
求出這個分割點的最小下標值
三、解題思路
理解這道題目的意思之後,可以通過動態規劃記錄從左到右的最大值,從右到左的最小值。比較兩個陣列,找出第一次滿足上述條件的下標即為本題的答案。
四、程式碼實現
const partitionDisjoint = A => { const len = A.length // 左邊的最大值 應該小於右邊的最小值 const max = [] max[0] = Number.MIN_SAFE_INTEGER const min = [] min[0] = Number.MAX_SAFE_INTEGER for (let i = 0; i < len - 1; i++) { max[i + 1] = Math.max(max[i], A[i]) min[i + 1] = Math.min(min[i], A[len - 1 - i]) } let ans for (let i = 1; i < len; i++) { const start = max[i] const end = min[len - i] if (start <= end) { ans = i break } } return ans }
如果本文對您有幫助,歡迎關注我的微信公眾號【超愛敲程式碼】,為您推送更多內容,ε=ε=ε=┏(゜ロ゜;)┛。
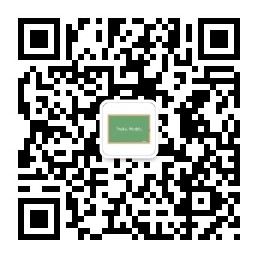