三、資料結構演算法-棧、佇列、優先佇列、雙端佇列
阿新 • • 發佈:2020-12-30
## 一、Stack (棧)
### 1、資料結構
Stack是棧。它的特性是:**先進後出(FILO, First In Last Out) 後進先出(Last in - First out)**。java工具包中的Stack是繼承於Vector(向量佇列)的,由於Vector是通過陣列實現的,這就意味著,Stack也是通過陣列實現的,而非連結串列。當然,我們也可以將LinkedList當作棧來使用。
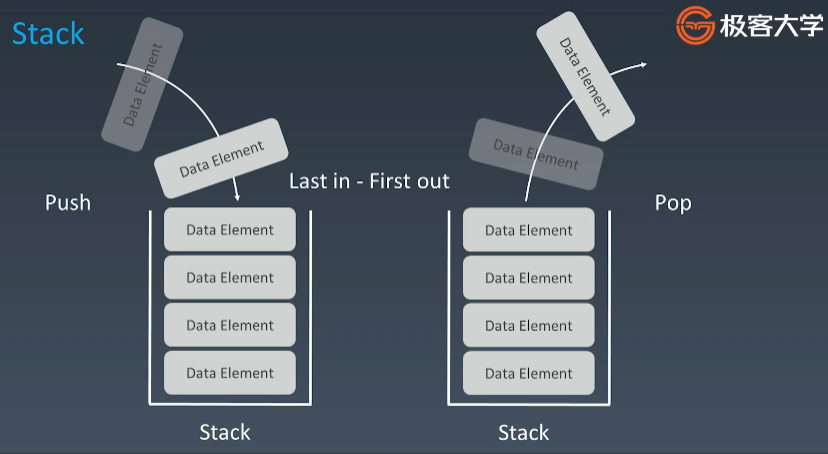
### 2、Stack的繼承關係
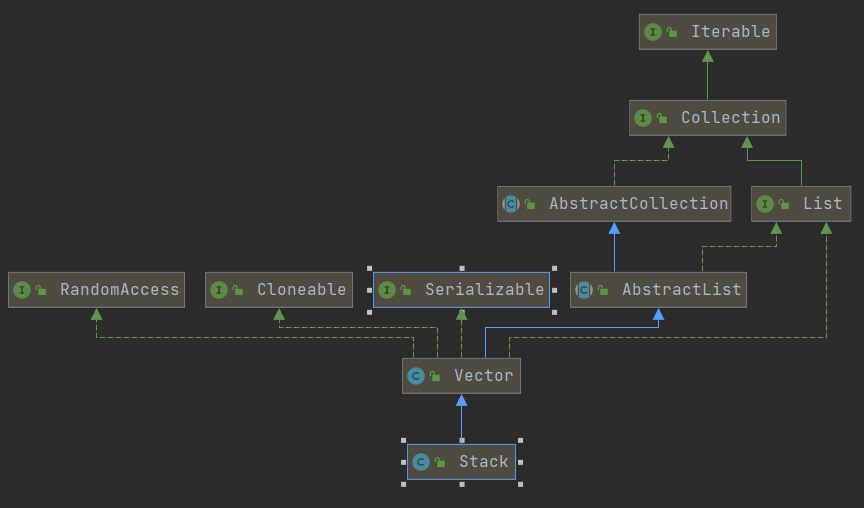
### 3、時間複雜度
| 操作 | 時間複雜度 |
| ---- | ---- |
| lookup | O(n) |
| insert | O(1) |
| delete | O(1) |
### 4、原始碼實現
`Stack` 繼承自 `Vector` 底層為動態陣列實現
```
/**
* 向棧頂新增元素
* Pushes an item onto the top of this stack. This has exactly
* the same effect as:
* object).
* @throws EmptyStackException if this stack is empty.
*/
public synchronized E pop() {
E obj;
int len = size();
obj = peek();
removeElementAt(len - 1); // Vector 中的按照下標移除元素的方法
return obj;
}
/**
* Looks at the object at the top of this stack without removing it
* from the stack.
* 檢視棧頂元素但不刪除
* @return the object at the top of this stack (the last item
* of the Vector object).
* @throws EmptyStackException if this stack is empty.
*/
public synchronized E peek() {
int len = size();
if (len == 0)
throw new EmptyStackException();
return elementAt(len - 1);
}
// 棧是否為空
public boolean empty() {
return size() == 0;
}
// 查詢“元素o”在棧中的位置:由棧底向棧頂方向數
public synchronized int search(Object o) {
// 獲取元素索引,elementAt()具體實現在Vector.java中
int i = lastIndexOf(o);
if (i >= 0) {
return size() - i;
}
return -1;
}
```
[Java 的 Stack 原始碼](http://developer.classpath.org/doc/java/util/Stack-source.html)
## 二、Queue (佇列)
### 1、資料結構
**First in - First out(先進先出 FIFO)**
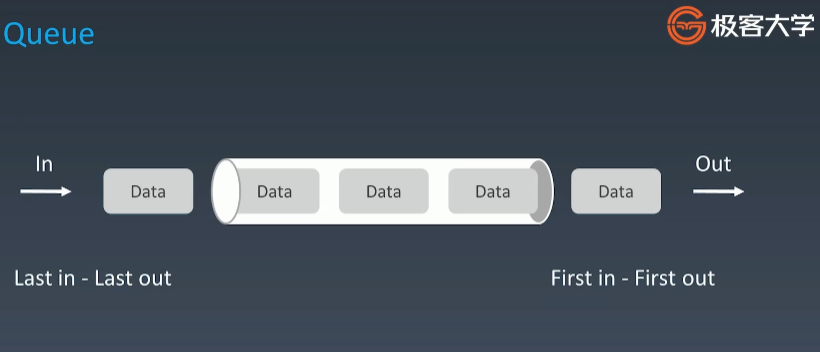
### 2、時間複雜度
| 操作 | 時間複雜度 |
| ---- | ---- |
| lookup | O(n) |
| insert | O(1) |
| delete | O(1) |
### 3、原始碼實現
```
//介面Queue:
public interfa
* * @param item the item to be pushed onto this stack. * @return the* addElement(item)
item
argument.
* @see java.util.Vector#addElement
*/
public E push(E item) {
addElement(item); // Vector 中的新增方法
return item;
}
/**
* Removes the object at the top of this stack and returns that
* object as the value of this function.
* 檢視棧頂元素並刪除
* @return The object at the top of this stack (the last item
* of the Vector