[LeetCode] Knight Probability in Chessboard 棋盤上騎士的可能性
On an N
xN
chessboard, a knight starts at the r
-th row and c
-th column and attempts to make exactly K
moves. The rows and columns are 0 indexed, so the top-left square is (0, 0)
, and the bottom-right square is (N-1, N-1)
.
A chess knight has 8 possible moves it can make, as illustrated below. Each move is two squares in a cardinal direction, then one square in an orthogonal direction.
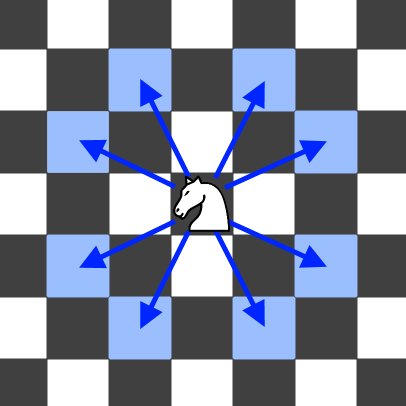
Each time the knight is to move, it chooses one of eight possible moves uniformly at random (even if the piece would go off the chessboard) and moves there.
The knight continues moving until it has made exactly K
moves or has moved off the chessboard. Return the probability that the knight remains on the board after it has stopped moving.
Example:
Input: 3, 2, 0, 0 Output: 0.0625 Explanation: There are two moves (to (1,2), (2,1)) that will keep the knight on the board. From each of those positions, there are also two moves that will keep the knight on the board. The total probability the knight stays on the board is 0.0625.
Note:
N
will be between 1 and 25.K
will be between 0 and 100.- The knight always initially starts on the board.
這道題給了我們一個大小為NxN國際象棋棋盤,上面有個騎士,相當於我們中國象棋中的馬,能走‘日’字,給了我們一個起始位置,然後說允許我們走K步,問走完K步之後還能留在棋盤上的概率是多少。那麼要求概率,我們必須要先分別求出分子和分母,其中分子是走完K步還在棋盤上的走法,分母是沒有限制條件的總共的走法。那麼分母最好算,每步走有8種跳法,那麼K步就是8的K次方種了。關鍵是要求出分子,博主開始向的方法是以給定位置為起始點,然後進行BFS,每步遍歷8種情況,遇到在棋盤上的就計數器加1,結果TLE了。上論壇看大家的解法,結果發現都是換了一個角度來解決問題的,並不很關心騎士的起始位置,而是把棋盤上所有位置上經過K步還留在棋盤上的走法總和都算出來,那麼最後直接返回需要的值即可。跟之前那道Out of Boundary Paths沒啥本質上的區別,又是換了一個馬甲就不會了系列。還是要用DP來做,我們可以用三維DP陣列,也可以用二維DP陣列來做,這裡為了省空間,我們就用二維DP陣列來做,其中dp[i][j]表示在棋盤(i, j)位置上走完當前步驟還留在棋盤上的走法總和,初始化為1,我們其實將步驟這個維度當成了時間維度在不停更新。好,下面我們先寫出8種‘日’字走法的位置的座標,就像之前遍歷迷宮上下左右四個方向座標一樣,這不過這次位置變了而已。然後我們一步一步來遍歷,每一步都需要完整遍歷一遍棋盤的每個位置,新建一個臨時陣列t,大小和dp陣列相同,但是初始化為0,然後對於遍歷到的棋盤上的每一個格子,我們都遍歷8中解法,如果新的位置不在棋盤上了,直接跳過,否則就加上上一步中的dp陣列中對應的值,遍歷完棋盤後,將dp陣列更新為這個臨時陣列t,參見程式碼如下:
解法一:
class Solution { public: double knightProbability(int N, int K, int r, int c) { if (K == 0) return 1; vector<vector<double>> dp(N, vector<double>(N, 1)); vector<vector<int>> dirs{{-1,-2},{-2,-1},{-2,1},{-1,2},{1,2},{2,1},{2,-1},{1,-2}}; for (int m = 0; m < K; ++m) { vector<vector<double>> t(N, vector<double>(N, 0)); for (int i = 0; i < N; ++i) { for (int j = 0; j < N; ++j) { for (auto dir : dirs) { int x = i + dir[0], y = j + dir[1]; if (x < 0 || x >= N || y < 0 || y >= N) continue; t[i][j] += dp[x][y]; } } } dp = t; } return dp[r][c] / pow(8, K); } };
我們也可以使用有memo陣列優化的遞迴來做,避免重複運算,從而能通過OJ。遞迴下的memo陣列其實就是迭代下的dp陣列,這裡我們用三維的陣列,初始化為0。在遞迴函式中,如果k為0了,說明已經走了k步,返回 1。如果memo[k][r][c]不為0,說明這種情況之前已經計算過,直接返回。然後遍歷8種走法,計算新的位置,如果不在棋盤上就跳過;然後更新memo[k][r][c],使其加上對新位置呼叫遞迴的返回值,注意此時帶入k-1和新的位置,退出迴圈後返回memo[k][r][c]即可,參見程式碼如下:
解法二:
class Solution { public: vector<vector<int>> dirs{{-1,-2},{-2,-1},{-2,1},{-1,2},{1,2},{2,1},{2,-1},{1,-2}}; double knightProbability(int N, int K, int r, int c) { vector<vector<vector<double>>> memo(K + 1, vector<vector<double>>(N, vector<double>(N, 0.0))); return helper(memo, N, K, r, c) / pow(8, K); } double helper(vector<vector<vector<double>>>& memo, int N, int k, int r, int c) { if (k == 0) return 1.0; if (memo[k][r][c] != 0.0) return memo[k][r][c]; for (auto dir : dirs) { int x = r + dir[0], y = c + dir[1]; if (x < 0 || x >= N || y < 0 || y >= N) continue; memo[k][r][c] += helper(memo, N, k - 1, x, y); } return memo[k][r][c]; } };
類似題目:
參考資料: