React Native and Forms Redeux: Part 1
React Native and Forms Redeux: Part 1
Yet (YET) another article on React and forms; with a React Native twist.

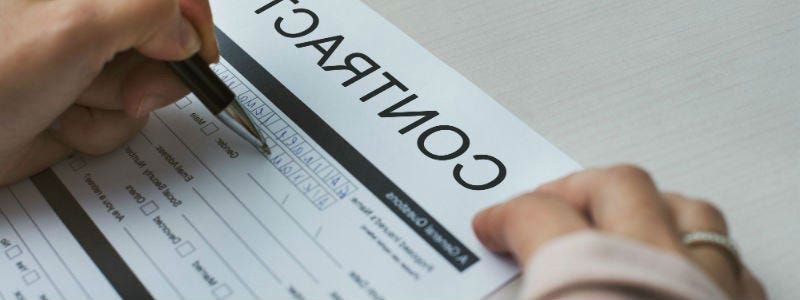
note: There is a reason the image is reversed and the for use of the word odd word redeux; read on.
The final application from this series is available for download.
Introduction
I just (today) finished writing a two part series,
Arggg! But, knowing that Redux Form is a bit clumsy and their documentation is overly complex, I decided to give Formik a try.
note: This series closely follows, if not plagiarizes, my other series.
Prerequisites
If you are looking for follow along, you will need:
- Node.js: JavaScript runtime; safest to use a LTS version (currently 8.12.0
- Yarn: Alternative Node.js package manager; had trouble with Expo with the default Node.js (npm) package manager
- Expo: React Native development platform; quickly becoming the defacto-standard platform for React Native development
Scaffolding Project
We start by creating an blank Expo project.
expo init
From within the new project folder, install the additional dependencies.
yarn add prop-typesyarn add formik
At this point, we can start the application.
expo start
Hello Form
We start by creating the absolute minimum form component, HelloForm, and add it to our application; the form component displays a button that, when clicked, outputs a message to the console.
App.js
And we create the form.
components / HelloForm / index.js
Observation:
- We provide a submit handler using the onSubmit handler to Formik
- In turn, the Formik (using the render prop pattern), provides a handleSubmit function to the render function (calling handleSubmit will in-turn call the submit handler provided to onSubmit)
- Unlike usage for the web, we don’t have any equivalent to the HTML form element; we simply add handleSubmit to the submit button. In the same vein, will not be using the Formik Form helper
Supporting Multiple Forms
As we want to retain all the form examples (for illustration purposes) in the application, we will be making copies of the previous examples as a starting point for the next example, e.g., we copy HelloForm to SimpleForm and rename the component.
In order to toggle the forms, we add buttons and state (old-school React).
App.js
Simple Form
We now modify the SimpleForm component to display two inputs (first and last name) and a button that, when clicked, outputs a message to the console (with the values of the first and last name).
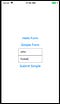
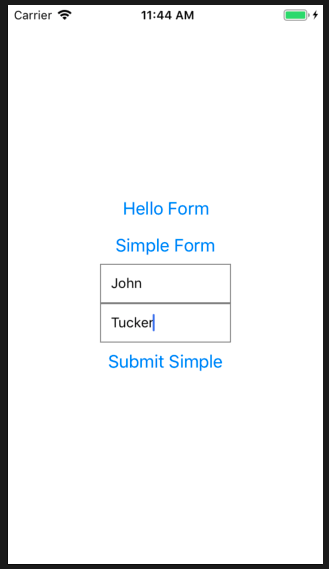
components / SimpleForm / index.js
Observations:
- In order to better see the TextInput component, we apply minimal styling through an external style sheet; good practice is to separate the styles (can be complex) from the view component
- Unlike usage for the web, for React Native, we need to supply the onChangeText handler using handleChange with a parameter, the name of the field. The return value of this function is another function (supplied to onChangeText)
components / SimpleForm / styles.js
Refactor (with Field)
Looking at the SimpleForm implementation, there was as fair amount of repetition between the two TextInputs. We can use the Formik Field component to address this.
As before, we start by copying the SimpleForm folder to a RefactorForm folder (renaming the component as appropriate) and adding to App.js.
We first create a general purpose component that provides a view, using a React Native TextInput component, for a Formik Field.
components / FKTextInput / index.js
Observation:
- The implementation is very similar to our previous example, except that the change handler property is onChange instead of handleChange. The reason for this is for the web implementation you can simply spread field onto an input directly (JSX spread attributes)
- As this is the view for our field, we centralize our styling here
components / FKTextInput / styles.js
We then use the FKTextInput with Formik Field to simplify our form; this will become more dramatic as we add additional functionality to our form.
components / RefactorForm / index.js
Observation:
- We no longer use the styles.js file in the form
Next Steps
In the next article, React Native and Forms Redeux: Part 2, we will introduce form validation and submission.