React For Beginners (Part 2)
You may have seen this funny syntax which looks like HTML code inside of your React application. This is JSX.
JSX is a syntax extension for JavaScript that looks like a combination between HTML and JavaScript. We use JSX elements to produce React elements.
Why Do We Use JSX?
In traditional applications, we separate the view (HTML) from the logic (JavaScript) React is built upon the idea that the logic which renders components is related to the data within the application. Thus, React couples the markup and the logic together inside of components.
JSX isn’t mandatory for creating React applications, but it can be extremely useful.
JSX Expressions
JSX expressions are used to pass variables to elements and properties, evaluate boolean expressions, and more! We can use curly braces ({}) to create expressions in JSX.
Let’s take a look at an example.
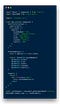
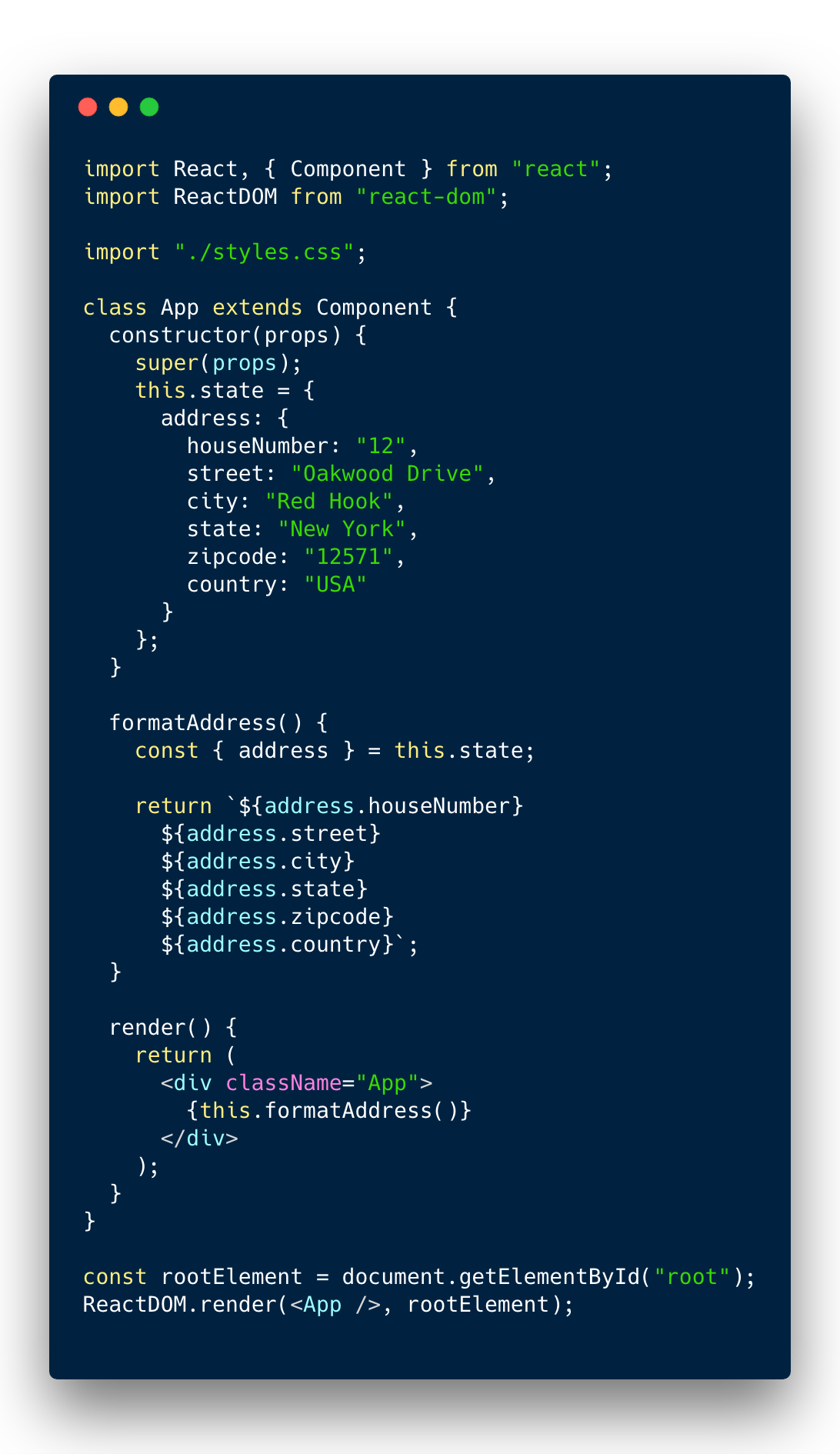
Note: React uses className
instead of class
, which is a preserved keyword in JavaScript. React DOM uses the camelCase naming convention.
In the App component, we create this.state = {...}
and add in an address
object. We manipulate this object into a concatenated string inside the formatAddress
function.
The syntax const { address } = this.state;
is called destructuring assignment. This makes it possible to place values from objects and arrays into variables.
If you’re unfamiliar with the syntax used to create the concatenated address string, it’s called a template literal. Template literals are extremely useful if you need to display the value inside of a variable within a string. You begin and end template literals with backticks (`) and any variables you want to evaluate must be placed inside a dollar sign with curly braces${...}
.

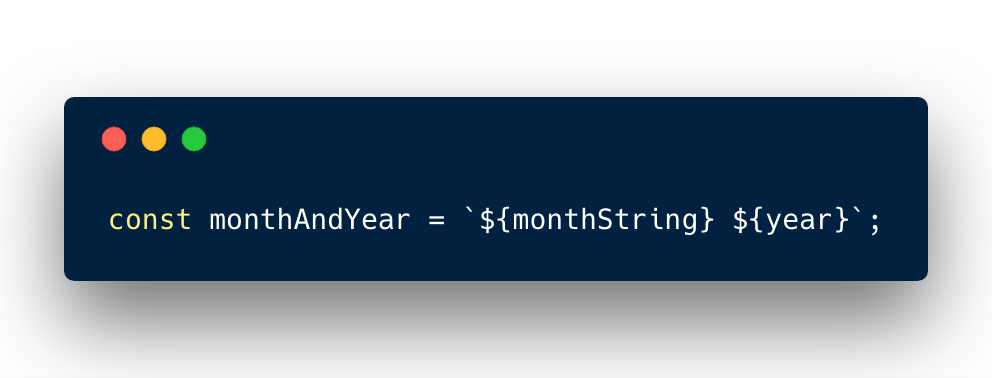
Finally, in the JSX code within the render function, we use JSX expressions to call formatAddress()
.
I would also like to note here that there are a couple of ways to create react components. The method above uses the class syntax. This is logically equivalent to the method below, the format is just a little different. (There are special use cases for each of these: i.e. stateful and stateless components, but we won’t delve into that now).

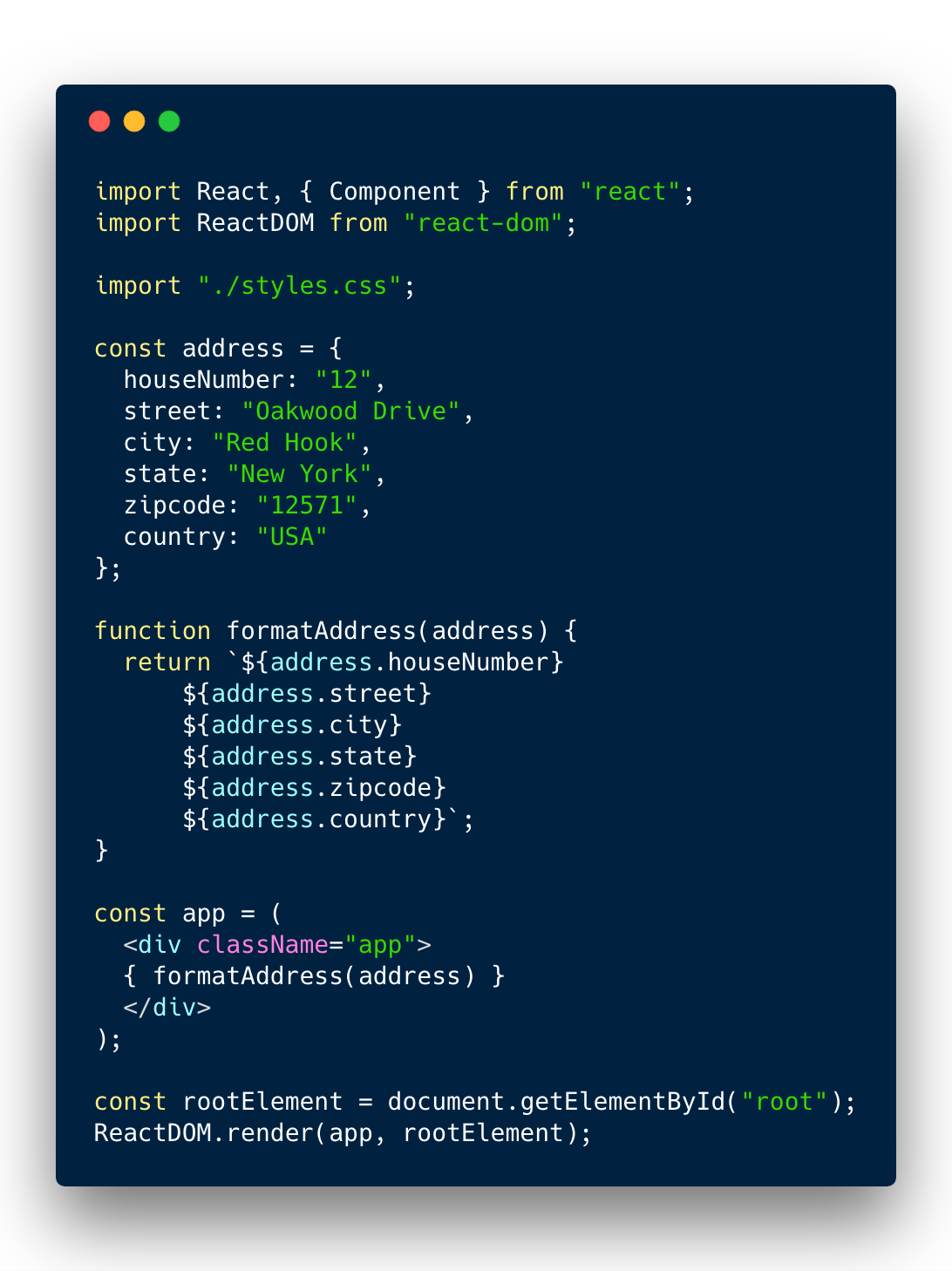
Attributes
You can specify string attributes, just like you do in HTML, with quotes or double quotes.

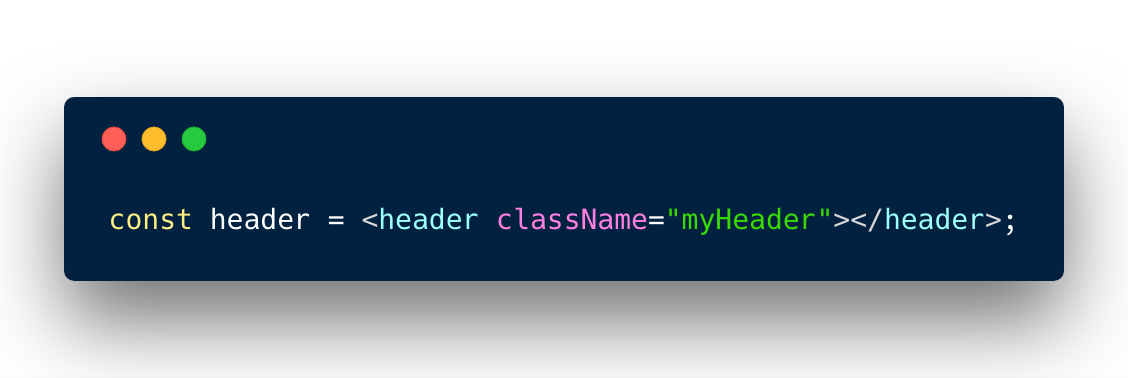
But you can also use the curly braces to evaluate a JavaScript expression. You don’t need to wrap the curly braces in quotes; you use either quotes (strings) or curly braces (expressions), but not both.

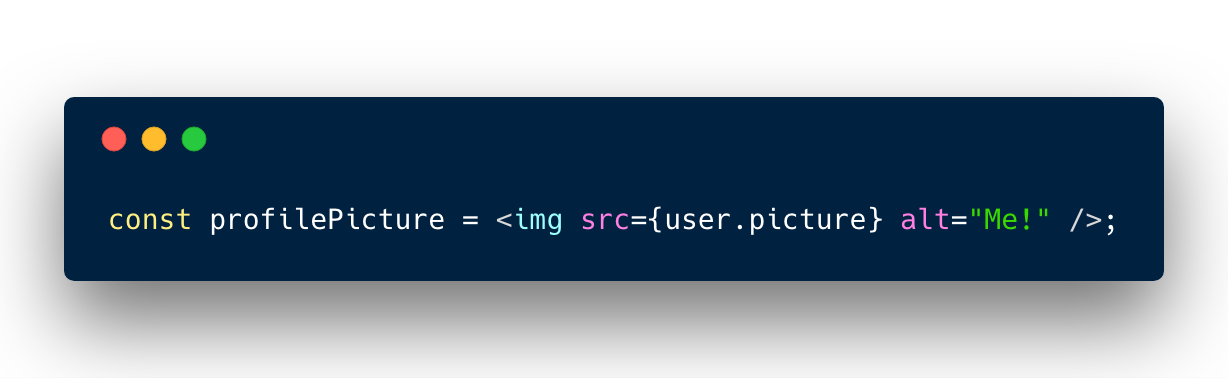
Conditional Classes
Setting conditional classes based on a variable is extremely useful. Conditional classes allow you to say: if this condition is true, add these classes to the component, otherwise add these classes.
You can use it to show and hide dialogs or modals when it’s visibility is toggled, for example. Here is the basic syntax.

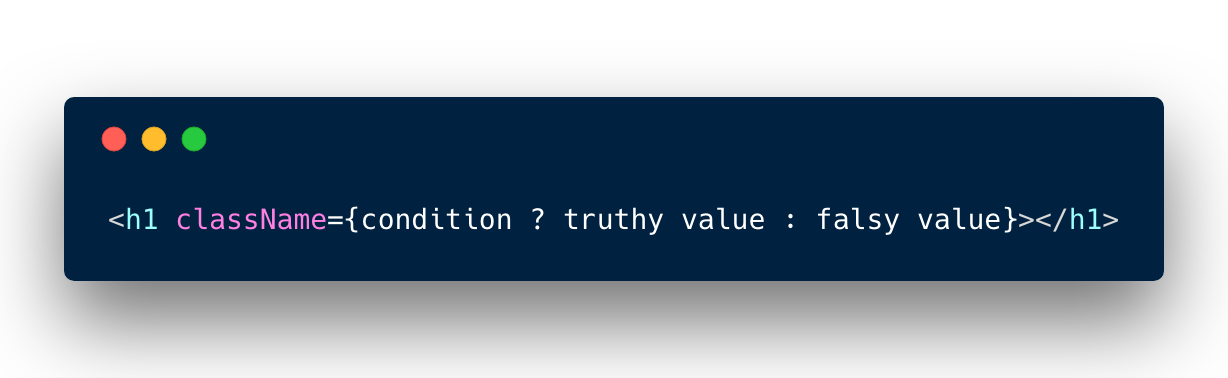
Let’s take a quick example. Let’s say we want to create a calendar application which shows the current month and year at the top of the application. We can use JSX expressions and conditional classes to show the correct data, and style it accordingly.
If the current month is later in the year than July, we’ll style the text with the color blue, otherwise we’ll style the text with the color red.

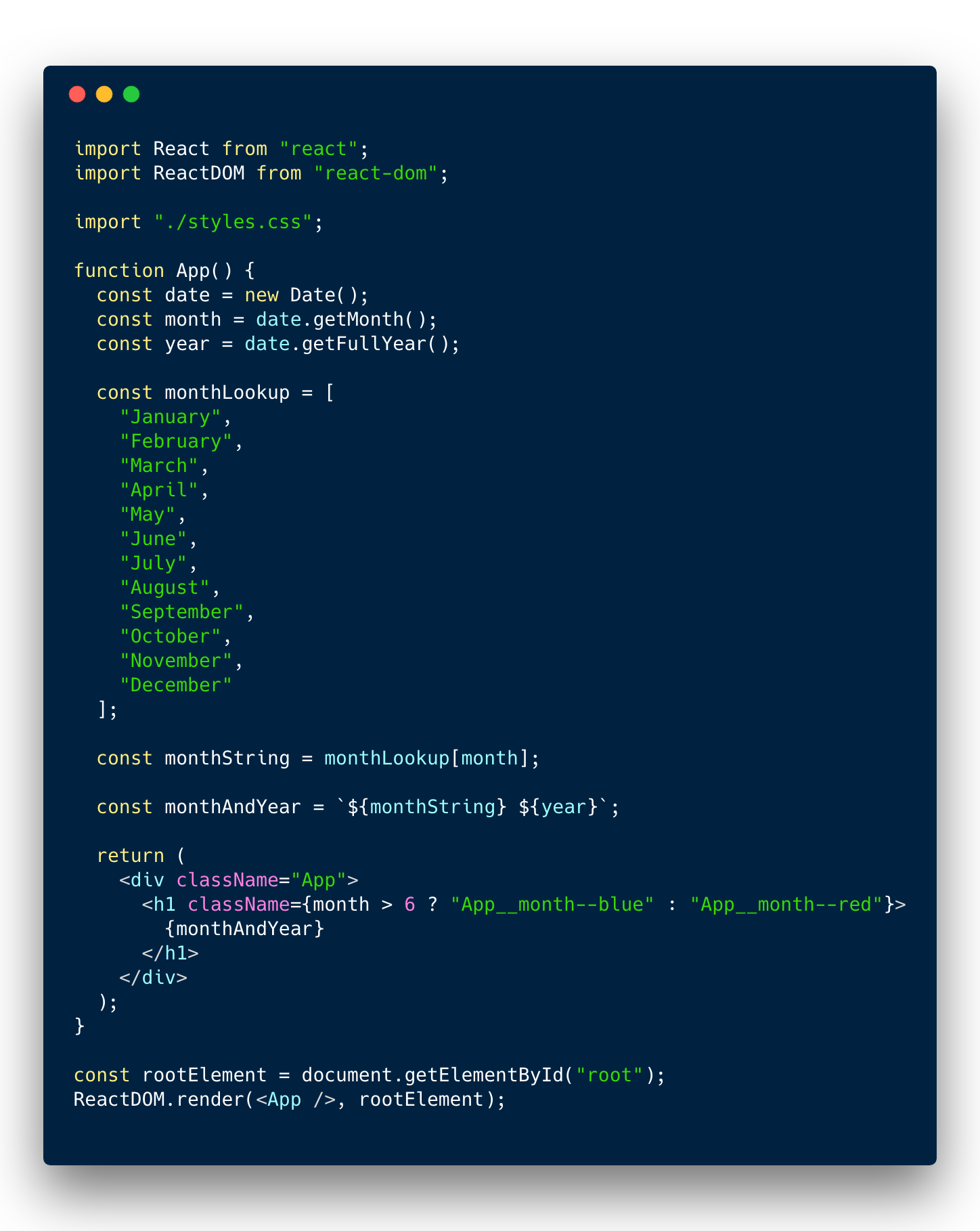
You can check out the full code here.
JSX Inside Functions
Since JSX in and of itself is an expression, we can use it within functions and if/else blocks.

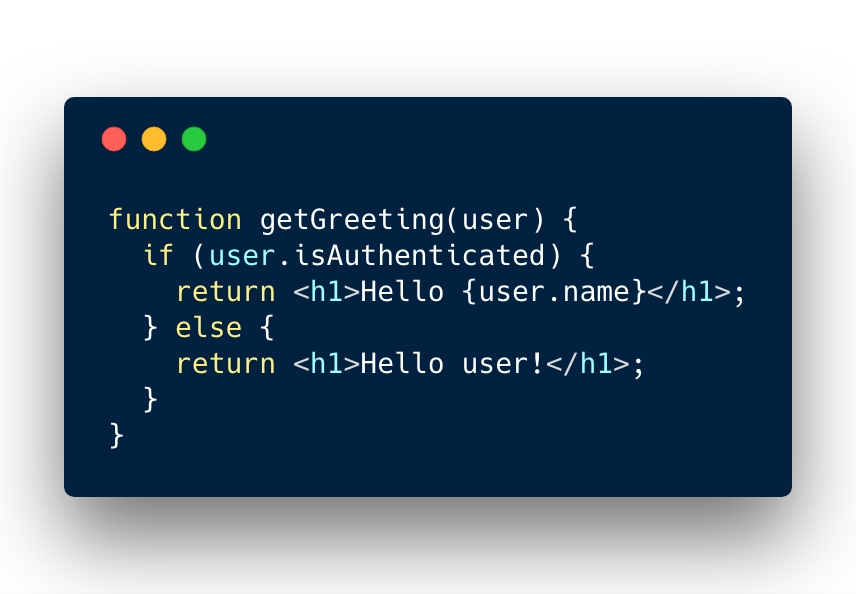
Above, we return the user’s name if they’re logged in and otherwise just return Hello User!
.
We can even re-factor this to make it a bit more succinct.