Android file類使用詳解-SDcard
阿新 • • 發佈:2019-01-03
Android file類使用詳解
一.Android file類 在開發Android應用時免不了會跟檔案打交道,本篇文章記錄總結自己常用到的檔案操作,資料的儲存有多種方式,比如資料庫儲存、SharedPreferences儲存、檔案儲存等;這裡我們將要介紹最簡單的檔案儲存方式;檔案儲存簡單的來說就是一般的JAVASE中的IO流,只是把他應用於Android手機中而已。
<uses-permission android:name="android.permission.MOUNT_UNMOUNT_FILESYSTEMS"/>
2.SDCard寫入資料許可權 <uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE"/>
程式碼如下:
File類語法 1.File(String pathname) File file = new File ("/mnt/sdcard/test.txt");
2.File(String dir, String subpath) File file = new File("/mnt/sdcard/temp", "test.txt");
File類常用方法
boolean exists() 測試檔案是否存在
boolean delete() 刪除此物件指定的檔案
boolean createNewFile() 建立新的空檔案
boolean isDirectory() 測試此File物件表示的檔案是否是目錄
boolean mkdir() 建立由該File物件表示的目錄
boolean mkdirs() 建立包括父目錄的目錄
String getAbsolutePath() 返回此物件表示的檔案的絕對路徑名
String getName() 返回此物件表示的檔案的名稱
String getParent() 返回此File物件的路徑名的上一級,若路徑名沒有上一級,則返回null
使用mkdir建立由該File物件表示的目錄
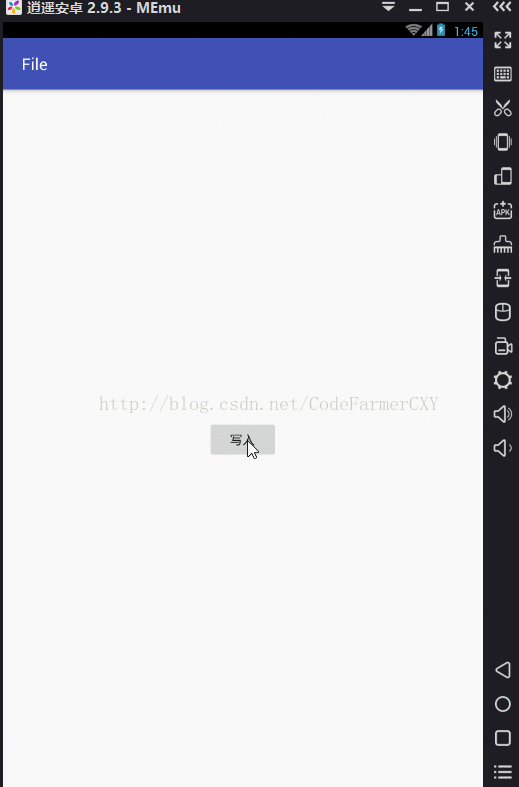
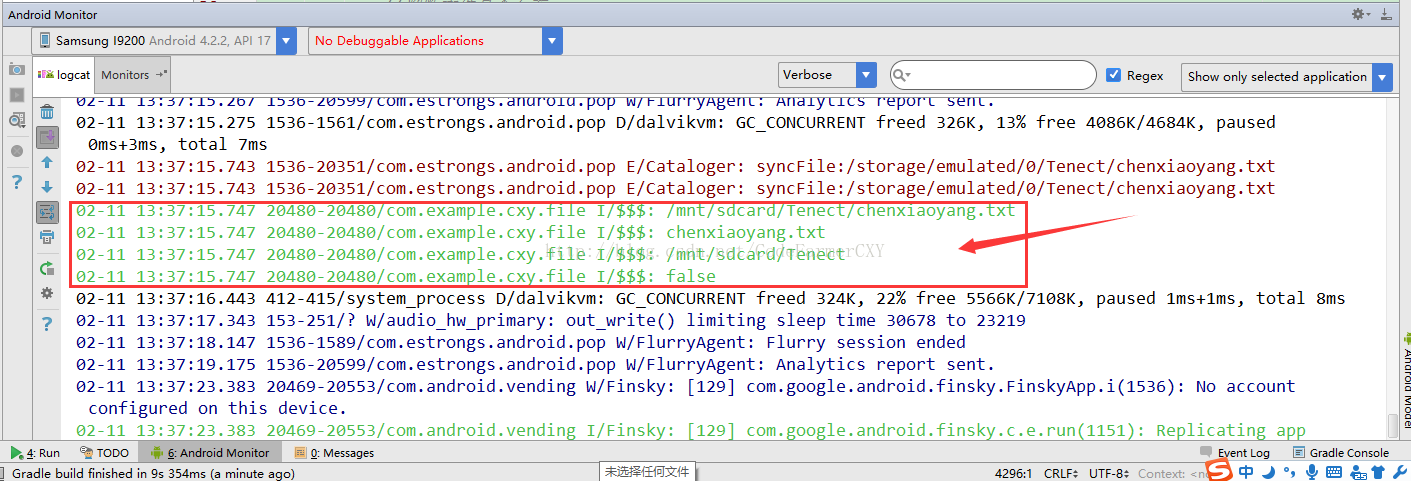
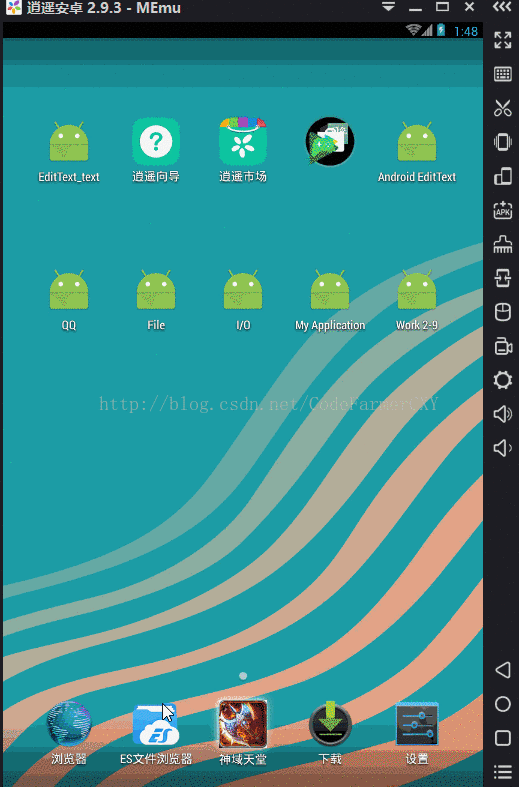
使用mkdirs建立包括父目錄的目錄
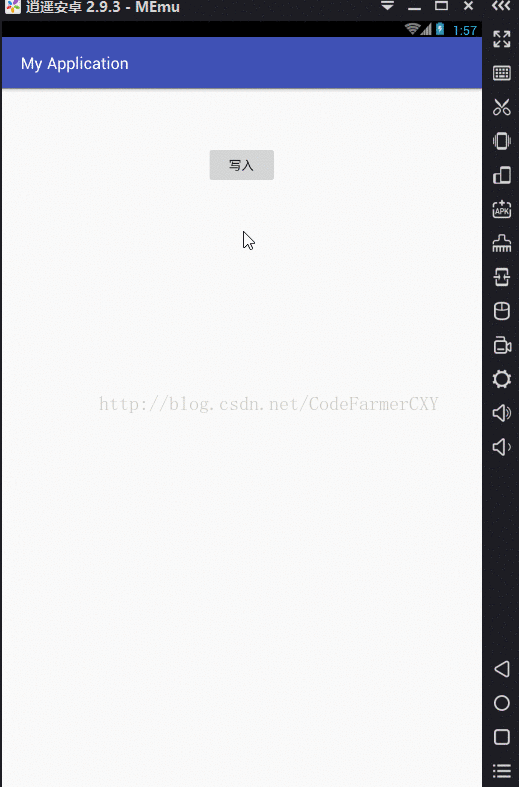
一.Android file類 在開發Android應用時免不了會跟檔案打交道,本篇文章記錄總結自己常用到的檔案操作,資料的儲存有多種方式,比如資料庫儲存、SharedPreferences儲存、檔案儲存等;這裡我們將要介紹最簡單的檔案儲存方式;檔案儲存簡單的來說就是一般的JAVASE中的IO流,只是把他應用於Android手機中而已。
二.Android file類使用
File檔案的儲存需要在程式中使用sdcard進行資料的儲存,需要在AndroidMainfset.xml檔案中進行許可權的配置: 1.SDCard中建立與刪除檔案許可權:2.SDCard寫入資料許可權 <uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE"/>
程式碼如下:
<?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android"package="com.example.cxy.file"> <uses-permission android:name="android.permission.MOUNT_FORMAT_FILESYSTEMS"></uses-permission> <uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE"></uses-permission> <application android:allowBackup="true"android:icon="@mipmap/ic_launcher" android:label="@string/app_name" android:supportsRtl="true" android:theme="@style/AppTheme"> <activity android:name=".MainActivity"> <intent-filter> <action android:name="android.intent.action.MAIN"/> <category android:name="android.intent.category.LAUNCHER"/> </intent-filter> </activity> </application> </manifest>
File類語法 1.File(String pathname) File file = new File ("/mnt/sdcard/test.txt");
2.File(String dir, String subpath) File file = new File("/mnt/sdcard/temp", "test.txt");
File類常用方法
boolean exists() 測試檔案是否存在
boolean delete() 刪除此物件指定的檔案
boolean createNewFile() 建立新的空檔案
boolean isDirectory() 測試此File物件表示的檔案是否是目錄
boolean mkdir() 建立由該File物件表示的目錄
boolean mkdirs() 建立包括父目錄的目錄
String getAbsolutePath() 返回此物件表示的檔案的絕對路徑名
String getName() 返回此物件表示的檔案的名稱
String getParent() 返回此File物件的路徑名的上一級,若路徑名沒有上一級,則返回null
使用mkdir建立由該File物件表示的目錄
package com.example.cxy.file; import android.os.Bundle; import android.support.v7.app.AppCompatActivity; import android.util.Log; import android.view.View; import android.widget.Button; import android.widget.Toast; import java.io.File; import java.io.IOException; public class MainActivity extends AppCompatActivity implements View.OnClickListener{ private Button btn; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); initView(); } private void initView(){ btn= (Button) findViewById(R.id.button); btn.setOnClickListener(this); } @Override public void onClick(View v) { //先例項化一個file物件,引數為路徑名 File file = new File("/mnt/sdcard/Tenect/chenxiaoyang.txt"); //File file = new File("/mnt/sdcard/Tenect","chenxiaoyang.txt"); try { //判斷檔案是否存在 if (file.exists()){ //檔案如果存在刪除這個檔案 file.delete(); Toast.makeText(MainActivity.this, "刪除成功了", Toast.LENGTH_SHORT).show(); }else{ //建立一個新的檔案 file=new File("/mnt/sdcard/Tenect"); //先建立資料夾 file.mkdir(); //建立這個檔案 file = new File("/mnt/sdcard/Tenect/chenxiaoyang.txt"); file.createNewFile(); Toast.makeText(MainActivity.this, "建立成功了", Toast.LENGTH_SHORT).show(); } //獲取當前file檔案的絕對路徑 Log.i("$$$",file.getAbsolutePath()); //獲取當前file檔案的名字,包括字尾名 Log.i("$$$",file.getName()); //獲取當前file檔案的以上所有級的目錄 Log.i("$$$",file.getParent()); //測試此file檔案是否是一個目錄 boolean directory=file.isDirectory(); Log.i("$$$",String.valueOf(directory)); } catch (IOException e) { e.printStackTrace(); Toast.makeText(MainActivity.this, "失敗了", Toast.LENGTH_SHORT).show(); } } }
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:paddingBottom="@dimen/activity_vertical_margin" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:paddingTop="@dimen/activity_vertical_margin" tools:context="com.example.cxy.file.MainActivity"> <Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="寫入" android:id="@+id/button" android:layout_centerVertical="true" android:layout_centerHorizontal="true"/> </RelativeLayout>
使用mkdirs建立包括父目錄的目錄
package com.example.cxy.myapplication; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.view.View; import android.widget.Button; import android.widget.Toast; import java.io.File; import java.io.IOException; public class MainActivity extends AppCompatActivity implements View.OnClickListener{ private Button btn; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); inintView(); } private void inintView() { btn= (Button) findViewById(R.id.button); btn.setOnClickListener(this); } @Override public void onClick(View v) { //先例項化一個file物件,引數為路徑名 File file=new File("/mnt/sdcard/tmp/one/two/three","test.txt"); try { //判斷檔案是否存在 if(file.exists()){ //檔案如果存在刪除這個檔案 file.delete(); Toast.makeText(MainActivity.this,"刪除成功", Toast.LENGTH_SHORT).show(); }else{ //建立一個新的檔案 file=new File("/mnt/sdcard/tmp/one/tow/three"); //先建立資料夾,mkdirds可直接建立多級資料夾 file.mkdirs(); //建立這個檔案 file=new File("/mnt/sdcard/tmp/one/tow/three/test.txt"); file.createNewFile(); } } catch (IOException e) { e.printStackTrace(); } } }
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:paddingBottom="@dimen/activity_vertical_margin" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:paddingTop="@dimen/activity_vertical_margin" tools:context="com.example.cxy.myapplication.MainActivity"> <Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="寫入" android:id="@+id/button" android:layout_alignParentTop="true" android:layout_centerHorizontal="true" android:layout_marginTop="56dp"/> </RelativeLayout>