3種 Springboot 全域性時間格式化方式,別再寫重複程式碼了
阿新 • • 發佈:2020-09-02
>本文收錄在個人部落格:[www.chengxy-nds.top](http://www.chengxy-nds.top),技術資料共享,同進步
時間格式化在專案中使用頻率是非常高的,當我們的 `API` 介面返回結果,需要對其中某一個 `date` 欄位屬性進行特殊的格式化處理,通常會用到 `SimpleDateFormat` 工具處理。
```javascript
SimpleDateFormat dateFormat = new SimpleDateFormat("yyyy-MM-dd");
Date stationTime = dateFormat.parse(dateFormat.format(PayEndTime()));
```
可一旦處理的地方較多,不僅 `CV` 操作頻繁,還產生很多重複臃腫的程式碼,而此時如果能將時間格式統一配置,就可以省下更多時間專注於業務開發了。
可能很多人覺得統一格式化時間很簡單啊,像下邊這樣配置一下就行了,但事實上這種方式只對 `date` 型別生效。
```javascript
spring.jackson.date-format=yyyy-MM-dd HH:mm:ss
spring.jackson.time-zone=GMT+8
```
而很多專案中用到的時間和日期`API` 比較混亂, `java.util.Date` 、 `java.util.Calendar` 和 `java.time LocalDateTime` 都存在,所以全域性時間格式化必須要同時相容性新舊 `API`。
---
看看配置全域性時間格式化前,介面返回時間欄位的格式。
```javascript
@Data
public class OrderDTO {
private LocalDateTime createTime;
private Date updateTime;
}
```
很明顯不符合頁面上的顯示要求(**有人抬槓為啥不讓前端解析時間,我只能說睡服程式碼比說服人容易得多~**)
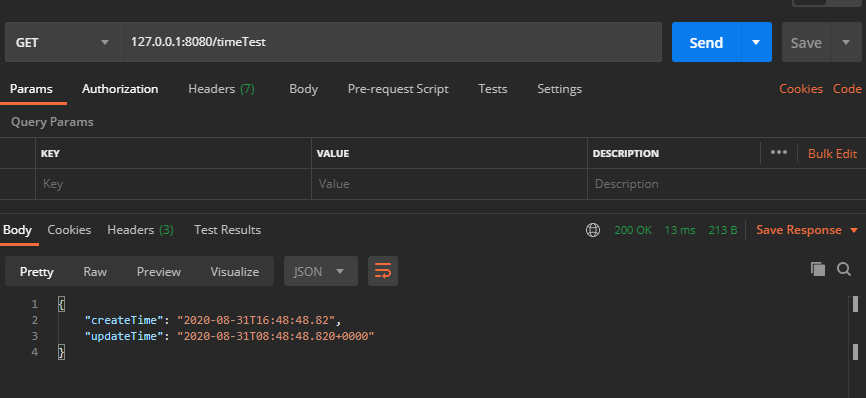
## 一、@JsonFormat 註解
`@JsonFormat` 註解方式嚴格意義上不能叫全域性時間格式化,應該叫部分格式化,因為`@JsonFormat` 註解需要用在實體類的時間欄位上,而只有使用相應的實體類,對應的欄位才能進行格式化。
```javascript
@Data
public class OrderDTO {
@JsonFormat(locale = "zh", timezone = "GMT+8", pattern = "yyyy-MM-dd")
private LocalDateTime createTime;
@JsonFormat(locale = "zh", timezone = "GMT+8", pattern = "yyyy-MM-dd HH:mm:ss")
private Date updateTime;
}
```
欄位加上 `@JsonFormat` 註解後,`LocalDateTime` 和 `Date` 時間格式化成功。
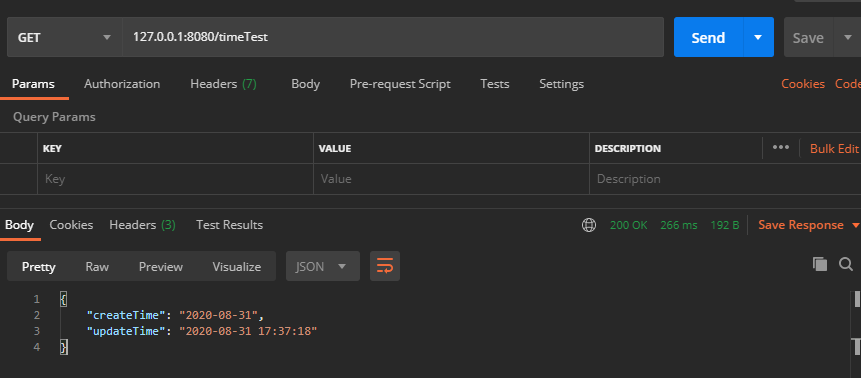
## 二、@JsonComponent 註解(**推薦**)
這是我個人比較推薦的一種方式,前邊看到使用 `@JsonFormat` 註解並不能完全做到全域性時間格式化,所以接下來我們使用 `@JsonComponent` 註解自定義一個全域性格式化類,分別對 `Date` 和 `LocalDate` 型別做格式化處理。
```javascript
@JsonComponent
public class DateFormatConfig {
@Value("${spring.jackson.date-format:yyyy-MM-dd HH:mm:ss}")
private String pattern;
/**
* @author xiaofu
* @description date 型別全域性時間格式化
* @date 2020/8/31 18:22
*/
@Bean
public Jackson2ObjectMapperBuilderCustomizer jackson2ObjectMapperBuilder() {
return builder -> {
TimeZone tz = TimeZone.getTimeZone("UTC");
DateFormat df = new SimpleDateFormat(pattern);
df.setTimeZone(tz);
builder.failOnEmptyBeans(false)
.failOnUnknownProperties(false)
.featuresToDisable(SerializationFeature.WRITE_DATES_AS_TIMESTAMPS)
.dateFormat(df);
};
}
/**
* @author xiaofu
* @description LocalDate 型別全域性時間格式化
* @date 2020/8/31 18:22
*/
@Bean
public LocalDateTimeSerializer localDateTimeDeserializer() {
return new LocalDateTimeSerializer(DateTimeFormatter.ofPattern(pattern));
}
@Bean
public Jackson2ObjectMapperBuilderCustomizer jackson2ObjectMapperBuilderCustomizer() {
return builder -> builder.serializerByType(LocalDateTime.class, localDateTimeDeserializer());
}
}
```
看到 `Date` 和 `LocalDate` 兩種時間型別格式化成功,此種方式有效。
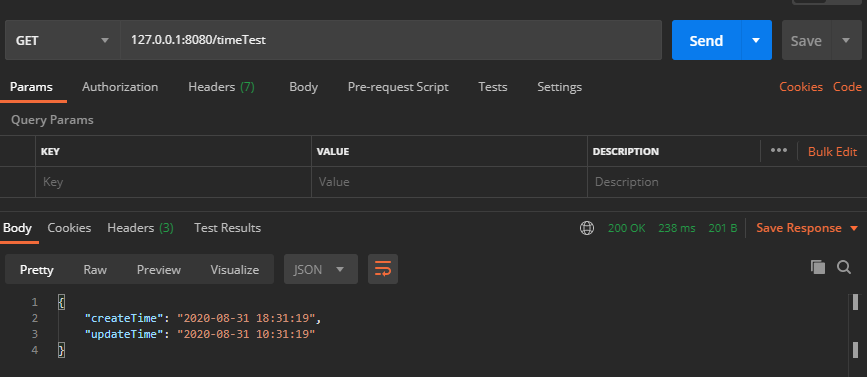
但還有個問題,實際開發中如果我有個欄位不想用全域性格式化設定的時間樣式,**想自定義格式怎麼辦?**
那就需要和 `@JsonFormat` 註解配合使用了。
```javascript
@Data
public class OrderDTO {
@JsonFormat(locale = "zh", timezone = "GMT+8", pattern = "yyyy-MM-dd")
private LocalDateTime createTime;
@JsonFormat(locale = "zh", timezone = "GMT+8", pattern = "yyyy-MM-dd")
private Date updateTime;
}
```
從結果上我們看到 `@JsonFormat` 註解的優先順序比較高,會以 `@JsonFormat` 註解的時間格式為主。
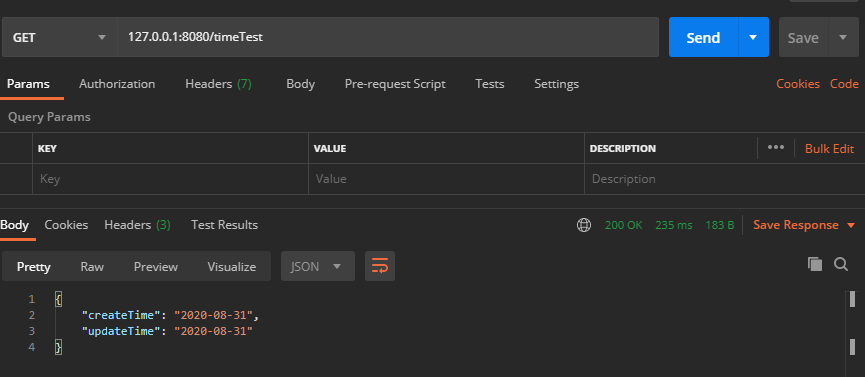
## 三、@Configuration 註解
這種全域性配置的實現方式與上邊的效果是一樣的。
>**注意**:在使用此種配置後,欄位手動配置`@JsonFormat` 註解將不再生效。
```javascript
@Configuration
public class DateFormatConfig2 {
@Value("${spring.jackson.date-format:yyyy-MM-dd HH:mm:ss}")
private String pattern;
public static DateFormat dateFormat = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
@Bean
@Primary
public ObjectMapper serializingObjectMapper() {
ObjectMapper objectMapper = new ObjectMapper();
JavaTimeModule javaTimeModule = new JavaTimeModule();
javaTimeModule.addSerializer(LocalDateTime.class, new LocalDateTimeSerializer());
javaTimeModule.addDeserializer(LocalDateTime.class, new LocalDateTimeDeserializer());
objectMapper.registerModule(javaTimeModule);
return objectMapper;
}
/**
* @author xiaofu
* @description Date 時間型別裝換
* @date 2020/9/1 17:25
*/
@Component
public class DateSerializer extends JsonSerializer {
@Override
public void serialize(Date date, JsonGenerator gen, SerializerProvider provider) throws IOException {
String formattedDate = dateFormat.format(date);
gen.writeString(formattedDate);
}
}
/**
* @author xiaofu
* @description Date 時間型別裝換
* @date 2020/9/1 17:25
*/
@Component
public class DateDeserializer extends JsonDeserializer {
@Override
public Date deserialize(JsonParser jsonParser, DeserializationContext deserializationContext) throws IOException {
try {
return dateFormat.parse(jsonParser.getValueAsString());
} catch (ParseException e) {
throw new RuntimeException("Could not parse date", e);
}
}
}
/**
* @author xiaofu
* @description LocalDate 時間型別裝換
* @date 2020/9/1 17:25
*/
public class LocalDateTimeSerializer extends JsonSerializer {
@Override
public void serialize(LocalDateTime value, JsonGenerator gen, SerializerProvider serializers) throws IOException {
gen.writeString(value.format(DateTimeFormatter.ofPattern(pattern)));
}
}
/**
* @author xiaofu
* @description LocalDate 時間型別裝換
* @date 2020/9/1 17:25
*/
public class LocalDateTimeDeserializer extends JsonDeserializer {
@Override
public LocalDateTime deserialize(JsonParser p, DeserializationContext deserializationContext) throws IOException {
return LocalDateTime.parse(p.getValueAsString(), DateTimeFormatter.ofPattern(pattern));
}
}
}
```
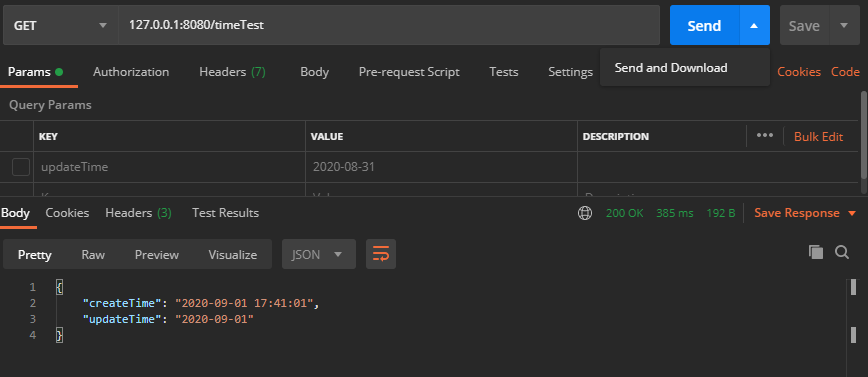
## 總結
分享了一個簡單卻又很實用的 `Springboot` 開發技巧,其實所謂的開發效率,不過是一個又一個開發技巧堆砌而來,聰明的程式設計師總是能用最少的程式碼完成任務。
如果對你有用,歡迎 `在看`、`點贊`、`轉發` ,您的認可是我最大的動力。
**原創不易,燃燒秀髮輸出內容,如果有一丟丟收穫,點個贊鼓勵一下吧!**
整理了幾百本各類技術電子書,送給小夥伴們。關注公號回覆【**666**】自行領取。和一些小夥伴們建了一個技術交流群,一起探討技術、分享技術資料,旨在共同學習進步,如果感興趣就加入我們吧!
![在這裡插入圖片描述](https://img-blog.csdnimg.cn/20200828110008952.png?x-oss-process=image/watermark,type_ZmFuZ3poZW5naGVpdGk,shadow_10,text_aHR0cHM6Ly9ibG9nLmNzZG4ubmV0L3hpbnpoaWZ1MQ==,size_16,color_FFFFFF,t_70#pic_