Blockchain Revolution: Part Four
Blockchain Revolution: Part Four
Building a simple blockchain application

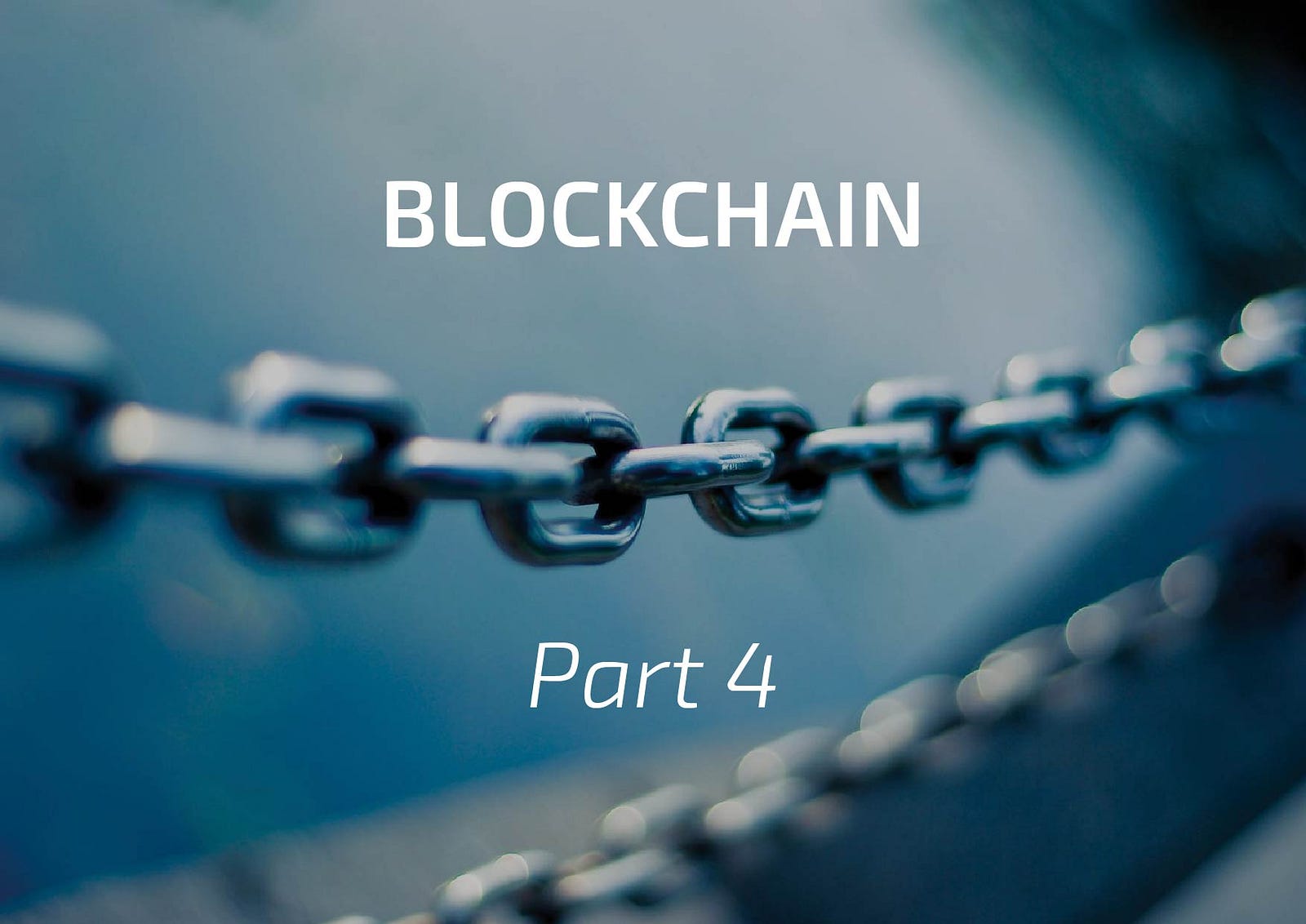
We are back with our fourth post of the series ‘Blockchain Revolution’ and if you have been following us, you would know that this post is about building a simple blockchain application. In case, you have missed the previous post here’s the
Now that we have a fair knowledge about what is a blockchain and how it works, let’s try implementing it. We will start by defining the block structure that has the following attributes:
- Index: This is the position of a block within the blockchain.
- Previous hash: All the blocks are linked together to form a chain called blockchain. It is a reference to the hash of previous block.
- Timestamp: Time when the block was generated.
- Data: This is the data that is stored on the block and it depends on the type of blockchain. This data can be medical records or transaction details of an individual.
- Current hash: This is the hash of the current block. Current hash is generated after the block is created.
The block structure mentioned above looks like this:
class Block {
constructor(data, previousHash, timestamp, index,
currentHash) {
this.index = index;
this.previousHash = previousHash;
this.timestamp = new Date().getTime();
this.data = data;
this.hash = currentHash;
}
}
Now that we have created a block, let’s calculate its hash. Hash is calculated by taking any input string and generating an output of fixed length. Hash is generated by taking all the contents of the block. Creating a hash can be achieved by importing a library ‘crypto-js’.
npm install crypto-js --save-dev
and then import the sha256 module of crypto-js, which converts the input string to hashed string.
const sha256 = require('crypto-js/sha256');
Create a method calculateHash() which takes contents of the block and creates a hash string of fixed length.
calculateHash() {
return sha256(this.index + this.previousHash +
this.timestamp +JSON.stringify(this.data)).toString();
}
Now add the above function to the constructor:
class Block {
constructor(data, previousHash, timestamp, index,
currentHash) {
this.index = index;
this.previousHash = previousHash;
this.timestamp = new Date().getTime();
this.data = data;
this.hash = this.calculateHash();
}
}
Create another class blockchain to develop methods related to operations performed on the blockchain.
Creating genesis block
Genesis block is the first block of the blockchain where there is no previous hash. Therefore, we will need to hard code the contents of the block.
class Blockchain {
createGenesisBlock() {
return new Block("Genesis Block", "89eb0ac031a63d", null,
0);
}
}
Creating a chain of blocks
Create a constructor inside blockchain class and add the above created genesis block to it. All the blocks created will be added to this chain. For now, we will store the blockchain in an array.
class Blockchain {
constructor() {
this.chain = [this.createGenesisBlock()];
}
}
Now let’s try fetching the latest block.
fetchLatestBlock() {
return this.chain[this.chain.length - 1];
}
Using this method you can fetch the latest block created in the blockchain. We will need this block to fetch the hash of the previous block.
Creating a block
Hash of the previous block is required to create a block. Data of the block will be provided by the user while we will create the rest of the contents of the block.
addNewBlock(newBlock) {
newBlock.index = this.fetchLatestBlock().index + 1;
newBlock.previousHash = this.fetchLatestBlock().hash;
newBlock.hash = newBlock.calculateHash();
this.chain.push(newBlock);
}
This method helps create and push the block to the blockchain.
Validating the chain
At any point of time we should be able to verify whether or not a block or chain of blocks are valid. For a block to be valid it must meet the following criteria:
Hash
of the current block should be valid. Current hash changes if the contents of the block are changed.previousHash
block must match the hash of the previous block.- Index of current block must be greater by a number than the index of previous block.
The function elaborated below verifies all these criteria and returns a Boolean value in response. If it returns true, then the chain is valid, if it returns false, then the chain is invalid.
isChainValid() {
for ( let i=1 ; i < this.chain.length ; i++) {
const currentBlock = this.chain[i];
const previousBlock = this.chain[i - 1];
if (currentBlock.hash !== currentBlock.calculateHash()) {
console.log("Hash is invalid.");
return false;
}
if (currentBlock.previousHash !== previousBlock.hash ) {
console.log("Hash of previous block is invalid.");
return false;
}
if (currentBlock.index !== previousBlock.index + 1){
console.log("Index is invalid.");
return false;
}
}
return true;
}
Now let’s test the blockchain we created. Create an instance of the blockchain and addnew blocks to the created blockchain.
let chainCoin = new Blockchain();
chainCoin.addNewBlock(new Block("{amount : 4}"));
chainCoin.addNewBlock(new Block("{amount : 10}"));
Here, we are just providing data for the block. All the other attributes including index, previous hash, timestamp and hash will be generated automatically.
So, this is how we create simple blockchain application. I hope this post helps you get started and transition smoothly into creating blockchains. For more information you can check the GitHub repository for the above code here. Stay tuned for the next post where we will walk you through how to implement PoW in application, and please do not forget to a leave a comment.