These React Fundamentals You Skip may be Killing You
If the component is a class-based component, the render
function is invoked to return the tree of elements.
class MyComponent extends React.Component { render() { //this function is invoked to return the tree of elements }}
If the component is a functional component, its return value yields the tree of elements.
function MyComponent() { // the return value yields the tree of elements return <div>
</div>}
Why is this important?
Consider a component, <MyComponent />
which takes in a prop
as shown below:
<MyComponent name='Ohans'/>
When this component is rendered, a tree of elements is returned.

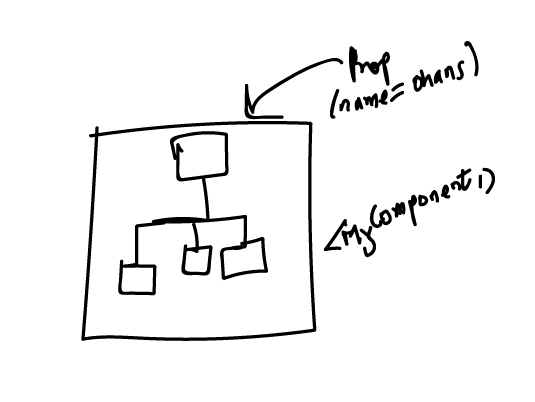
What happens when the value of name
changes?
<MyComponent name='Quincy'/>
Well, a new tree of elements is returned!

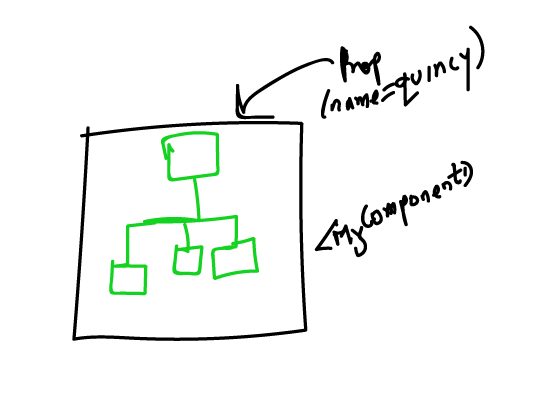
Okay.
Now, React has in its custody two different trees — the former and the current element tree.
At this point, React then compares both trees to find what exactly has changed.

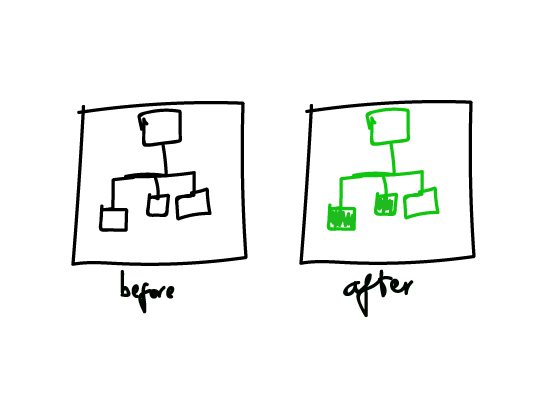
Most times the entire tree hasn’t changed. Just some updates here and there.
Upon comparing these two trees of elements, the actual DOM is then updated with the change in the new element tree.
Easy, huh?
This process of comparing two trees for changes is called “reconciliation”. It’s a technical process, but this conceptual overview is just great for understanding what goes on under the hood.
React Only Updates What’s Necessary. True?
When you get started with React, everyone’s told how awesome React is — particularly how it just updates the essential part of the DOM being updated.
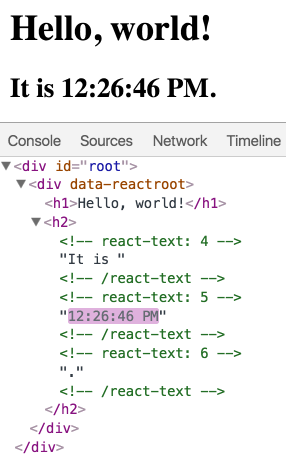
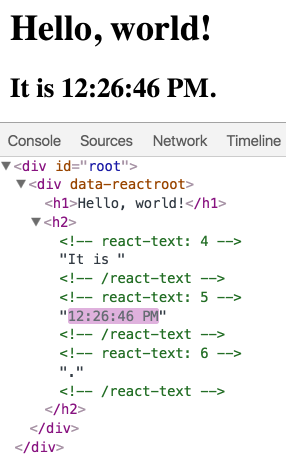
Is this completely true?
Yes it is.
However, before React gets to updating the DOM, remember that under the hood — it had first constructed the element tree for the various components and did the essential “diffing” before updating the DOM. In other words, it had compared the changes between the previous and current element trees.
The reason I re-iterate this is, if you’re new to React you may be blind to the performance ditches dug in your app because you think React just updates the DOM with what’s necessary.
While that is true, the performance concerns in most React apps begin with the process before the DOM is updated!
Wasted Renders vs. Visual Updates
No matter how small, rendering a component element tree takes some time (no matter how minute). The time for rendering gets larger as the component element tree increases.
The implication of this is that in your app you do not want React re-rending your component element tree if it is NOT important.
Let me show you a quick example.
Consider an app with a component structure as shown below:

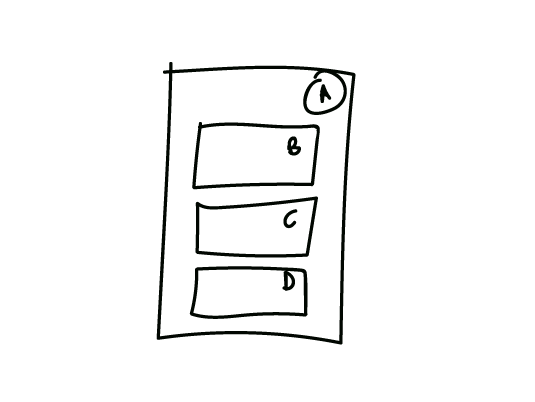
The overall container component, A
receives a certain prop. However, the sole reason for this is to pass the props down to component D
.

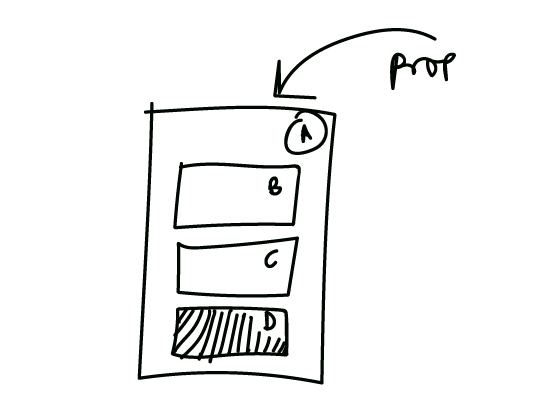
A
receives some props and passes them down to the child component D.Now, whenever the prop value in A
changes, the entire children elements of A
are re-rendered to compute a new element tree.